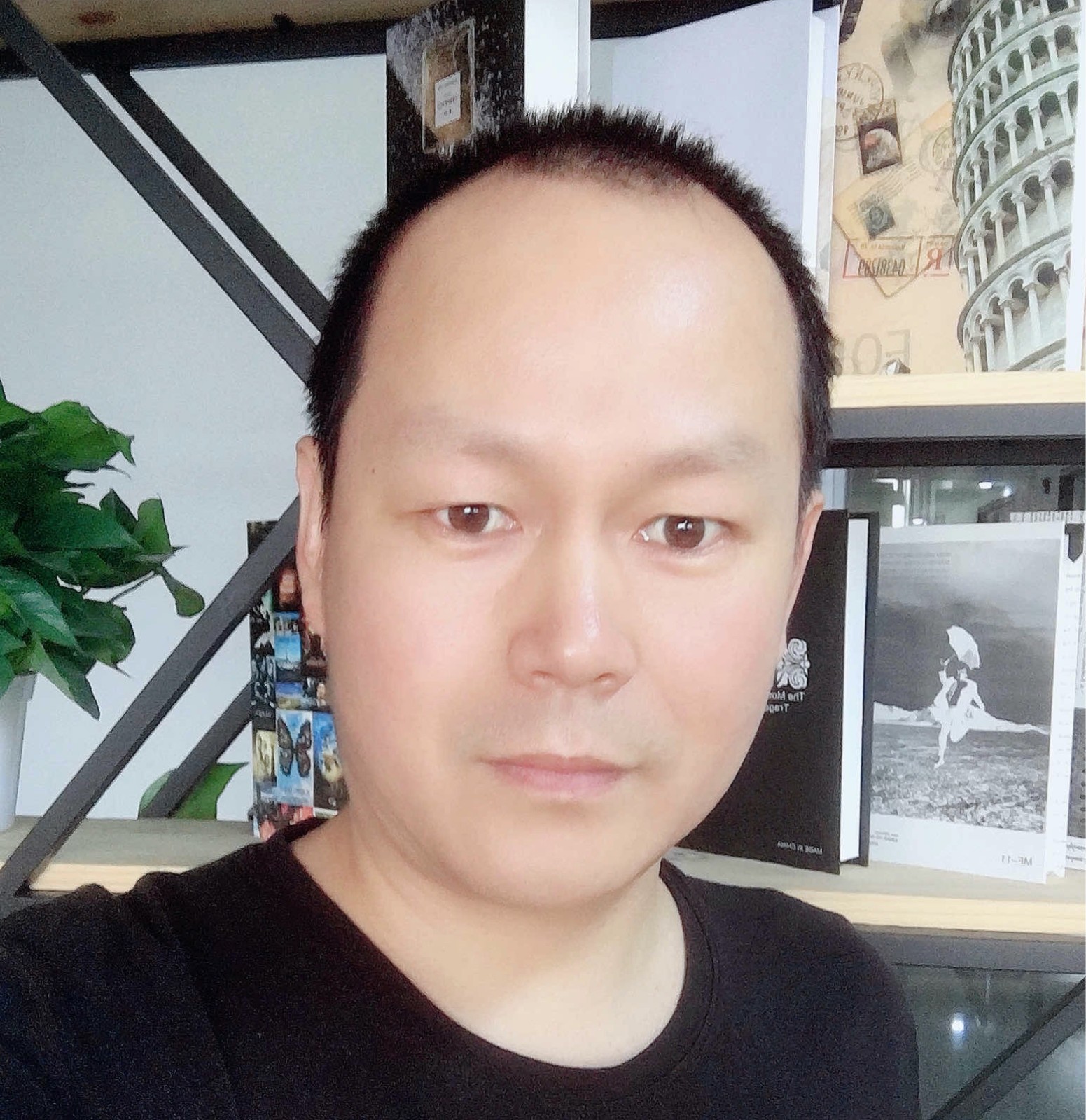
Correction status:qualified
Teacher's comments:棒棒的
<?php
//1.传统方式:include / require
//加载类文件
require 'app/controller/User.php';
//注册类别名
use app\controller\User;
//调用类成员
echo User::hello();
<?php
//2.注册自动加载函数
//加载类文件使用一个内置的函数来完成
spl_autoload_register(function($class){
//获取类的路径名称
$path = str_replace('\\',DIRECTORY_SEPARATOR,$class);
//生成一个完整的类文件名
$classFile = __DIR__ . '/' . $path . '.php';
//加载类文件
require $classFile;
});
use app\controller\User;
echo User::hello();
<?php
//3.使用composer中的自动加载
//调用的是composer中的自动加载功能
require_once __DIR__ . '/vendor/autoload.php';
use app\controller\User;
echo User::hello();
{
"autoload": {
"psr-4": {
"app\\controller\\": "app/controller"
}
},
"require": {
"gregwar/captcha": "^1.1"
}
}
<?php
//如何在项目中使用第三方依赖库/组件
//composer自动加载器
require_once __DIR__ . '/vendor/autoload.php';
//注册类(验证码)
use Gregwar\Captcha\CaptchaBuilder;
$captcha = new CaptchaBuilder;
$captcha
->build()
->save('out.jpg');
//获取验证码
$_SESSION['phrase'] = $captcha->getPhrase();
echo $_SESSION['phrase'];
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<table>
<caption>用户登录</caption>
<tr>
<td>邮箱:</td>
<td><input type="email"></td>
</tr>
<tr>
<td>密码:</td>
<td><input type="password"></td>
</tr>
<tr>
<td>验证码:</td>
<td><img src="<?php echo $captcha->inline();?>" onclick="location.reload()" alt=""></td>
</tr>
<tr>
<td colspan="2"><button>登录</button></td>
</tr>
</table>
</body>
</html>
模型用medoo包,用终端下载:输入composer require catfan/medoo
命令
视图用plates包,输入composer require league/plates
命令
<?php
//模型类
namespace core;
use Medoo\Medoo;
//继承自第三方包:catfan/medoo
class Model extends Medoo
{
//构造方法:连接数据库
public function __construct()
{
$options = [
'database_type'=>'mysql',
'database_name'=>'tp5',
'server'=>'localhost',
'username'=>'root',
'password'=>'wang1111'
];
parent::__construct($options);
}
}
<?php
//视图类
namespace core;
use League\Plates\Engine;
class View extends Engine
{
public $templates;
public function __construct($path)
{
$this->templates = parent::__construct($path);
}
}
<?php
namespace models;
use core\Model;
//用户自定义模型通常与一张数据表对应
class UsersModel extends Model
{
public function __construct()
{
parent::__construct();
}
}
<?php
namespace controllers;
class UsersController
{
//将依赖的模型对象和视图对象,使用依赖注入到构造方法中,使它在控制器中共享
public $model = null;
public $view = null;
//构造方法
public function __construct($model,$view)
{
$this->model = $model;
$this->view = $view;
}
public function index()
{
return __METHOD__;
}
public function select()
{
$users = $this->model->select('user',['id','name','username'],['LIMIT'=>5]);
//将数据渲染到模板上(模板赋值同步完成)
return $this->view->render('users/list',['users'=>$users]);
}
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>用户信息</title>
</head>
<body>
<table border="1" cellpadding="10px" cellspacing="0">
<caption>用户管理</caption>
<tr>
<th>id</th>
<th>姓名</th>
<th>账号</th>
</tr>
<?php foreach($users as $user): ?>
<tr>
<td><?=$this->e($user['id'])?></td>
<td><?=$this->e($user['name'])?></td>
<td><?=$this->e($user['username'])?></td>
</tr>
<?php endforeach; ?>
</table>
</body>
</html>
{
"require": {
"catfan/medoo": "^1.7",
"league/plates": "^3.3"
},
"autoload": {
"psr-4": {
"models\\": "app/models",
"core\\": "core",
"views\\": "app/views",
"controllers\\": "app/controllers"
}
}
}
composer dumpautoload
更新类的映射关系
<?php
use models\UsersModel;
use core\View;
use controllers\UsersController;
//composer加载器
require_once __DIR__ . '/vendor/autoload.php';
//测试模型
$model = new UsersModel();
//var_dump($model);
//测试视图
$view = new View('app/views');
//var_dump($view);
//测试控制器
$controller = new UsersController($model,$view);
//var_dump($controller);
print_r($controller->select());