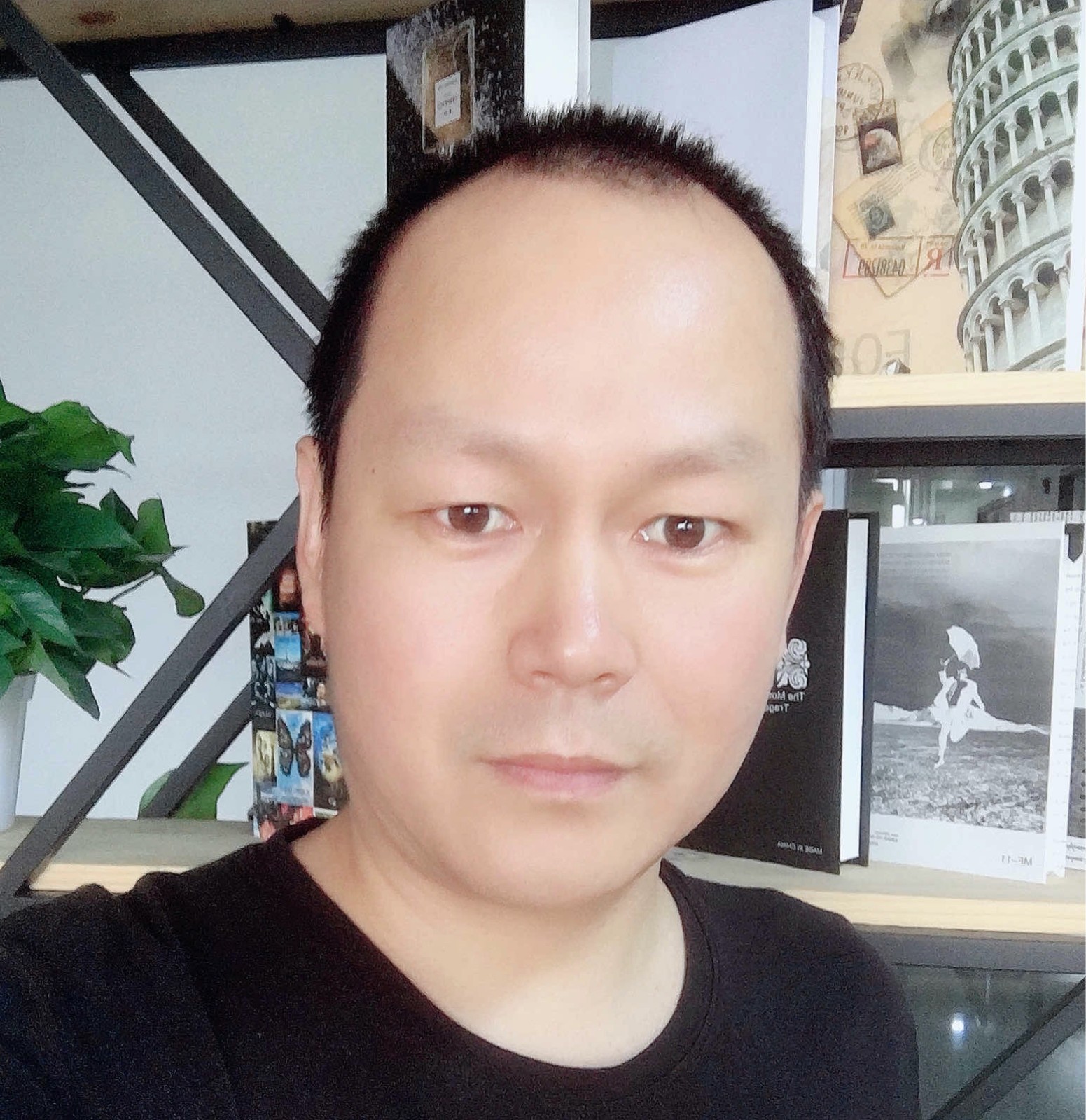
Correction status:qualified
Teacher's comments:json 是目前互联网数据的基础,很重要
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>数据类型与访问方式</title>
</head>
<body>
<script>
// 1.原始类型
var grade = 20;
var username = "melinda";
var flag = false;
// 类型检查用 typeof,会得到:number数值,string字符串,bool布尔
console.log(typeof grade, typeof username, typeof flag);
// 2.特殊类型
// null是空对象,得到object
var title = null;
console.log(typeof title);
// undefined未定义
var role = undefined;
console.log(typeof role);
console.log(null === undefined);
// 4.值相等,但类型不同,所以 === 返回false
// 5. 返回数组,对象: object, array, function
var fruits = ["watermelon", "apple", "orange", "peach", "pear"];
console.log(fruits);
var student = {
id: 1,
name: "melinda",
email: "melinda@php.cn",
course: [90, 88, 93],
"my scroe": {
php: 90,
js: 60,
css: 70,
},
};
var keys = Object.keys(student);
// 6.命名函数
function f1(a, b) {
console.log(a + b);
}
f1(1, 2);
// 7.匿名函数
var f2 = function (a, b) {
console.log(a + b);
};
f2(100, 20);
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>流程控制</title>
</head>
<body>
<ul>
<li>item1</li>
<li>item2</li>
<li>item3</li>
</ul>
<ul>
<li>item1</li>
<li>item2</li>
<li>item3</li>
</ul>
<script>
// 1. 分支:单分支
var age = 59;
age = 30;
// 如果代码块中只有一条语句,可以省略"{...}"
if (age >= 50) {
console.log("退休");
}
// 双分支
if (age >= 50) {
console.log("回农村");
} else {
console.log("奋斗吧");
}
// 多分支
age = 25;
if (age > 18 && age < 30) {
console.log("努力赚钱");
} else if (age >= 30 && age < 50) {
console.log("有家有孩子");
} else if (age >= 50) {
console.log("老有所依");
}
// 默认分支 else
else {
console.log("我才", age, ", 努力工作");
}
// switch简化
switch (true) {
case age > 18 && age < 30:
console.log("奋斗");
break;
case age >= 30 && age < 50:
console.log("有房有车");
break;
case age >= 50:
console.log("白头到老");
break;
default:
console.log("我才", age, ", 努力工作");
}
// 循环
var lis = document.querySelectorAll("ul:first-of-type li");
// for
for (var i = 0; i < lis.length; i++) {
lis[i].style.backgroundColor = "lightgreen";
}
// while
var lis = document.querySelectorAll("ul:last-of-type li");
var i = 0;
while (i < lis.length) {
lis[i].style.backgroundColor = "yellow";
i++;
}
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>js对象序列化,json格式的字符串</title>
</head>
<body>
<script>
// ## 1. JSON 是什么
// - JSON: JavaScript Object Notation(JS 对象表示法)
// - JSON: 2006 年成为国际标准,并成为表示结构化数据的通用的格式
// - JSON 借用了**JS 对象字面量**的语法规则,但并非 JS 对象,也并非只是 JS 才用 JSON
// - JSON 独立于任何编程语言, 几乎所有编程语言都提供了访问 JSON 数据的 API 接口
// - 尽管 JSON 与 JS 并无直接关系,但 JSON 在 JS 代码中应用最广泛
// ## 2. JSON 语法
// JOSN 支持三种数据类型: 简单值, 对象, 数组
// ### 2.1 简单值
// - 数值: `150`, `3.24`
// - 字符串: `"Hello World!"`,必须使用**双引号**做为定界符
// - 布尔值: `true`, `false`
// - 空值: `null`
// > 注意: 不支持`undefined`
var person = {
name: "melinda",
age: 25,
isMarried: true,
course: {
name: "JavaScript",
grade: 99,
},
getName: function () {
return this.name;
},
hobby: undefined,
toString: function () {
return "继承属性";
},
};
// 将js对象转为json格式的字符串
var jsonStr = JSON.stringify(person);
// 传入第二参数数组,对输出的结果进行限制
jsonStr = JSON.stringify(person, ["name", "age"]);
// 如果第二个参数是一个回调,可以进行动态过滤,传键和值
jsonStr = JSON.stringify(person, function (key, value) {
switch (key) {
case "age":
return "保密";
case "isMarried":
return undefined;
// 必须要有default, 才可以输出其它未处理的属性
default:
return value;
}
});
// json中没有方法, undefined也没有
console.log(jsonStr);
</script>
</body>
</html>
JOSN
支持三种数据类型: 简单值, 对象, 数组10
, 10.1
"Hello World!"
,必须使用双引号做为定界符true
, false
null
undefined