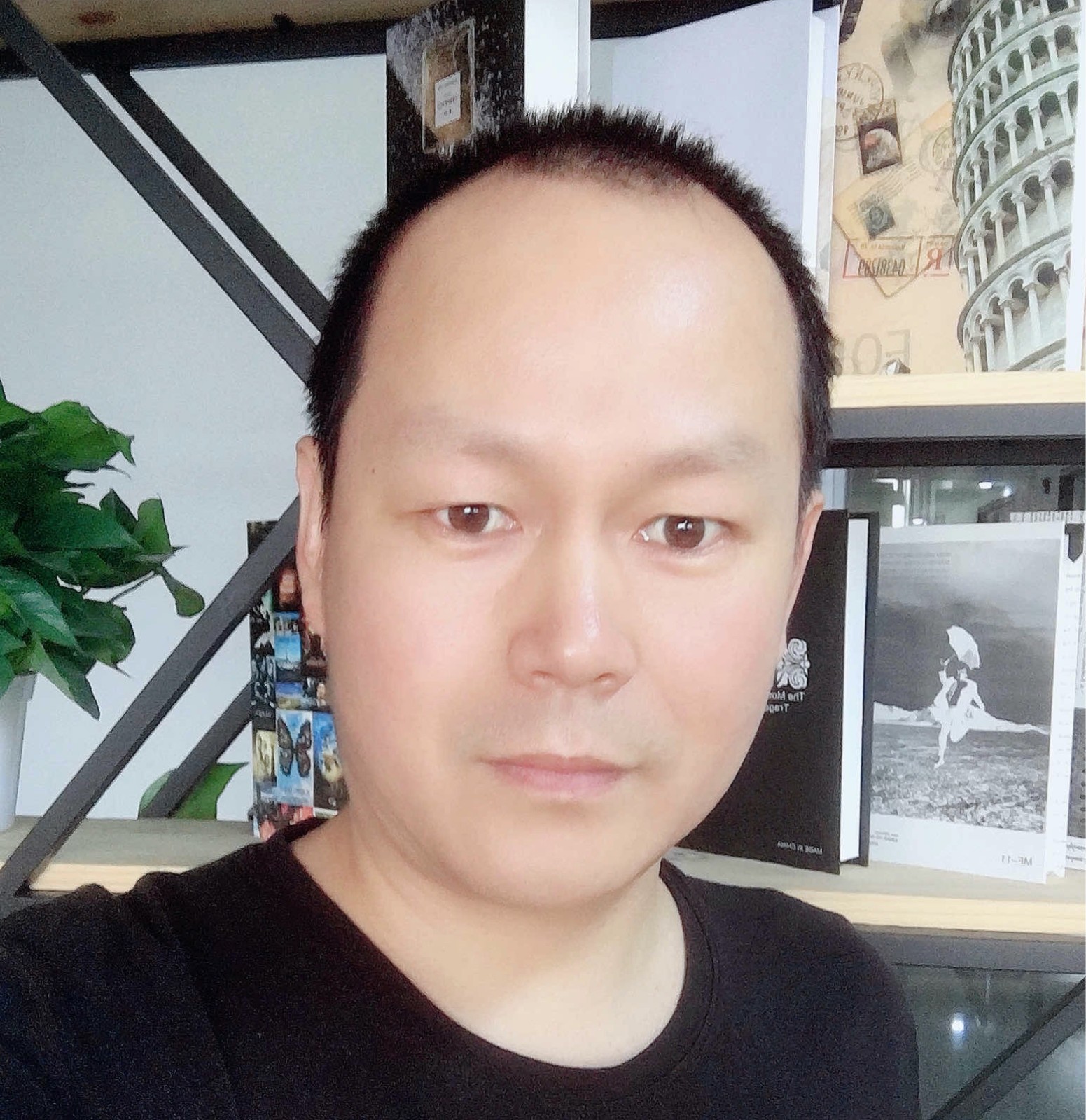
Correction status:qualified
Teacher's comments:其实不必完全照抄课堂代码的, 你可以有自己的想法
function addNum($num1,$num2)
{
retrun $num1 + $num2;
}
echo addNum(1,2);
由系统事先声明好,用户只须调用即可
将函数的名称放在一个变量中进行引用
//与演示中的自定义函数相关联
$funcName = 'addNum';
echo '和值:',$funcName(9000, 0.6);
//命名函数: 有名称
// 匿名函数: 没有名称
$getPrice = function (float $money, float $discount) : float {
return $money * $discount;
};
// 匿名函数在php中也称之为闭包,闭包也是一个函数,可以访问上一级空间的成员
// 将折扣率变量声明到它的全局空间,是它的父作用域
$discount = 0.8;
$p = function (float $money) use ($discount) : float {
return $money * $discount;
};
echo '实付金额: ' , $p(10000), ' 元<hr>';
// 闭包更多是指子函数
$f = function ($discount) {
// $discount = 0.8;
return function (float $money, int $n) use ($discount) : float {
$total = $money * $n;
return ($total > 20000) ? ($total * $discount) : $total;
};
};
// $p 是闭包函数的引用
// $p = $f(0.5);
echo '实付金额: ' , $p(10000, 5) , ' 元<hr>';
echo '实付金额: ' , $f(0.6)(10000, 5) , ' 元<hr>';
// 闭包是匿名函数最常用的应用场景之一
// 闭包是一个子函数,全局不可见,但是它的父函数可见
// 闭包全局无法调用,可以当成父函数的返回值返回到全局,它可以将函数中的一些内容带给全局使用
// 因为函数一旦调用完成,内部全部释放掉, 外部不再能访问,外部不能访问函数中的私有成员
// 那么函数中的私有成员 , 可以通过它的子函数(闭包)返回到全局供它使用
function demo1(): string
{
$status = 1;
$message = '成功';
return $status . $message ;
}
echo demo1();
function demo2(): array
{
$status = 1;
$message = '成功';
return ['status'=>$status, 'message'=>$message] ;
}
function demo3(): string
{
$status = 1;
$message = '成功';
// 将数组进行json编码,返回json格式的字符串
return json_encode(['status'=>$status, 'message'=>$message]);
}
function demo4(): string
{
$status = 1;
$message = '成功';
// 将返回值序列化成字符串返回多个值
return serialize(['status'=>$status, 'message'=>$message]);
}
$str = demo4();
echo $str;
$arr = unserialize($str);
print_r($arr);
1.值参数
// 1. 值参数: 值传递参数,这是默认的方式
function demo1(float $arg) : float
{
// 在函数中对参数的改变并不会映射到外部的参数
// return $arg = $arg * 2;
return $arg *= 2;
}
$var = 100;
echo demo1($var), '<br>';
echo $var, '<hr>';
2.引用参数
// 2. 引用参数, 在参数前添加取地址符: &
function demo2(float &$arg) : float
{
// 在函数中对参数的改变映射到外部的参数
return $arg *= 2;
}
$var = 100;
echo demo2($var), '<br>';
echo $var, '<hr>';
3.默认参数
// 3. 默认参数
function demo3(float $a, float $b, string $opt = '+') : string
{
switch ($opt) {
case '+':
return sprintf('%d + %d = %d', $a, $b, ($a + $b));
break;
case '-':
return sprintf('%d - %d = %d', $a, $b, ($a - $b));
break;
case '*':
return sprintf('%d * %d = %d', $a, $b, ($a * $b));
break;
case '/':
if ($b !== 0)
return sprintf('%d / %d = %f', $a, $b, ($a / $b));
else die('除数不能为零');
break;
default:
die('非法操作符');
}
}
echo demo3(10, 20, '+'), '<br>';
echo demo3(10, 20), '<br>';
echo demo3(10, 20, '*'), '<br>';
echo demo3(10, 20, '/'), '<br>';
4.剩余参数
// ...rest, ...spread 语法
function demo4($a, $b, $c)
{
return $a + $b + $c;
}
// 这个函数只能计算二个数的和
echo demo4(39, 22, 20), '<br>';
function demo5()
{
// print_r(func_get_args());
return array_sum(func_get_args());
}
echo demo5(12,34,55,3,8,12,90), '<br>';
// ...rest 剩余参数
// ...args: 将传给参数的所有参数全部压到一个数组中,args
function demo6(...$args)
{
// print_r($args);
return array_sum($args);
}
echo demo6(12,34,55,3,8,12,90), '<br>';
// ...rest / ...spread 混合在一起用
function demo7(...$args)
{
// print_r($args);
return array_sum($args);
}
$data = [12,34,55,3,8,12,90];
// ...spread用在调用时的参数中,用在有具体值的参数列表,实参
echo demo7(...$data), '<br>';
echo demo7(...[2,3,6,7,1,9]);
// ...name: 当用在调用参数中, 是展开功能,当用在声明参数中, 是归内功能
// 直接调用(同步调用:这条调用语句不执行完成, 后面语句不会被执行)
echo demo1(1,2,3), '<br>';
// 以回调的方式来执行一个函数
echo call_user_func('demo1', 1,2,3);
echo '<hr>';
echo call_user_func_array('demo1', [1,2,3]);
// 例如,demo1()函数执行时间非常的耗时,此时用提回调方式执行,那么后面的"echo 'hello word';:"不必等待demo1()执行完成
// echo 'hello word';
echo '<hr>';
// 回调函数是异步执行的重要工具
(PHP 4 >= 4.0.4, PHP 5, PHP 7)
call_user_func_array — 调用回调函数,并把一个数组参数作为回调函数的参数
call_user_func_array ( callable $callback , array $param_arr ) : mixed
把第一个参数作为回调函数(callback)调用,把参数数组作(param_arr)为回调函数的的参数传入。
callback
被调用的回调函数。
param_arr
要被传入回调函数的数组,这个数组得是索引数组。
返回回调函数的结果。如果出错的话就返回FALSE
namespcae
<?php
// 函数的命名空间
// __FUNCTION__: 返回当前的函数名称字符串
namespace ns1;
function demo1()
{
return __FUNCTION__;
}
echo demo1();
echo '<hr>';
// 在php中不允许在同一个命名空间中声明声明同名函数的, js可以
namespace ns2;
function demo1()
{
return __FUNCTION__;
}
echo demo1();
echo '<br>';
// 也可调用另一个空间的函数
echo \ns1\demo1();
字符串长度上限: 2G
创建方式
$str = 'string';
// 单引号中的变量不能被解析出来
echo 'This is a $str hello word. <br>';
// 通过字符串与变量接来来解析变量,将变量与字符串组合后输出
echo 'This is a '.$str.' hello word. <br>';
// 单引号的特殊字符不能被解析,原样输出
echo 'hello \n\r word. <br>';
// 单引号其实有二重意义: 1. 本义就是字符, 2. 字符串的定界符
// 单引号中又出现单引号,必须使用转义符: \
echo 'This is a \'test\'. <br>';
// 如果又出现了转义符: \, 应该连写2个才可以正常输出,否则就是转义
echo 'This is a \'test\\\'. <br>';
echo '<hr>';
// 双引号中的变量能被解析出来
echo "This is a $str hello word. <br>";
// echo "This is a $str123 hello word. <br>";
// echo "This is a $str 123 hello word. <br>";
echo "This is a {$str}123 hello word. <br>";
// ${var}: 与es6中的模板字符串语法相同
echo "This is a ${str}123 hello word. <br>";
// 双引号的特殊字符能被解析,多个空格或回车在页面视为1个
echo "hello \n\r word. <br>";
echo "This is a \"demo\". <hr>";
$hello = 'php.cn';
echo "<p>Hello <span style=\"color:red\">$hello</span></p>";
// heredoc: 可以解析变量,且双引号不需要转义,非常适合php+html写模板
echo <<< HELLO
<p>Hello <span style="color:red">$hello</span></p>
HELLO;
// heredoc的起始标识符可以添加双引号
适合大段的纯文本,不适合内嵌变量或特殊字符的文本
echo 'This is test';
// nowdoc的起始标签,必须添加单引号
echo <<< 'abc'
This is test<br>This is test<br>This is test<br>This is test<br>
abc;