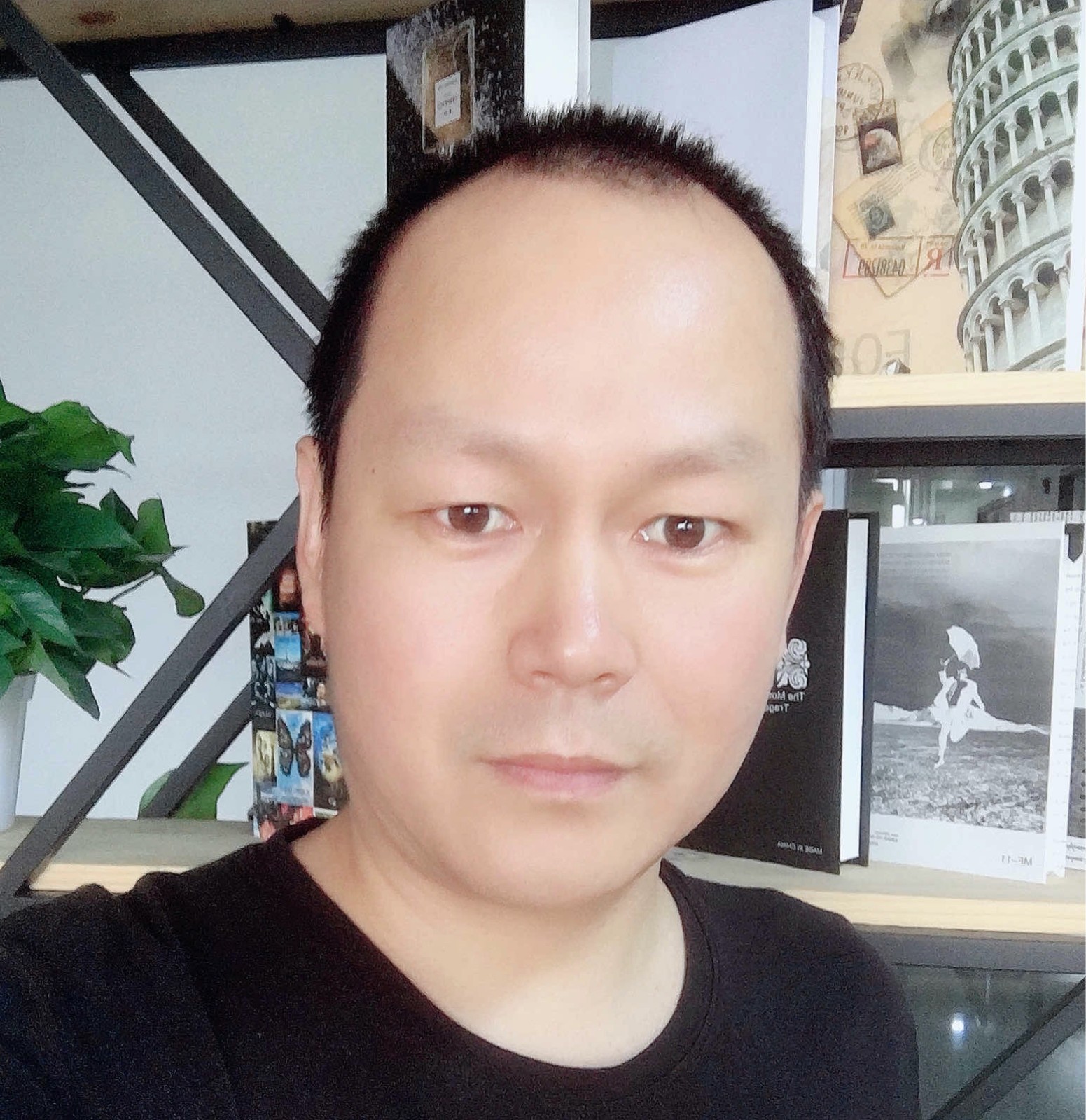
Correction status:qualified
Teacher's comments:
建议遵循PSR-2命名规范和PSR-4自动加载规范
类的命名采用驼峰法(首字母大写),例如 User、UserType;
属性的命名使用驼峰法(首字母小写),例如 tableName、instance;
方法的命名使用驼峰法(首字母小写),例如 getUserName;
//1.类的声明
class Goods{
//类成员
//1.类属性(可理解为变量)
//2.类方法(可理解为函数)
//类属性
public $a="hello";
//抽象属性(未赋值)
public $b;
//类的实例构造方法/器
public function __construct()
{
}
}
//2.类的实例化,就是创建对象的对象
//类的构造方法必须用:new来调用
$goods=new Goods(); //如果不需要参数,可以写成new Goods;不带()
var_dump($goods); //是一个对象
echo "<br>";
var_dump($goods instanceof Goods); //返回布尔值,看对象是否属于实例
//实例和对象在不引起误会情况下,可以通用
echo "<br>";
//动态类
$classname="Goods";
$books= new $classname();
echo get_class($books);//返回对象实例 obj 所属类的名字
class User{
//公共成员,在类内部和类外部都可以访问,都可见。
public $name="张三";
public $points=[100,80,90];
//静态属性,如果此属性只用一次,可以不创建类实例,直接通过类来调用
public static $nation="CHINA";
//抽象属性,没被初始化的属性
public $a; //默认值是Null
}
//实例化一个对象
$user=new User;
//类中成员 属性的访问
echo $user->name;
echo "<br>";
print_r($user->points);
echo "<br>";
echo $user->points[1];
echo "<br>";
//调用类静态属性 用::
echo User::$nation;
echo "<br>";
var_dump($user->a);
//类中的方法
class User{
public $name="张三";
Public $age="20";
public static $nation="中国";
public function write1(){
$user=new User;
return "名字是:{$user->name},年龄是{$user->age}";
}
public function write2(){
$gongzi="8000";
//self类引用 this类对象引用
//$user=new self;
//$this=new self; 会自动创建。 不能再重新创建。
return "名字是:{$this->name},年龄是{$this->age},工资是{$gongzi}";
}
//类方法中访问类外部成员:变量和函数
public function write3(){
$str=hello();
global $username;//声明一个外部变量。 不推荐这样用。应该用依赖注入
return $str.$username;
//或者用 return $str.$GLOBAL['username'];
}
//实际项目中,外部项目应该通过方法参数注入到方法中,而不是在方法中直接调用
//依赖注入
public function write4(Closure $hello2,string $username){
return $hello2().$username;
}
//静态方法访问静态成员 不依赖实例,可以通过类直接调用
public static function write5(){
//静态成员属于类,不属于类实例,不能使用类实例的引用$this
return "国籍是:".self::$nation;
}
//备注:普通方法也可以访问静态成员,但不建议用,以后可能被删除了。
}
$user=new User;
echo "write1结果是:".$user->write1();
echo "<hr>";
echo "write2结果是:".$user->write2();
function hello(){
return "hello,world!";
}
$username="孤独求败";
echo "<hr>";
echo "write3结果是:".$user->write3();
$hello2=function(){
return "你好,哈哈!";
};
echo "<hr>";
echo "write4结果是:".$user->write4($hello2,$username);
echo "<hr>";
echo "write5结果是:".$user->write5();
//类成员的访问控制
class User{
//公共成员,类外部,内部,子类都可访问
public $name="张三";
//private 私有成员只能在类内部访问。外部和子类访问不到
private $age=20;
//protected 受保护成员 外部访问不到,类内部和子类可用。
protected $gongzi=8000;
public function write(){
return $this->name.$this->age.$this->gongzi;
}
}
//子类
class Rob extends User{
public function write(){
return $this->name.$this->age.$this->gongzi;
}
}
//类外部
$user=new User;
echo "类外部访问: ".$user->name;
echo "<hr>";
echo "类内部访问: ".$user->write();
echo "<hr>";
$rob=new Rob;
echo "子类访问: ".$rob->write();
//用下面的也可
//echo "子类访问: ".(new Rob)->write();
类可以被继承,类里面的属性和方法也可以被继承和扩展。
class User{
protected $name="张三";
public function write(){
return "名字是{$this->name}";
}
}
class Rob extends User{
//1.扩展
//属性扩展
protected $age=20;
//方法扩展
public function write(){
//parent::write() 调用父类的函数
return parent::write()."年龄是{$this->age}";
}
//2.重写
//属性重写
protected $name="李四";
//方法重写
// public function write(){
// return "名字是{$this->name}年龄是{$this->age}";
// }
}
$rob=new Rob;
echo $rob->write();
如果不想让方法被继承和重写,要在前面加上final。如final public function write()
不想让类被继承,在前面也加上final。如final class User