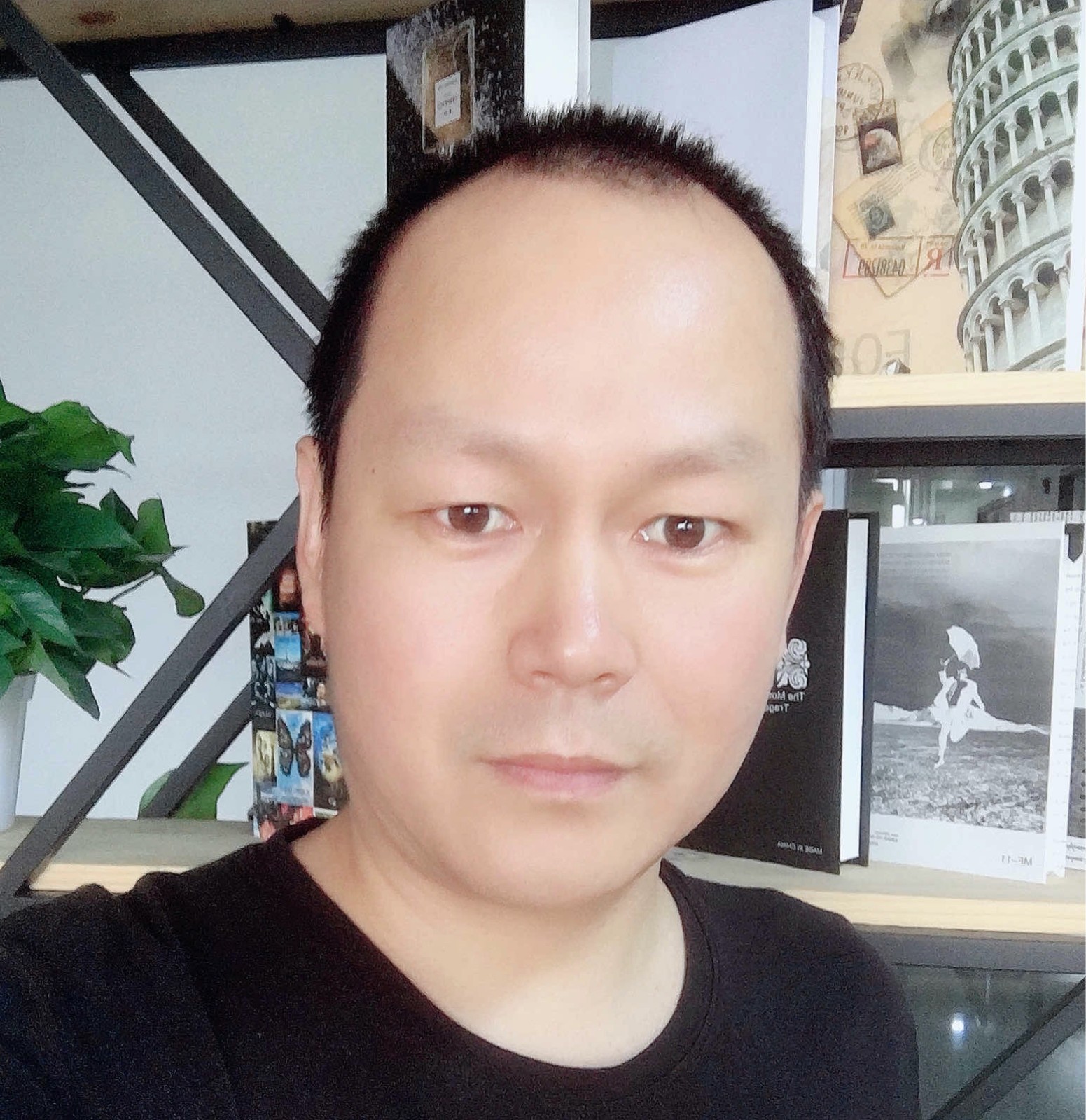
Correction status:qualified
Teacher's comments:不错
<!DOCTYPE html>
<html lang="zh-cn">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="css/demo3.css">
<title>轮播图案例</title>
</head>
<body>
<div class="container">
<img src="banner/banner1.png" alt="" data-index=1 class="slider active">
<img src="banner/banner2.png" alt="" data-index=2 class="slider">
<img src="banner/banner3.png" alt="" data-index=3 class="slider">
<img src="banner/banner4.png" alt="" data-index=4 class="slider">
<img src="banner/banner5.png" alt="" data-index=5 class="slider">
<img src="banner/banner6.png" alt="" data-index=6 class="slider">
<!-- 小圆点 -->
<div class="point-list"></div>
<!-- 翻页 -->
<span class="skip prev"><</span>
<span class="skip next">></span>
</div>
</body>
<script src="js/demo3.js"></script>
</html>
/* 轮播图CSS */
* {
margin: 0;
padding: 0;
box-sizing: content-box;
}
.container {
width: 1000px;
height: 300px;
margin: auto;
position: relative;
}
.container .slider {
width: 1000px;
height: 300px;
display: none;
}
.container .slider.active {
display: block;
}
.point-list {
width: 80px;
height: 20px;
position: absolute;
top: 280px;
left: 450px;
}
.point-list .point {
display: inline-block;
width: 10px;
height: 10px;
margin-right: 3px;
border-radius: 100%;
background-color: rgba(200, 200, 200, 0.7);
}
.point-list .point.active {
background-color: tomato;
}
.point-list .point:hover {
background-color: tomato;
cursor: pointer;
}
.skip {
font-size: 30px;
color: rgba(255, 255, 255, 0);
position: absolute;
}
.skip:hover {
color: tomato;
cursor: pointer;
background-color: rgba(200, 200, 200, 0.7);
}
.prev {
top: 130px;
left: 10px;
}
.next {
top: 130px;
right: 10px;
}
// 轮播图js
// 获取所有图片
var imgs = document.querySelectorAll("img");
// 获取小圆点组
var pointList = document.querySelector(".point-list");
// 动态生成小圆点
imgs.forEach(function (img, index) {
var span = document.createElement("span");
if (index == 0) {
span.classList.add("point", "active");
}
span.classList.add("point");
// 给当前小圆点添加自定义的 data-index
span.dataset.index = img.dataset.index;
pointList.appendChild(span);
});
// 获取所有小圆点
var points = document.querySelectorAll(".point");
// 给小圆点组添加点击事件(代理)
pointList.addEventListener("click", function (ev) {
// 遍历每个轮播图片
imgs.forEach(function (img) {
// 判断 index 的值是否与当前点击的小圆点 index 值相等
// 相等的话给默认小圆点添加样式 active
if (img.dataset.index === ev.target.dataset.index) {
// 先去掉已经高亮的小圆点样式
imgs.forEach(function (img) {
img.classList.remove("active");
});
// 添加默认小圆点高亮样式
img.classList.add("active");
// 设置与当前图片对应的小圆点高亮显示
// 由于这个功能要多处使用,声明为公共函数
setPointActive(img.dataset.index);
}
});
});
// 设置当前图片对应的小圆点高亮显示
function setPointActive(imgIndex) {
// 去掉已高亮的小圆点样式
points.forEach(function (point) {
point.classList.remove("active");
});
// 当前点击小圆点添加高亮
points.forEach(function (point) {
if (point.dataset.index === imgIndex) {
point.classList.add("active");
}
});
}
// ============图片自动播放=============
// 鼠标移出2秒后自动播放,鼠标移入时自动停止播放
// 获取图片父元素
var container = document.querySelector(".container");
// 向后翻页函数
function playImg() {
// 1.获取当前图片
var autoImg = null;
// 2.遍历图片
imgs.forEach(function (img) {
// 判断图片 class 中是否包含 active
if (img.classList.contains("active")) {
// 如果包含则更换当前图片
autoImg = img;
}
});
// 3.去掉当前图片的 active 属性
autoImg.classList.remove("active");
// 4.获取下一页图片
// nextElementSibling属性:返回指定元素的下一个兄弟元素
autoImg = autoImg.nextElementSibling;
// 如果存在后一张图片,添加 active 样式,否则进入循环现实第一张
if (autoImg !== null && autoImg.nodeName === "IMG") {
autoImg.classList.add("active");
} else {
// 循环获取下一页
autoImg = imgs[0];
autoImg.classList.add("active");
}
// 小圆点高亮
setPointActive(autoImg.dataset.index);
}
// 声明自动播放变量
var autoPlay = null;
// 给图片父元素添加鼠标移出事件(代理)
container.addEventListener("mouseover", function () {
clearInterval(autoPlay);
});
// 给图片父元素添加鼠标移入事件(代理)
container.addEventListener("mouseout", function () {
autoPlay = setInterval(playImg, 2000);
});
// ============翻页==============
// 获取翻页按钮
var skip = document.querySelectorAll(".skip");
// 给翻页按钮添加事件
skip.item(0).addEventListener("click", skipImg, false);
skip.item(1).addEventListener("click", skipImg, false);
// 翻页函数
function skipImg(ev) {
// 1.获取当前图片
var currentImg = null;
// 遍历图片
imgs.forEach(function (img) {
// 判断图片 class 中是否包含 active
if (img.classList.contains("active")) {
// 如果包含则更换当前图片
currentImg = img;
}
});
// 2.判断是否点击了前一页按钮
if (ev.target.classList.contains("prev")) {
// 去掉当前图片的 active 属性
currentImg.classList.remove("active");
// 如果点击前一页按钮就将当前显示图片替换成签一张图片
// previousElementSibling属性:返回指定元素的前一个兄弟元素
currentImg = currentImg.previousElementSibling;
// 如果存在前一张图片,添加 active 样式,否则进入循环现实最后一张
if (currentImg !== null && currentImg.nodeName === "IMG") {
currentImg.classList.add("active");
} else {
// 循环获取当前页
currentImg = imgs[imgs.length - 1];
currentImg.classList.add("active");
}
}
// 3.判断是否点击了下一页按钮
if (ev.target.classList.contains("next")) {
// 去掉当前图片的 active 属性
currentImg.classList.remove("active");
// 如果点击下一页按钮就将当前显示图片替换成后一张图片
// nextElementSibling属性:返回指定元素的下一个兄弟元素
currentImg = currentImg.nextElementSibling;
// 如果存在后一张图片,添加 active 样式,否则进入循环现实第一张
if (currentImg !== null && currentImg.nodeName === "IMG") {
currentImg.classList.add("active");
} else {
// 循环获取当前页
currentImg = imgs[0];
currentImg.classList.add("active");
}
}
// 小圆点高亮
setPointActive(currentImg.dataset.index);
}
1.翻页
2.鼠标移出自动播放,移入停止播放