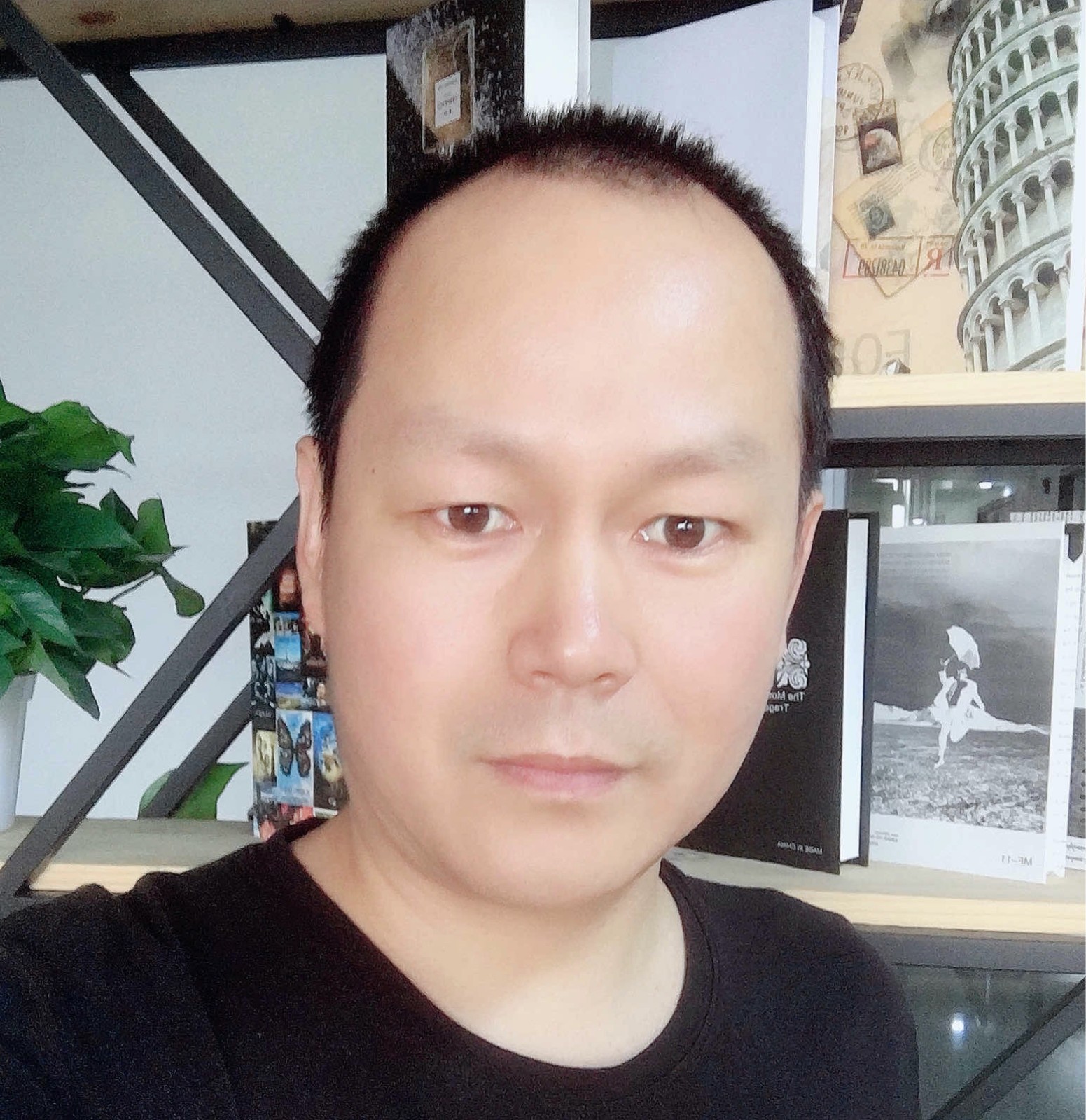
Correction status:qualified
Teacher's comments:mvc是现代所有框架的基础, 目前 流行的几乎都是它的扩展
composer require catfan/medoo
composer require league/plates
目录图片:
组件的具体使用查看组件的介绍文档,以下部分是core目录
的代码:
Controller.php
<?php
namespace core;
use core\View;
// 自定义核心控制器
abstract class Controller
{
// 每一个控制器都需要调用到视图组件,实例化存储到属性view中
protected $view = '';
// 构造函数,进行视图类的实例赋值到属性view
public function __construct()
{
$this->view = new View('app/views');
}
}
Model.php
<?php
// 模型类
namespace core;
// 模型类继承自 组件Medoo
use Medoo\Medoo;
abstract class Model extends Medoo
{
public function __construct()
{
$optinos = [
'database_type' => 'mysql',
'database_name' => 'phpedu',
'server' => 'localhost',
'username' => 'root',
'password' => 'root',
];
parent::__construct($optinos);
}
}
View.php
<?php
// 视图类
namespace core;
// 视图类继承自 plates组件
use League\Plates\Engine;
class View extends Engine
{ public $templates = null;
public function __construct($path)
{
$this->templates = parent::__construct($path);
}
}
以下是app目录
用户应用:controllers/UsersController.php
<?php
namespace controllers;
use core\Controller;
use models\UsersModel;
// 继承核心controller
class UsersController extends Controller
{
public function __construct()
{
// 可以不写,但最好调用一下父类的构造方法
parent::__construct();
}
// 首页展示方法
public function index()
{
$model = new UsersModel();
$users = $model ->select('users',['id','name','email'],['id[>]'=>20,'LIMIT'=>5]);
return $this->view->render('users/list',['users'=>$users]);
}
// 删除数据方法
public function delete()
{
$id = $_GET['id'];
$model = new UsersModel();
$result = $model->delete("users", [
"id" => $id
]);
// return __METHOD__;
return $result->rowCount();;
}
// 展示修改页面
public function edit()
{
$id = $_GET['id'];
$model = new UsersModel();
$user = $model->get("users", ['id','name','email'], [
"id" => $id
]);
return $this->view->render('users/edit',['user'=>$user]);
// return print_r($_GET,true);
}
// 更新数据方法
public function update()
{
$id = $_GET['id'];
$model = new UsersModel();
$data = $model->update("users", [
"name" => $_POST['name'],
"email" =>$_POST['email']
], [
"id" => $id
]);
return $data->rowCount();
// return print_r($_POST,true);
}
}
models/UsersModel.php
<?php
namespace models;
use core\Model;
// 继承核心的Model
class UsersModel extends Model
{
public function __construct()
{
parent::__construct();
}
// 自行创建model组件中没有的方法/定制式的方法
public function idGetDate()
{
return '自定义模型数据处理';
}
}
Views
文件夹主要存放的是视图文件,查看组件文档有几点重点:
users/list.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>用户信息</title>
<style>
body {
display: flex;
flex-direction: column;
align-items: center;
}
table {
border-collapse: collapse;
border: 1px solid;
width: 50%;
text-align: center;
}
th,
td {
border: 1px solid;
padding: 5px;
}
tr:first-child {
background-color: #eee;
}
</style>
</head>
<body>
<h3>用户管理系统</h3>
<table>
<tr>
<th>id</th>
<th>姓名</th>
<th>邮箱</th>
<th>操作</th>
</tr>
<?php foreach ($users as $user): ?>
<tr>
<!-- $this->e:模板引擎Plates中的一个函数,用来过滤特殊字符 -->
<td><?=$user['id']?></td>
<td><?=$this->e($user['name'])?></td>
<td><?=$this->e($user['email'])?></td>
<td><button onclick="location.href='/mvc/index.php/users/edit.html?id=<?=$user['id']?>'" >编辑</button>
<button onclick="del(<?=$user['id']?>)">删除</button></td>
</tr>
<?php endforeach ?>
</table>
<p>
<a href="">假分页</a>
<a href="">1</a>
<a href="">2</a>
<a href="">3</a>
<a href="">4</a>
</p>
</body>
<script>
function del(id){
let url = '/mvc/index.php/users/delete.html?id='+id;
return confirm('是否删除?') ?location.href=url : false;
}
</script>
</html>
users/edit.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>用户编辑页</title>
</head>
<body>
<form action="/mvc/index.php/users/update.html?id=<?=$this->e($user['id'])?>" method="POST" >
<fieldset>
<legend>用户编辑</legend>
<p>
<label for="">用户名:</label>
<input type="text" name="name" id="name" value="<?=$this->e($user['name'])?>" >
</p>
<p>
<label for="">邮箱:</label>
<input type="email" name="email" id="email" value="<?=$this->e($user['email'])?>">
</p>
<p>
<button>保存</button>
</p>
</fieldset>
</form>
</body>
</html>
创建入口文件index.php
<?php
//加载composer的自动加载文件
require __DIR__ . '/vendor/autoload.php';
// 路由访问拦截
$pathinfo = array_values(array_filter(explode('/', str_replace('.html','',$_SERVER['PATH_INFO']))));
// 如果要用路由拦截访问,不能使用USE进行文件别名,
$controller = '\\controllers\\' . ucfirst(array_shift($pathinfo)) . 'Controller';
$action = array_shift($pathinfo);
// 测试
// echo $controller.'-'.$action;
// printf('<pre>%s</pre>', print_r($pathinfo, true));
$params = [];
// 每次处理的是二个元素,所以$i = $i + 2
for ($i=0; $i < count($pathinfo); $i+=2) {
// $pathinfo中前一个元素是新数组中的键名, 后面一个是它的值
if (isset($pathinfo[$i + 1])) {
$params[$pathinfo[$i]] = $pathinfo[$i + 1];
}
}
echo call_user_func_array([(new $controller), $action], $params );