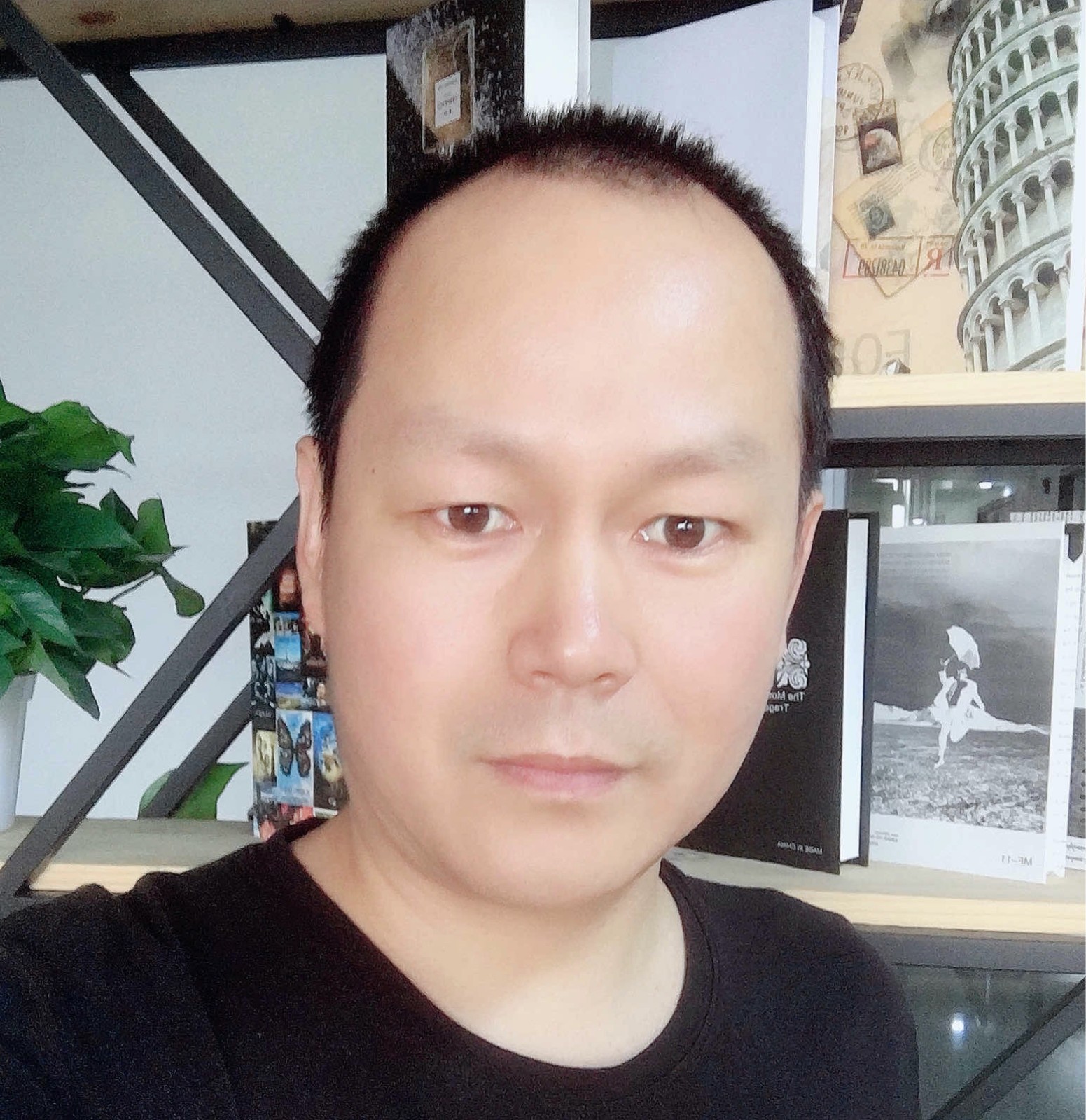
Correction status:qualified
Teacher's comments:文件上传是不是也挺简单的,有套路的
try{// 在try代码块内触发异常
// spl_autoload_register()通过回调自动加载外部文件
spl_autoload_register(function ($class){
// 把$class中的\替换成系统分隔符
$path=str_replace('\\', DIRECTORY_SEPARATOR, $class);
// DIRECTORY_SEPARATOR是一个显示系统分隔符的常量
$file=__DIR__. DIRECTORY_SEPARATOR .$path .'.php';
// is_file() 检查指定的文件是否是常规的文件;file_exists() 检查文件或目录是否存在
if(!(is_file($file) && file_exists($file)))
// 抛出异常
throw new Exception ('不是文件名或文件不存在');
// 文件存在即引入
require $file;
});
}catch(Exception $e){// 捕获异常
die($e->getMessage());
}
// 自动加载外部文件类
require __DIR__ .'/loader.php';// 引入loader文件
use inc\lib\Test1;// 类的别名与原始别名相同时,可以省去
use inc\lib\Test2 as Te;
echo Test1::$site, '<br>';
echo Te::$site, '<br>';
<?php
printf('<pre>%s</pre>',print_r($_FILES, true));
echo '<hr>';
//printf()输出格式化的字符串
// print_r()用于打印变量;第2个参数为true则不输出结果,将结果赋值给一个变量,false则直接输出结果
// $_FILES 是一个预定义的数组,用来获取通过 POST 方法上传文件的相关信息。如果为单个文件上传,那么 $_FILES 为二维数组;如果为多个文件上传,那么 $_FILES 为三维数组
// 一、
// 步骤1.获取错误代码
// $error=$_FILES['pic']['error'] ?? null;
// // 步骤2.判断错误代码
// if($error===0) echo '文件上传成功<hr>';
// elseif($error===1) echo '超过了php.ini中的大小<hr>';
// elseif($error===2) echo '超过了上传表单中的MAX_FILE_SIZE值的大小<hr>';
// elseif($error===4) echo '文件上传失败<hr>';
// 二、
// 1.获临时文件类型
// $type = $_FILES['my_pic']['type'];
// // strstr() 函数搜索字符串在另一字符串中的第一次出现
// if (strstr($type, '/', true) !== 'image') echo '文件类型正确<hr>';// 搜索/在$type中第一次出现之前的字符串部分
// 三、
// 1.获取临时文件名
// $tmp=$_FILES['pic']['tmp_name'];
// // 2. is_uploaded_file()判断 文件是否是通过POST上传的?
// if (is_uploaded_file($tmp)) echo '上传文件安全<hr>';
// else echo '非POST<hr>';
// 四、图片上传
// 1.获取临时文件名
$tmpFile=$_FILES['pic']['tmp_name'];
// uploads/ :用户自定义目录,文件存放处
// echo strstr($_FILES['pic']['name'], '.');// 获取文件后缀.jpg
// 获取最终文件名,用md5对原始文件名进行加密
// echo md5($_FILES['pic']['name']).'<hr>';
// echo $_FILES['pic']['name'].'<hr>';
// echo strstr($_FILES['pic']['name'], '.').'<hr>';
$destFileName = './uploads/' .md5($_FILES['pic']['name']).strstr($_FILES['pic']['name'], '.');
// echo $destFileName.'<hr>';
// move_uploaded_file(临时文件名,正式路径)
if (move_uploaded_file($tmpFile, $destFileName)){
echo '<img src="'. $destFileName .'" width="200px;">';// 对图片进行预览
echo '<p>上传成功了</p>';
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>单文件上传</title>
</head>
<body>
<!-- 文件上传必须满足2个条件:1、method:post;2、enctype: multipart/form-data -->
<form action="" method="post" enctype="multipart/form-data">
<fieldset><!-- 对表单中的相关元素进行分组 -->
<legend>文件上传</legend>
<!-- 设置允许上传的文件大小, 这个隐藏域必须写到input:file -->
<!-- <input type="hidden" name="MAX_FILE_SIZE" value="5000"/> -->
<input type="file" name="pic" id=""/>
<button>上传</button>
</fieldset>
</form>
</body>
</html>
<?php
printf('<pre>%s</pre>',print_r($_FILES, true));
// 将文件从临时目录中移动到正式的目录中
// move_uploaded_file(临时文件, 正式路径)
// 1.获临时文件名
// $tmpFile = $_FILES['my_pic']['tmp_name'];
// 最终文件名
// echo strstr($_FILES['my_pic']['name'], '.');
foreach ($_FILES as $file) {
if ($file['error'] === 0) {
$destFileName = './uploads/' . md5($file['name']). strstr($file['name'], '.');
if (move_uploaded_file($file['tmp_name'], $destFileName)){
echo '<p>上传成功了</p>';
echo '<img src="'. $destFileName .'" width="200">';
}
}
}
// die;
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>多文件上传</title>
</head>
<body>
<form action="" method="post" enctype="multipart/form-data">
<fieldset>
<legend>单文件上传</legend>
<input type="file" name="my_pic1" id="">
<input type="file" name="my_pic2" id="">
<input type="file" name="my_pic3" id="">
<button>上传</button>
</fieldset>
</form>
</body>
</html>