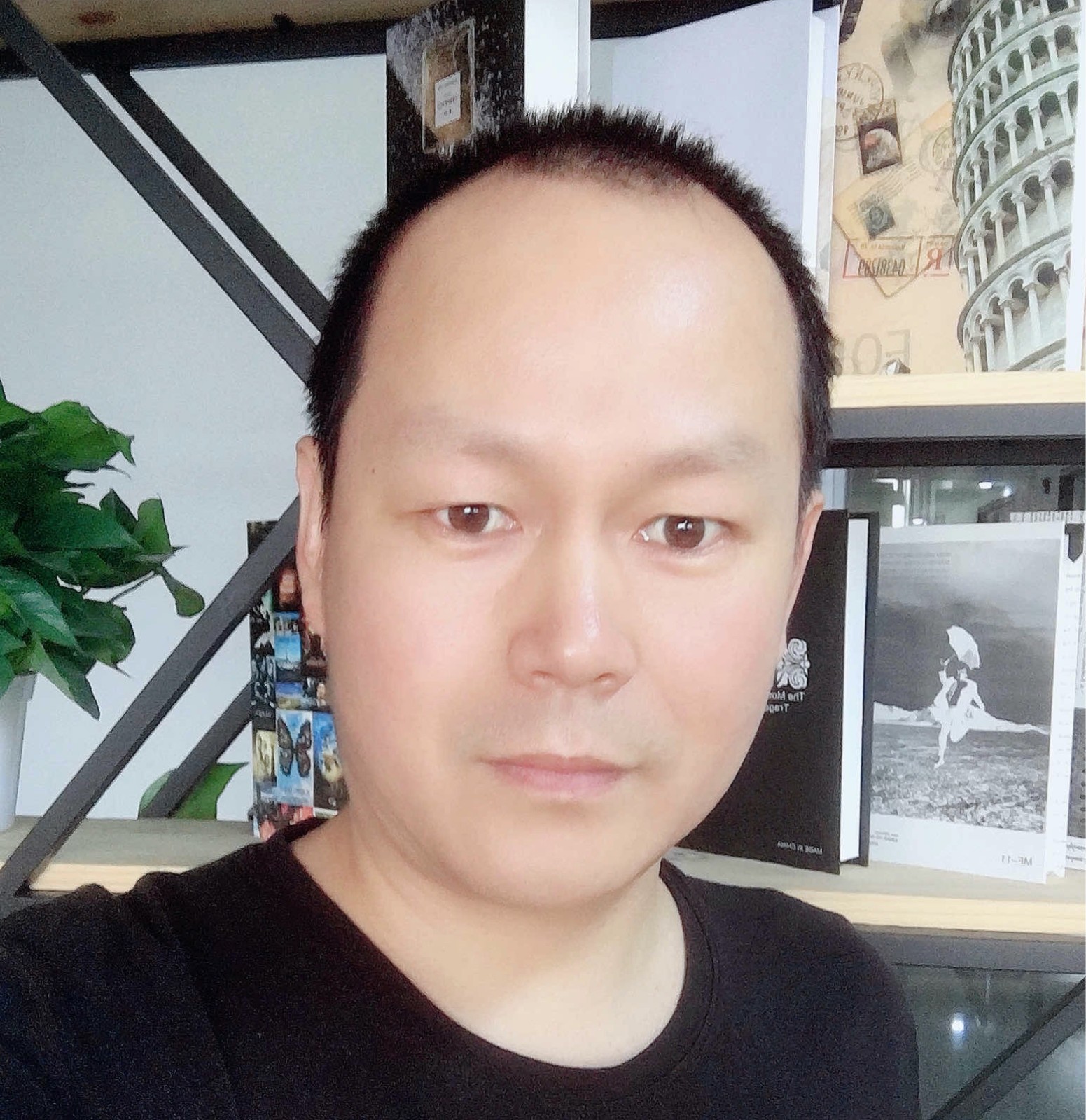
Correction status:qualified
Teacher's comments:以后凡是遇到多行字符串, 和字符串与变量的组成,尽可能使用模板字符串
Javascript 作为一门强大的脚本语言,主要是用于前端开发。相比于其他脚本语言来讲,是有一定难度,所以要想学好它就必须下足功夫。对于如何学好一门语言想必大家都有各自的一套办法,但是方法再五花八门,有一点一定是相同的——那就是打好基础!下面来介绍两个重要的基础知识:
- 模板字面量和标签函数
- 对象和数组的解构
什么是字面量?
官方定义是:在计算机科学中,字面量(literal)是用于表达源代码中一个固定值的表示法。
我的理解是:所谓字面量就是你看到的某种语言的数据类型的一种展现形式。
比如在 JavaScript 中
// 字面量
let name = "zhanshan";
let age = 30;
const student = {
id: 1,
class: "三年二班",
name: "zhou",
};
等号右边的zhanshan
、30
、{ id: 1, class: "三年二班", name: "zhou", }
这几种“形式”分别表示字符串
、数值
、对象
类型的数据。
我们一看到它们的“长相”就知道它们是谁。
在引出这个概念之前先来看个东西。
在 ES6 之前,将字符串连接到一起的方法是+
或者concat()
方法,如
let name = "lisi";
let tel = 13866668888;
let str = "我的名字是:" + name + " 电话号码是:" + tel;
console.log(str); //我的名字是:lisi 电话号码是:13866668888
let a = "hello";
let hellowWorld = a.concat(" world!");
console.log(hellowWorld); // hello world!
ES6 之后,我们可以使用模板字面量来让这些拼接字符串更为方便的表达。
如
let name = "lisi";
let tel = 13866668888;
let str = `我的名字是:${name} 电话号码是:${tel}`;
console.log(str); //我的名字是:lisi 电话号码是:13866668888
let a = "hello";
let hellowWorld = `${a} world!`;
console.log(hellowWorld); // hello world!
是不是方便了很多?不用担心过引号写错,不用担心加号没放对地方。
什么是模板字面量?
模板字面量本质上是包含嵌入式表达式的字符串字面量.
模板字面量用倒引号 ( `` )(而不是单引号 ( ‘’ ) 或双引号( “” ))表示,可以包含用 \${expression} 表示的占位符
模板字面量中,变量或表达式叫”插值”, 变量和表达式必须返回一个值
用来自定义模板字面量中的插值的行为的一个函数。
function selfIntroduce(literal, ...val) {
// console.log(literal);
// console.log(Array.isArray(literal));
// console.log(val);
let info = `${literal[0]}${val[0]}${literal[1]}${val[1]}${literal[2]}${literal[3]}${val[2]}`;
return info;
}
let name = "zhangshan";
let age = 20;
let city = "shanghai";
let result = selfIntroduce`my name is ${name},i am ${age} years old , i am come from ${city}`;
console.log(result); //my name is zhangshan,i am 20 years old , i am come from shanghai
函数的第一个参数必须是模板字面中的原始字符串的内容组成的数组
第一个参数可以看作是用
${...}
符号切割模板字面量(字符串)组成的一个数组。
从第二个参数开始,与模板字面量中的插值一一对应
例子中我使用了 rest 语法(…val),如果不用可以写成离散型参数:val1,val2,val3…。
什么是解构赋值?
解构赋值允许你使用类似数组或对象字面量的语法将数组和对象的属性赋给各种变量。这种赋值语法极度简洁,同时还比传统的属性访问方法更为清晰。
通常我们如果想把一个数组或者对象的属性值赋予给几个变量时都是如下这样做
const arr = ["a", 10, "b"];
let a = arr[0];
let b = arr[1];
let c = arr[2];
现在通过解构赋值可以这样
const arr = ["a", 10, "b"];
[a, b, c] = arr;
console.log(a, b, c);
是不是代码更为简洁优雅?
解构赋值通常针对的是复合数据类型才有意义,比如对象和数组。
通过解构对象,你可以把它的每个属性与不同的变量绑定。
const { variable1, variable2, ..., variableN ]} = object;
或者
({ variable1, variable2, ..., variableN ]} = object;)
因为 JavaScript 语法通知解析引擎将任何以
{
开始的语句解析为一个块语句(例如,{console}
是一个合法块语句)。解决方案是将整个表达式用一对小括号包裹
(一)正常解构
js 代码
//定义数组
const obj = {
name: "zhangshan",
age: "30",
city: "shanghai",
sex: "male",
married: true,
};
//解构
({ name, age, city, sex, married } = obj);
console.log(
"name:",
name,
"age:",
age,
"city:",
city,
"sex:",
sex,
"married:",
married
);
console.log("-----------------------");
// 别名解构
({ name, age, city, sex: gentleman, married } = obj);
console.log(
"name:",
name,
"age:",
age,
"city:",
city,
"gentleman:",
gentleman,
"married:",
married
);
结果
(二)嵌套
js 代码
const obj = {
info: ["zhangshan", "30", "shanghai", "male"],
married: true,
};
const { info, married } = obj;
console.log("info", info);
console.log("married", married);
结果
什么时候是不完全解构?个人理解是=
两边变量和数组的个数不一致时就会导致不完全解构的现象
js 代码
const obj = {
name: "zhangshan",
age: "30",
city: "shanghai",
sex: "male",
married: true,
};
//变量名和值对不上
({ name, age, city, sex, married, others } = obj);
console.log(
"name:",
name,
"age:",
age,
"city:",
city,
"sex:",
sex,
"married:",
married,
"others:",
others
);
console.log("-----------------------");
//默认值
({ name, age, city, sex, married, edu = "大专" } = obj);
console.log(
"name:",
name,
"age:",
age,
"city:",
city,
"sex:",
sex,
"married:",
married,
"edu:",
edu
);
console.log("-----------------------");
// rest语法
({ name, ...other } = obj);
console.log("name:", name);
console.log("other:", other);
结果
对象的属性名要与变量名一致,要不然无法解构。还有在对象解构中无法使用数组解构时的对位解构(前面写几个逗号留空)
如下
const obj = {
name: "zhangshan",
age: "30",
city: "shanghai",
sex: "male",
married: true,
};
// 变量名与属性名不一致,无法解构
const { a, b, c, d, e } = obj;
console.log(a, b, c, d, e);
结果
[ variable1, variable2, ..., variableN ] = array;
这将为 variable1 到 variableN 的变量赋予数组中相应元素项的值。如果你想在赋值的同时声明变量,可在赋值语句前加入 var、let 或 const 关键字
var [ variable1, variable2, ..., variableN ] = array;
let [ variable1, variable2, ..., variableN ] = array;
const [ variable1, variable2, ..., variableN ] = array;
事实上,用变量来描述并不恰当,因为你可以对任意深度的嵌套数组进行解构
var [a, [[b], c]] = [1, [[2], 3]];
console.log(a);
// 1
console.log(b);
// 2
console.log(c);
// 3
js 代码:
const arr = ["a", 10, "b", false];
[a, b, c, d] = arr;
console.log(a, b, c, d);
结果:
=
两边变量和数组的个数不一致时就会导致不完全解构的现象
js 代码:
//等号左边留两个空位,其余对位解构
const arr = ["zhangshan", 30, "shanghai", "male"];
[, , city, sex] = arr;
console.log("city: " + city);
console.log("sex: " + sex);
//rest 语法
[name, ...other] = arr;
console.log("-----------------------");
console.log(name, other);
console.log("name: " + name);
console.log("other: " + other);
//默认值
[name, age, city, sex, married = true] = arr;
console.log("-----------------------");
console.log(name, age, city, sex, married);
结果:
加油,多敲代码!
参考链接:https://www.cnblogs.com/cheng-du-lang-wo1/p/7259343.html