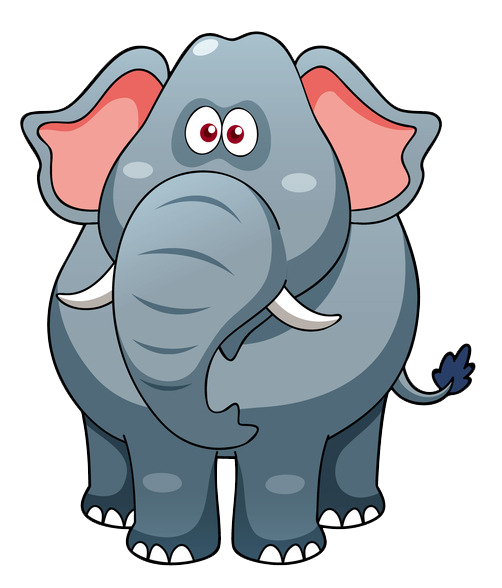
Correction status:qualified
Teacher's comments:完成的不错, 总结的很好; 下次的作业标题不用带上时间, 可以根据作业内容取标题; 并且下次在"我的课程作业"中提交作业
实例演示分支的不同类型,注意else的本质
js
中的数据类型主要有以下两种
原始数据类型:包含数值/字符串/布尔/undefined/null
数组/对象/函数
let colors = new Array();
console.log(typeof colors);//输出object
(1) 创建一个长度为10的数组
colors = new Array(20);
console.log(typeof colors);//输出object
(2) 创建一个含有元素的数组
colors = new Array('red','blue','green');
console.log(typeof colors);//输出object
(3) 使用Array构造函数时候,也可以省略new操作符
colors = Array('red','blue','green');
console.log(typeof colors);//同样输出object
array literal
// 用中括号表示的数组字面量
colors = ['red','blue','green'];
let names = [];
let values = [1,2];
(1)多维数组
forEach
方法为例
const arr1 = [
['豪宅','游艇','大美女'],
['跑车','房车','越野车'],
['美元','欧元','人民币'],
];
// 遍历,forEach()的参数是一个回调函数
arr1.forEach(
function(item,key){
console.log(key + "=>" + item)
});
/* 输出结果:
0=>豪宅,游艇,大美女
1=>跑车,房车,越野车
2=>美元,欧元,人民币
*/
(2)对象数组
json
格式的数据.
const arr2 = [
{'hz':'豪宅','yt':'游艇','mv':'大美女'},
{'pc':'跑车','fc':'房车','yy':'越野车'},
{'my':'美元','oy':'欧元','rm':'人民币'},
];
arr1.forEach(function(item,key){console.log(key + "=>" + item)});
/* 输出结果:
0=>豪宅,游艇,大美女
1=>跑车,房车,越野车
2=>美元,欧元,人民币
*
(3)类数组
length
属性Array构造函数的成员行数from
转化为数组
const obj1 = {
'hz':'豪宅',
'yt':'游艇',
'mv':'大美女',
'length':3,
};
Array.from(obj1).forEach(function(item){console.log(item)});
/*输出结果
豪宅
游艇
大美女
*/
(4)函数数组
// * 数组成员是函数
const events = [
function () {
return '准备发射'
},
function () {
return '击中目标'
},
function () {
return '敌人投降'
},
]
events.forEach(ev => console.log(ev()))
/*输出结果
准备发射
击中目标
敌人投降
*/
js中的分支结构一般分为条件分支结构和循环分支结构
if,if-else,if-elseif-else,swith
等;for,forEach,for-in,for-of
等; 1.if
条件结构
const dt = '9月30日';
if(dt == '9月30日'){
console.log('明天是国庆节,放假7天!')
}
//输出结果:明天是国庆节,放假7天!
2.if-else
结构
let num1 = 5;
if(num1 %2 == 0){
console.log(num1 + '是偶数')
}else{
console.log(num1 + '是奇数')
}
//输出结果:5是奇数
console.log((num1 % 2 == 0) ? 'num1是偶数':'num1是奇数');
//输出结果:5是奇数
3.if-elseif-else
结构
grade = 'D'
if (grade === 'A') {
console.log('优秀')
} else if (grade === 'B') {
console.log('良好')
} else if (grade === 'C') {
console.log('合格')
} else if (grade === 'D') {
console.log('补考')
} else {
console.log('非法输入')
}
console.log('-------------')
//输出结果:补考
4.switch
结构
grade = 'E';
switch(grade){
case 'A' :
console.log('优秀');
break;
case 'B' :
console.log('良好');
break;
case 'C' :
console.log('及格');
break;
case 'D' :
console.log('补考');
break;
default:
console.log('非法输入');
}
// 输出结果:非法输入
switch(true){}
let score = 95
// switch(true) 才能进入到代码体
switch (true) {
case score >= 80 && score <= 100:
console.log('优秀')
break
case score >= 70 && score < 80:
console.log('良好')
break
case score >= 60 && score < 70:
console.log('合格')
break
case score > 0 && score < 60:
console.log('补考')
break
default:
console.log('非法输入')
//输出结果:优秀