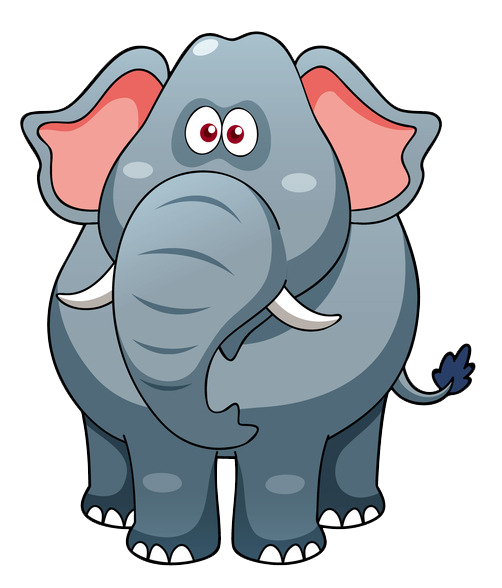
Correction status:qualified
Teacher's comments:作业一直完成的都很好, 继续加油
数组解构赋值
let [brand,price] = ['苹果手机',8848];
console.log(brand,price);
// 苹果手机 8848
// 更改数组参数的值
[brand,price] = ['Huawei',8008];
console.log(brand,price);
// Huawei 8008
console.log('--------------');
// 1. 值数量 < 变量数量, 默认值
[brand, price, stock = 99] = ['Xiaomi', 9988];
console.log(brand, price, stock);
// Xiaomi 9988 99
// 2. 值数量 > 变量数量, ...rest
[brand, ...arr] = ['Oppo', 6688, 99];
console.log(brand, arr);
// Oppo [ 6688, 99 ]
console.log(brand, ...arr);
// Oppo 6688 99
console.log('--------------');
console.log('-----交换变量----');
//? 应用场景: 交接二个数
let x = 10
let y = 20
console.log(`x = ${x}, y = ${y}`);
[y, x] = [x, y];
console.log(`x = ${x}, y = ${y}`);
对象解构赋值
// ? 变量名 === 属性名
let { brand, price } = { brand: "Apple", price: 5000 };
console.log(brand, price); // Apple 5000
({ brand, price } = { brand: "华为", price: 6000 });
console.log(brand, price); // 华为 6000
let { stock, price: uniPrice } = { stock: 100, price: 8000 };
console.log(stock, uniPrice); // 100 8000
console.log('-----------------');
// ? 应用场景1: 克隆对象
let phone = { brand: 'Vivo', price: 8008 };
console.log(phone); // { brand: 'Vivo', price: 8008 }
let { ...obj } = phone;
console.log(obj); // { brand: 'Vivo', price: 8008 }
console.log('-----------------')
// ? 应用场景2 : 解构传参
let show = function (phone) {
// user 是一个对象
return `手机品牌: ${phone.brand} 价格:${phone.price}元`
}
phone = { brand: '诺基亚', price: 198 }
console.log(show(phone)) // 手机品牌: 诺基亚 价格:198元
// * 使用对象解构进行简化传参
show = function ({ brand, price }) {
// user 是一个对象
return `手机品牌: ${brand} 价格:${price}元`
}
phone = { brand: '索尼', price: 498 }
console.log(show(phone)) // 手机品牌: 索尼 价格:498元
样例
let phone = {
info : {
brand : 'Huawei',
price : 998,
},
//访问器属性写法,前边加个get
//如 get getInfo()...;后续如果调用,只需要写 .getInfo即可,区别于.getInfo()
getInfo() {
return {
brand: this.info.brand,
price: this.info.price
}
},
//访问器属性写法,前边加个set
// 如 set setPrice(price)...;后续如果调用,则需要用.setPrice = value,区别于.setPrice(value)
setPrice(price){
if(price >= 100 && price <=20000){
this.info.price = price
}else{
console.log("你这手机价格就离谱")
}
},
}
console.log(phone.info.price)
// 998
console.log(phone.getInfo())
// { brand: 'Huawei', price: 998 }
phone.setPrice(699)
console.log(phone.getInfo())
// { brand: 'Huawei', price: 699 }
个人认为,
访问器属性和普通属性的区别在于:访问器属性增加对象的”函数方法”.
联系在于:当自定义了访问器属性值的configurable=false
,则不可以被转化为普通属性;普通属性在被转化为访问器属性后,普通属性的value和writable就会被废弃
以下内容不是很理解,先放在这里
数据属性有 value
,configurable
,enumerable
和writable**
Value
包含这个属性的数据值。读取属性值的时候,从这个位置读取,写入值得时候,把新值保存在这个位置。这个特性得默认值为undefined
Configurable
表示能否通过delete删除属性从而重新定义属性,能否修改属性的特性,或者能否把属性修改为访问器属性。直接在对象上定义的属性,它们的这个特性默认值为true
Enumerable
表示能否通过for-in循环返回属性,直接在对象上定义的属性,它们的这个特性默认值为true
Writable
表示能否修改属性的值,直接在对象上定义的属性,它们的这个特性默认值为true
访问器属性有get
,set
,其他还有configurable
和enumerable
(类同数据属性)
get
set
普通属性可以和访问器属性互相转化[前提比较多,比如必须是通过Object.defineProperty来进行操作]
Array.prototype.map()
和Array.prototype.forEach()
区别
forEach()
针对每一个元素执行提供函数,不会返回执行结果
map()
创建一个新的数组,其中每个数组由调用数组中的每一个元素执行提供函数得来,即:得到一个新数组,并返回
let arr = [1,2,3,4,5]
arr.forEach((num,index) =>{
return (arr[index] = num * 2)
})
console.log(arr) // arr = [2,4,6,8,10]
let doubled = arr.map(num => {
return num*2
})
console.log(doubled)
// doubled = [4,8,10,16,20] ,
// 因为forEach 已经让arr翻倍了
// 这时候如果打印arr,仍然是[2,4,6,...]
为什么模板函数中字符串字面量数组长度=变量数组长度+1
是为了保证返回值肯定是一个拼接字符串(需要借助’ ‘来和数字一起拼字符串),举个极端的例子
// 声明一个模板函数
const sum = function (strings, ...args) {
// 字面量数组
console.log(strings)
console.log(strings.length)
// 变量表达式数组
console.log(args)
console.log(args.length)
}
let num = 10
sum `${num}`
// 打印结果如下
// [ '', '' ]
// 2
// [ 10 ]
// 1