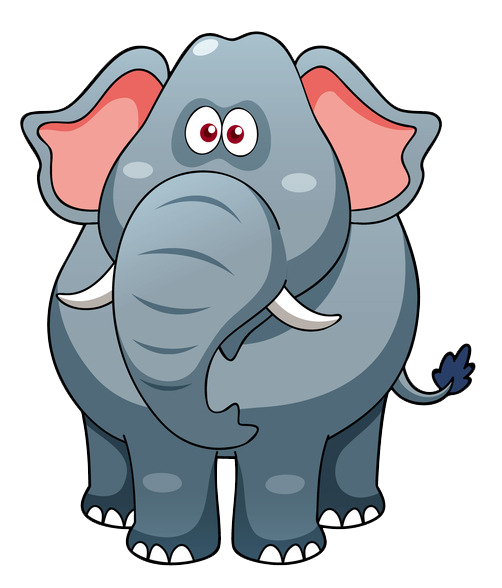
Correction status:qualified
Teacher's comments:
实现一个含有图片及图片指示符的轮播图,主要要几个方面:
1.图片的动态加载,从js里调入各种图片的信息,如图片源、图片指向的链接等;
2.实现按钮的动态生成;
3.样式的加载与取消 classList.add()和classList.remove等;
4.图片和按钮的自动显示与隐藏等;
5.图片和按钮的自动轮播,数组的自动循环,shift()和push()的应用
<body>
<div class="slidershow">
<div class="imgs"></div>
<div class="btns"></div>
</div>
<script type="module">
// 获取图片和按钮容器
const imgs = document.querySelector('.imgs');
const btns = document.querySelector('.btns')
// 从外部导入函数,别名为imagesSlider,注意,使用时要采取 "imagesSlider.xxx"的形式
import * as imagesSlider from "./js/slider.js";
// 已有代码加载完成后,加载图片和按钮等
window.onload = ()=>{
// 加载图片组
imagesSlider.createImage(imgs);
// 加载按钮
imagesSlider.createBtns(imgs,btns);
// 创建按钮事件
[...btns.children].forEach(function(btn){
btn.onclick = function(){
imagesSlider.switchImg(this,imgs);
}
})
// [...btns.children].forEach(btn =>(btn.onclick = () => imagesSlider.switchImg(this,imgs)));不能用箭头函数,在箭头函数里,this表示undefiend。
//自动播放
// 按钮数组,三个span
const btnArr = [...btns.children];
const btnKeys =Object.keys(btnArr);
setInterval(
function(btnArr,btnKeys){
imagesSlider.timePlay(btnArr,btnKeys);
},2000,btnArr,btnKeys);
}
</script>
</body>
// the information of the picture
const imgArr = [
{
key:1,
src:"images/item1.jpg",
url:"https://www.jacgoo.com"
},
{
key:2,
src:"images/item2.jpg",
url:"www.php.cn"
},
{
key:3,
src:"images/item3.jpg",
url:"www.baidu.com"
}
]
// 产生图片的函数
function createImage(imgs){
// 产生一个文档片段
console.log("------------------------")
const frag = new DocumentFragment();
for (let i = 0;i < imgArr.length;i++){
console.log(imgArr[i].src);
// 创建图片
const img = new Image();
img.src = imgArr[i].src;
// 创建dataset属性
img.dataset.key = imgArr[i].key;
if(i === 0) img.classList.add('active');
// 添加事件
img.onclick = () => (location.href = imgArr[i].url);
// 添加图片到片段中
frag.append(img);
}
// 片段图片添加到图片容器中
imgs.append(frag);
}
//产生按钮的函数
function createBtns(imgs,btns){
// 计算出所有图片的数量,根据这个来创建相同数量的按钮
const imagesLength = imgs.childElementCount;
console.log("button---------------")
for(let i = 0;i < imagesLength;i++){
const btn = document.createElement('span');
// 按钮索引:data-key,必须与图片索引一致
btn.dataset.key = imgs.children[i].dataset.key;
// 第一个按钮处于激活状态
if(i === 0 ) btn.classList.add('active')
btns.append(btn);
}
}
//更换图片函数
function switchImg(currentbtn,imgs){
// 去掉图片和按钮的激活状态
[...currentbtn.parentNode.children].forEach(btn => btn.classList.remove('active'));
[...imgs.children].forEach(img=>img.classList.remove('active'));
// 将当前的按钮处于激活状态
currentbtn.classList.add('active');
// 根据索引,找到当前图片并显示出来,用链式写法实现。
const currImg = [...imgs.children].find(img => img.dataset.key === currentbtn.dataset.key).classList.add('active');
}
//自动播放图片
function timePlay(btnArr,btnKeys){
// 取数组的第一个
let key = btnKeys.shift();
// 根据索引找到对应的按钮,再给他自动派发一个点击事件
btnArr[key].dispatchEvent(new Event('click'));
// 把刚才导出的按钮再从尾部进入,实现首尾相连
btnKeys.push(key);
}
导出四个函数
export {createImage,createBtns,switchImg,timePlay};
/* 图片 */
.slidershow {
width:240px;
height:360px;
}
.slidershow img {
width:100%;
height:100%;
border-radius: 10px;
display: none;
}
.slidershow img.active{
display: block;
}
/* 按钮容器 */
.slidershow .btns {
display:flex;
place-content: center;
transform:translateY(-40px);
}
.slidershow .btns > span {
background-color:gold;
height: 16px;
width: 16px;
border-radius: 50%;
margin:5px;
}
.slidershow .btns > span.active {
background-color: orangered;
}
.slidershow .btns > span:hover {
cursor:pointer;
}