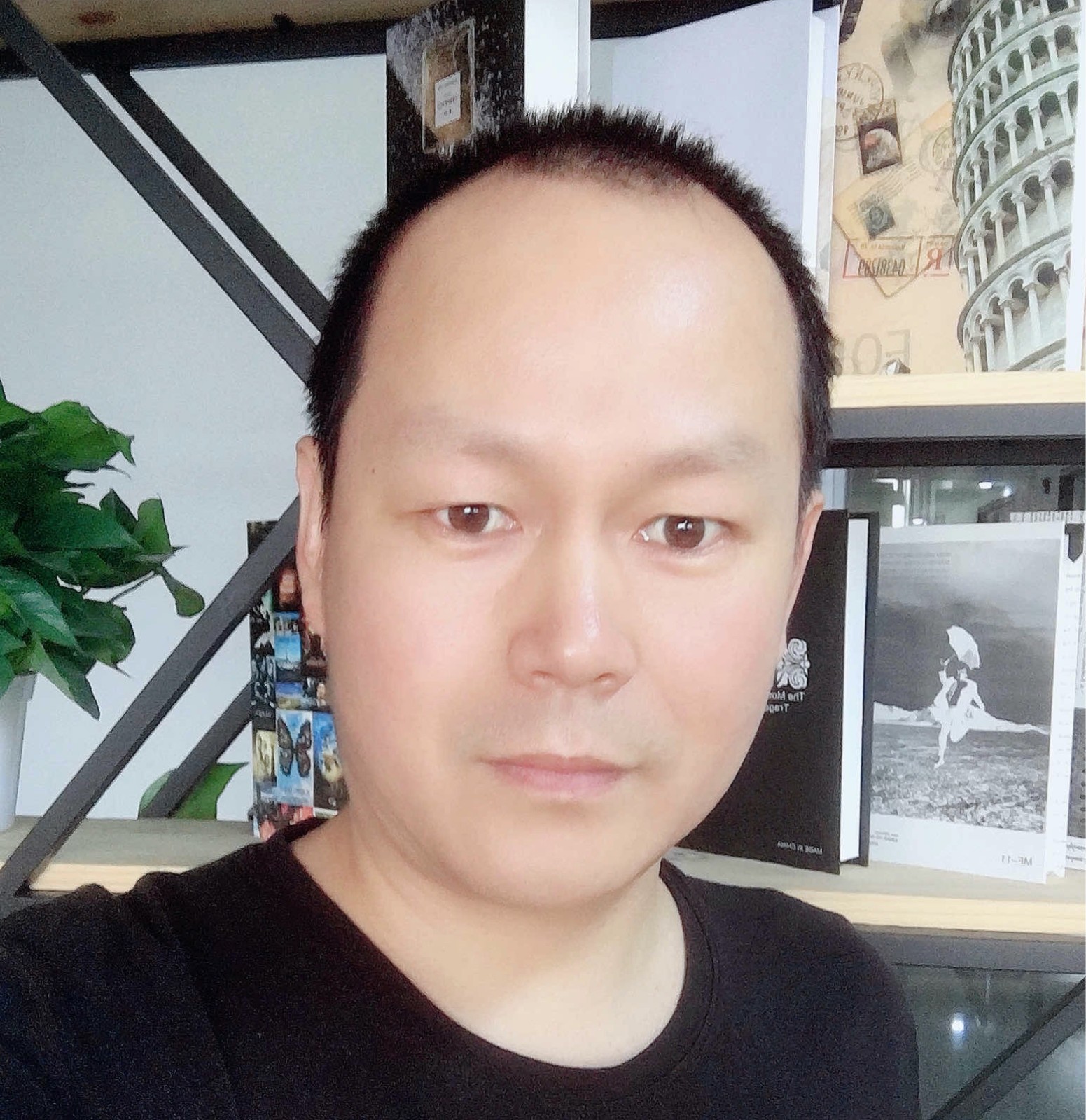
Correction status:qualified
Teacher's comments:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>对象和数组解构</title>
</head>
<body>
<script>
//解析要求等于2边类型相同
({ name, age } = { name: "誓言", age: 22 });
console.log(name, age);
//支持默认值
({ UserName, Age, Sex = "男" } = { UserName: "誓言1", Age: 28 });
console.log(UserName, Age, Sex);
//支持别名
({ UserName: 姓名, Age, Sex = "男" } = { UserName: "誓言1", Age: 28 });
console.log("姓名", Age, Sex);
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>对象和数组解构</title>
</head>
<body>
<script>
//解析要求等于2边类型相同
({ name, age } = { name: "誓言", age: 22 });
console.log(name, age);
//支持默认值
({ UserName, Age, Sex = "男" } = { UserName: "誓言1", Age: 28 });
console.log(UserName, Age, Sex);
//支持别名
({ UserName: 姓名, Age, Sex = "男" } = { UserName: "誓言1", Age: 28 });
console.log("姓名", Age, Sex);
//数组解构
const color = ["red", "bule", "green"];
let [c1, c2, c3] = color;
console.log(c1, c2, c3);
//多维数组解构
let [a, [b, c], d] = [1, [2, 3], 4];
console.log(a, b, c, d);
//数组也支持默认值
let [x, y, z = 100] = [100, 200];
console.log(x, y, z);
</script>
</body>
</html>
let score = 70 ;
if(score >= 60) console.log("及格了");
if(score >= 60) console.log("及格了")
else console.log("不及格");
if (score >= 60 && score <= 80) console.log('合格');
else if (score >= 81 && score <= 100) console.log('学霸');
else if (score >= 100 && score <= 0) console.log('分数无效');
else console.log("不及格");
let response = 'Success';
//单值字符串需要格式统一化,使用.tolowerCase()方法,全转为大写或小写
switch(response.toLowerCase()){
case 'fail':
console.log('请求失败');
break;
case 'success':
console.log('请求成功');
break;
case 'error':
console.log('参数错误');
break;
default:console.log('未知错误');
}
(判断)? true : false;
let score = 20;
let str = (score >= 60) ? '及格了' :'补考吧';
console.log(str);
一般用来遍历数组
可以用arr.length
来获取数组长度
const arr = [1,2,3,4,5];
let i = 0;
while(i < arr.length){
console.log(arr[i]);
i++;
}
do...while
do…while不管条件成立不成立都会执行一次,而while只有条件成立都会执行
do{
console.log(arr[i]);
i++;
}while(i < arr.length);
语法:
for(初始化循环变量;设置循环条件;更新循环条件){...}
const arr = [1,2,3,4,5];
for(let i = 0;i < arr.length; i ++){
//从第三个开始输出
if(i >= 3) console.log(arr[i]);
//或者
if(i < 3) baeak;
console.log(arr[i]);
//continue调出
if(arr[i] % 2 ===0) continue;
console.log(arr[i]);
}
for in
const arr = [1,2,3,4,5];
const obj = {
id:10000,
userName:'誓言',
email:'php@php.cn'
}
for(let key in obj){
console.log(`${key} => ${obj[key]}`);
}
for of
for(let val of arr){
console.log(val);
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<script>
const arr = [1, 2, 3, 4, 5];
const obj = {
id: 10000,
userName: "誓言",
email: "php@php.cn",
};
for (let key in obj) {
console.log(`${key} => ${obj[key]}`);
}
for (let val of arr) {
console.log(val);
}
</script>
</body>
</html>