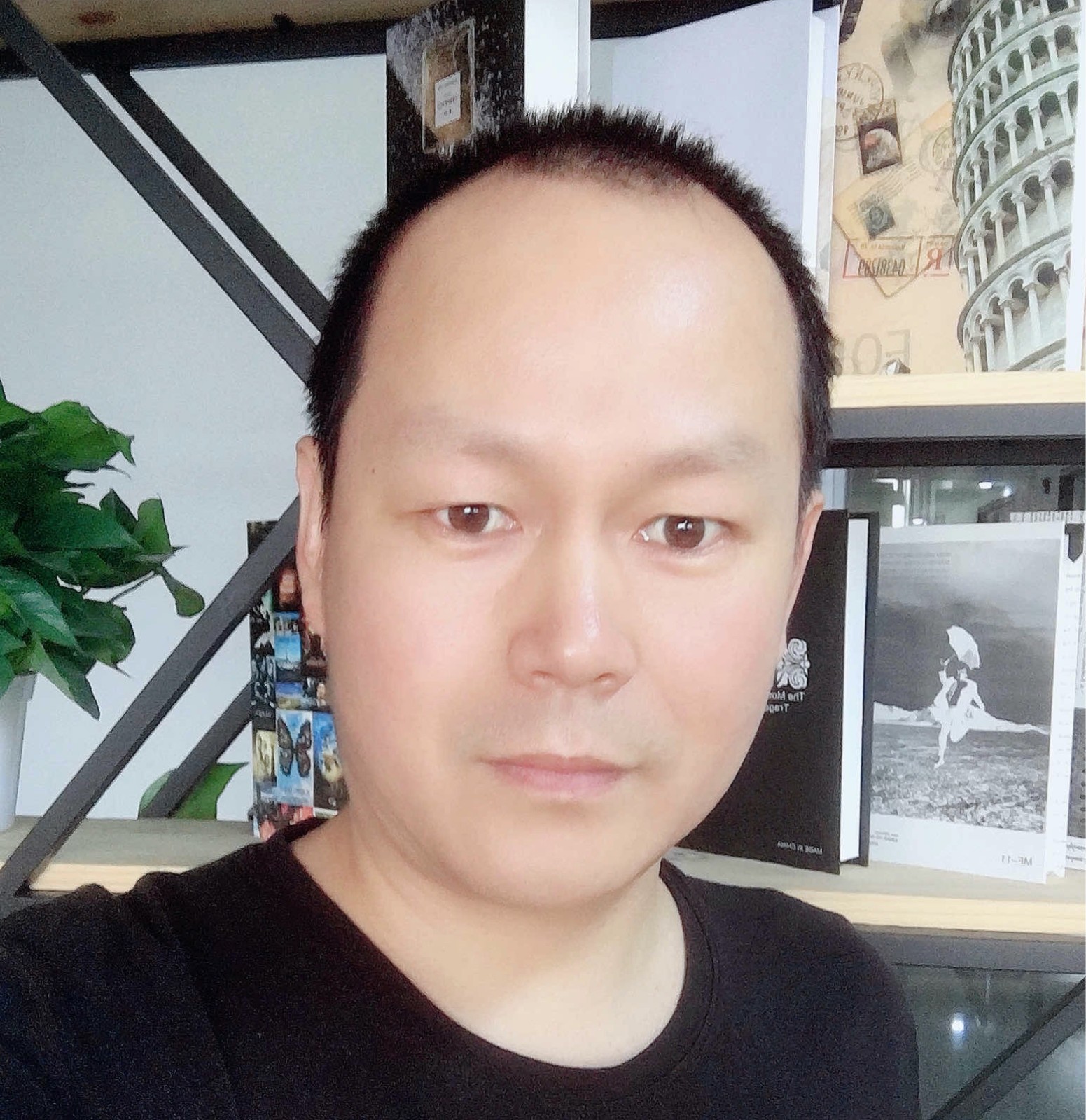
Correction status:qualified
Teacher's comments:
作业内容:
//定义常量,必须要初始化
const URL_HOST = "www.gzj2001.com";
console.log(URL_HOST);
//定义变量,可以不初始化,变量可以重写
let blogName;
console.log(blogName);
console.log("-------");
blogName = "城南花开";
console.log(blogName);
//函数可以被重写
function getInformation() {
return "美好的事情即将发生~";
}
console.log(getInformation());
function getInformation() {
return "现在美好的事情已经发生了~";
}
//匿名函数
let good = function () {
return "你改变不了我的内容";
}
console.log(good);
console.log(good());
console.log("---------");
//使用箭头函数
let sum = function (a, b) {
return a + b;
};
// 箭头函数用来简化 "匿名函数" 的声明
sum = (a, b) => {
return a + b;
};
//箭头函数的参数特征
//如果函数只有一条语句,可不写return
let list = (a, b) => a + b;
console.log(list(1, 2));
//如果只有一个参数,小括号可以省略
let list2 = a => a + "小括号能省略";
console.log(list2("list2"));
//如果没有参数,小括号不能省略
let list3 = () => "小括号不能省略";
console.log(list3());
//闭包
function getAllUser() {
let userList = {
id: 1,
name: "admin",
email: "admin@qq.com",
}
return function () {
return userList;
}
}
console.log("--------");
console.log(getAllUser());
console.table(getAllUser()());
//高阶函数
// 1.回调函数:写在参数内的函数
document.addEventListener("click", function () {
alert("Hello World~~");
});
// 2.偏函数: 简化了声明时的参数声明
let sum2 = function (a, b) {
return function (c, d) {
return a + b + c + d;
};
};
//可以先储存固定需要传入的一部分参数,然后一起传入
let f1 = sum2(2, 3);
console.log(f1(3, 4));
// 3.柯里化:简化了调用参数
sum3 = function (a) {
return function (b) {
return function (c) {
return function (d) {
return a + b + c + d;
};
};
};
};
//一层一层的传入参数
let res = sum3(10)(20)(30)(40);
console.log(res);
// 4.纯函数:完全独立于调用上下文,返回值只能受到传入的参数影响
function addSum(a,b){
return a + b;
}
console.log(addSum(1,8));
//理解回调和纯函数是什么。
// 回调函数是沟通两个不同功能函数的中间件,类似以前的电话接线员。
// 纯函数是自己写的功能函数,返回的内容受到传入参数的影响而变化的。
function testA(a,b){
console.log("a is" + a);
console.log("b is" + b);
// console.log(testB(a,b));
testB(a,b);
}
function testB(c,d){
console.log(c + d);
}
testA(11,52);