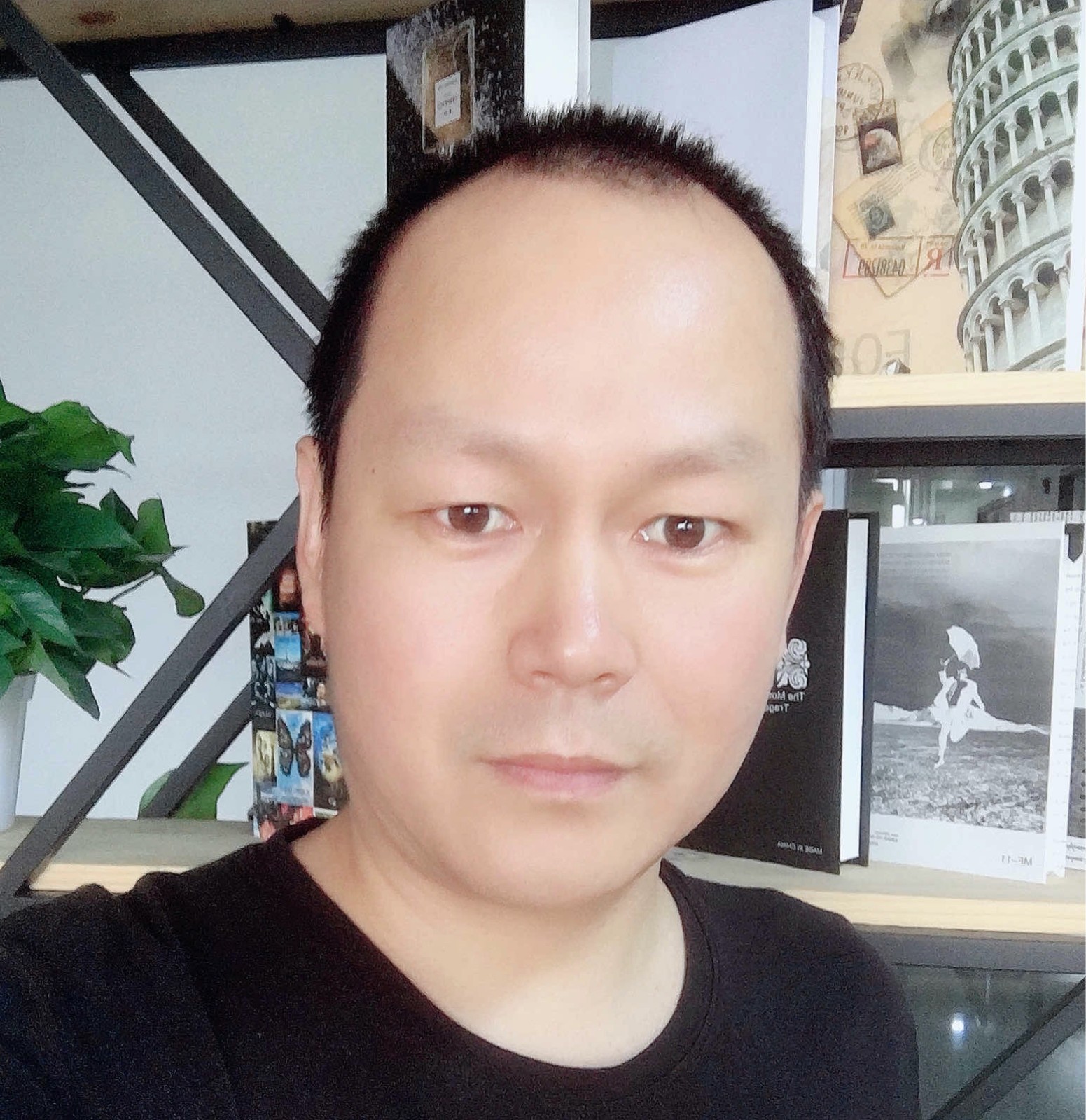
Correction status:qualified
Teacher's comments:
<script>
//以数组为例
const colors = ['red','green','blue'];
//初始变量,循环条件,条件更新
let i = 0 ;
while(i<colors.length){
cobsole.log("%c%s","color:red",colors[i]);
i++;
}
//出口判断
i = 0;
do {
cobsole.log("%c%s","color:red",colors[i]);
i++;
}while(i<colors.length);
//for 循环
for(let i = 0;i<colors.length;i++){
cobsole.log("%c%s","color:red",colors[i]);
}
//对象使用for-in
const lesson = {name:'js',score:80};
for (let key in lesson){
cobsole.log("%c%s","color:red",colors[key]);
}
// for - of
for (let item of colors){
cobsole.log("%c%s","color:red",item);
}
</script>
<script>
// 手写迭代器
function myIterator(data){
//迭代器中必须要有一个-next()
let i = 0;
return {
next(){
//done:表示遍历是否完成
// value:当前遍历的数据
let done = i >= data.length ? false : true
let value = !done ? data[i++]:undefined;
return {done,value}
}
}
}
let iterator = myIterator(['a','b','c']);
console.log(iterator.next());
</script>
— 任何一个函数都有一个原型属性:portotype
<script>
function f1(){}
console.dir(f1);
//声明一个构造函数
function User(name,email){
//创建出一个新对象,用this来表示(伪d代码,系统自动创建并执行
const this = new User;、
//初始化对象,给对象添加自定义属性,用来和其他实列进行区分
this.name = name;
this.email = email;
//返回这个对象
//return this;
}
const user = new User('admin','admin@php.cn');
console.log(user);
user.__proto__.salary = 8899;
console.log(user.salary);
const user1 = new User('admin1','admin1@php.cn');
console.log(user1);
//实列的原型永远指向它的构造函数的原型,实例的原型从构造函数的原型继承成员(属性/方法)
console.log(user1.__proto__ === User.prototype);
//需要被所有实列共享的成员,应该写道构造函数的原型上
User.prototype.nation = "CHINA";
console.log(user.nation,user1.nation);
//属性不应该共享,它是区分不同对象的标志,方法更适合共享
User.prototype.show = function(){
return {name:this.name,email:this.emali,salary:this.salary}
}
console.log(user.show());
console.log(user1.show());
</script>
构造函数模拟类
-constructor 构造方法
-静态方法:static 不需要通过对象调用,直接用类调用
-静态方法中的this指向类
-私有成员,只能在奔本类中使用,类外,子类中不能用 #,静态方法不能访问,声明为私有主要是为访问限制
类的继承
-扩展父类的功能,可以拥有自己的属性或方法
-子类中可以访问父类的构造方法,原型方法,静态方法,不能访问私有的
-使用super()调用父类构造方法,确定this指向
const User = function(name,email){
this.name = name;
this.email = email;
}
//原型方法
User.prototype.show = function(){
return {name:this.name,email:this.email};
}
const user = new User("世界","你好@qq.com")
console.log(user.show);
// es6中的类,来改写上面
class User1{
//构造方法
constructor(name,email){
this.name = name;
this.email = email;
}
show(){
return {name:this.name,email:this.email,agethis.age};
}
//静态方法:不需要通过对象调用,直接用类调用
static fetch(){
//return {name:this.name,email:this.email};
return this.hello(this.userName);
}
static userName = '你好';
static hello(name){
return 'hello' + name;
}
#age = 40;
//访问器属性
set age(value){
if(value>=18 && value<=80){
this.#age = value;
}else{
throw new Error('年龄必须在18-60之间')
}
}
get age(){
return this.#agep;
}
}
const user1 = new User1("世界","你好@qq.com")
console.log(user1.show);
console.log(Array.of(1,2,3));
user.age = 53;
console.log(user1.age);
// 类的继承
class Child extends User1{
constructor(name,email,la){
super(name,email);
this.la = la;
}
show(){
return {name:this.name,email:this.email,la:this.la}
}
}
const child = new Child('世界','shijie@11.con','1亿');
console.log(child);
<ul id="lilst">
<li class="item"></li>
<li class="item"></li>
<li class="item"></li>
<li class="item"></li>
<li class="item"></li>
<ul>
<script>
//使用css选择器是最直观的
//获取满足条件的所有元素
const lis = document.querySelectorAll('#list > *);
console.log(lis);
//Nodelist 是浏览器内置的集合类型,属性类数组
let lisArr = Array.from(lis);
console.log(lisArr);
// ...
let larr = [...lis];
console.,log(larr);
//Nodelist可以直接用forEach()遍历
lis.forEach(function(item,index,arr){
console.log(item,index,arr);
})
//使用箭头函数
lis.forEach((item)=>console.log(item));
// 只获取一个,但返回的仍然是一个集合
let first = document.querySelecouAll('#list 里:first-of-type');
console.log(first[0]);
//获取满足条件的第一个元素
first = document.querySelector('#list l;i');
</script>
创建元素
-createElement();
-parent appendChild(newEl),添加到页面
-将html逐个添加 字符串直接解析dom元素 insertAdjacentHTML(beforeEnd(到最后))
insertAdjacentHTML(afterBegin(到前面))
-如果大量添加元素应该使用文档片段完成
更新
-replaceWith()
-replaceChild();
移除
-removeChild()
-遍历查询
-获取所有的子元素 children
<ul id="lsit">
<li></li>
<li></li>
<li></li>
<li></li>
</ul>
<script>
const ul = document.querySelector('#list');
//创建元素
const li = document.createElement('li');
//parent appendChild(newEl),添加到页面
ul.appendChild(li);
li.innerText = 'item6';
//将html逐个添加 字符串直接解析dom元素 insertAdjacentHTML()
let htmlStr = "<li style='color:red'>item7</li>";
ul.insertAdjacentHTML('beforeEnd',htmlStr);
//如果大量添加元素应该使用文档片段完成
const frag = doucument.createDocumentFrament();
const frag = new DocumentFragment():
for(let i=0;i<5;i++){
const li = document.creatElement('li');
li.textContent = 'Hello' + (i+1);
frag.appendChild(li);
}
ul.appendChild(frag);
htmlStr = `
<li style="color:violet">牛号<li>
`;
//用元素不用字符串
ul.insertAdjacentElement("afterBegin",htmlStr)
//更新
let h3 = document.createElememt("h3");
h3.innerHTML = '晚上好';
document.querySelector('li:nth-of-type(3)').replaceWith(h3);
document.querySelector('li:last-of-type').replaceChild(h3);
// 移除
ul.removeChild(document.querySelector('#list h3'));
//遍历查询
//获取所有子元素
console.log(ul.children);
//获取子元素的数量
console.log(ul.children.length)
console.log(ul.childElementCount)
// 第一个子元素
console.log(ul.firstElementChild);
//最后一个
console.log(ul.lastElementChild);
//父节点
console.log(ul.lastElementChild.parentElement);
//前一个兄弟节点
const jiu = document.querySelector('#list li:net-of-type(9)');
jiu.style.background = 'yellow';
console.log(jiu.previousElementSibling.innerHTML);
//后一个兄弟
console.log(jiu.nextElementSibling.innerHTML);
</script>