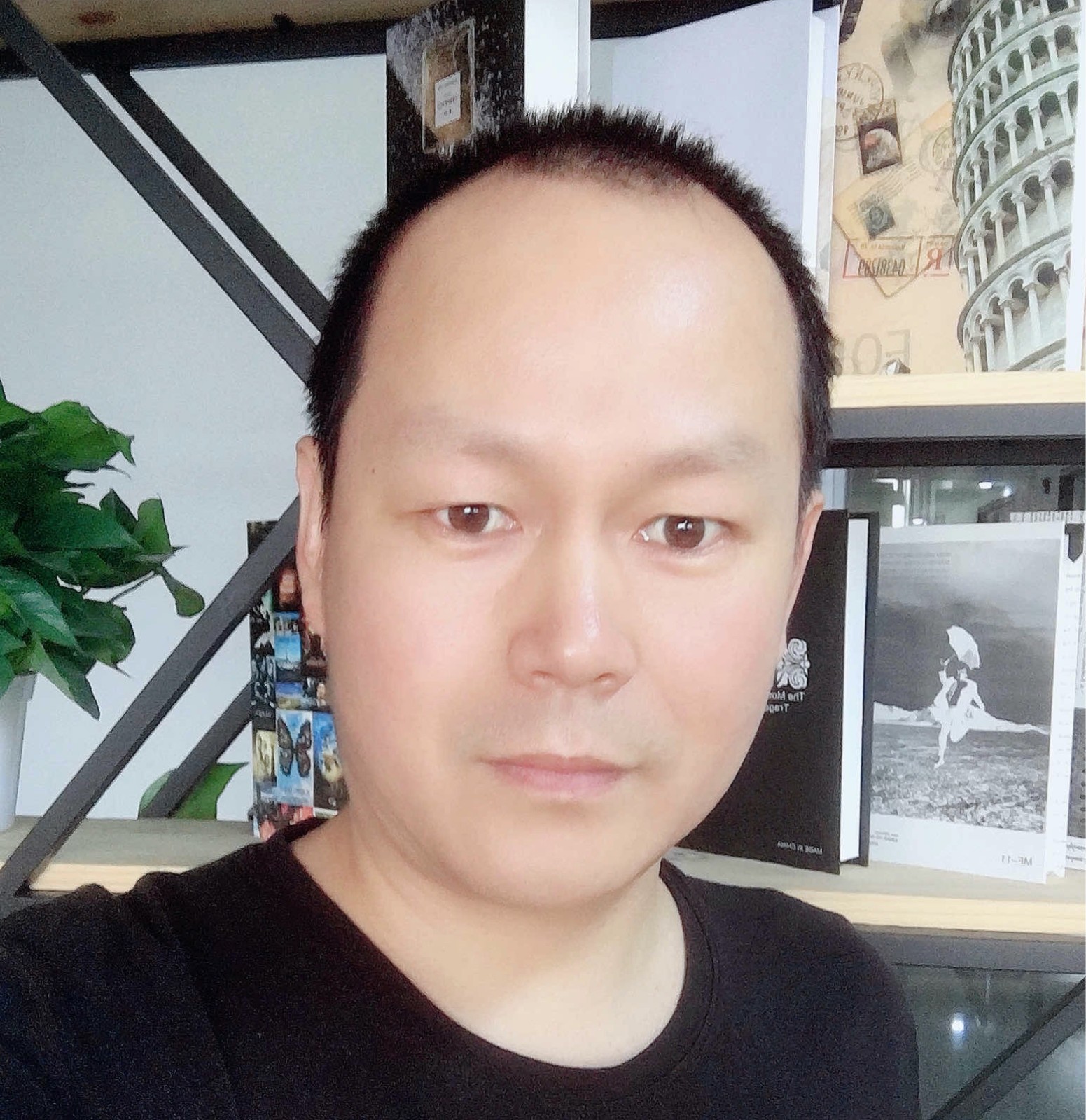
Correction status:qualified
Teacher's comments:
事件监听调用addEventListener属性。此监听可以添加多个事件,且不会被覆盖
删除事件监听调用removeEventListener属性。
<button>按钮</button>
<script>
const btn=document.querySelector("button");
const handle=()=>console.log(btn.innerHTML,"移除");
btn.addEventListener("click",handle);
btn.removeEventListener("click",handle);
</script>
不用调用,直接就执行了。
const ev=new Event("click");
btn.addEventListener("click",function(){
console.log("自动执行了");
});
btn.dispatchEvent(ev);
两种方式捕获,冒泡
捕获,从最外层元素逐级向内直到事件的绑定者 事件为true;
2.冒泡:从目标再由内向外逐级向上直到最外层元素 事件为false;
<style>
#fu{
background-color: yellow;
width: 200px;
height: 200px;
}
#zi{
background-color: green;
width: 50px;
height: 50px;
}
</style>
<body>
<div id="fu">
<div id="zi">
</div>
</div>
<script>
const fu=document.querySelector("#fu");
const zi=document.querySelector("#zi");
fu.addEventListener("click",function(ev){
console.log(ev.currentTarget);
},true);
zi.addEventListener("click",function(ev){
console.log(ev.currentTarget);
},true);
</script>
</body>
如果元素太多,给父元素添加事件即可
<ul>
<li>item1</li>
<li>item2</li>
<li>item3</li>
</ul>
<script>
const ul=document.querySelector("ul");
ul.addEventListener("click",function(ev){
ev.target.style.color="red";
});
</script>
留言板点击留言删除
<label for=""><input type="text" name="message"></label>
<ol id="list"></ol>
<script>
const msg=document.querySelector("input");
const list=document.querySelector("#list");
msg.onkeydown=ev=>{
//键盘事件中,key表示按下的键名
console.log(ev.key);
if(ev.key==="Enter"){
if(ev.currentTarget.value.length===0){
alert("内容不能为空");
}else{
let str=`<li>${ev.currentTarget.value}</li>`;
list.insertAdjacentHTML("afterbegin",str);
//清空上条数据
ev.currentTarget.value=null;
}
}
}
//点击某条留言则删除
list.addEventListener("click",function(ev){
list.removeChild(ev.target);
});
</script>
//slice(start,end)取子串
let str="hello php.cn";
let res=str.slice(0,5);
//截取
substr(start,length)
res=str.substr(0,5);
//trim()删除两边空格
let psw=" root ";
psw.trim();
//字符串长度
str.length;
//查找字符串 某一个指定的字符首次出现的位置
str.indexOf("hello");
//替换内容replace("被替换","替换后的内容");
str.replace("hello","morning");
//大小写转换
str.toUpperCase();//转为大写
str.toLowerCase();//转为小写
//转为数组
str.split(" ");使用空格分隔
//内容匹配,查找字符串中特定的字符,找到则返回这个字符
str.match("hello");
//复制字符串repeat(次数)
str.repeat(2);