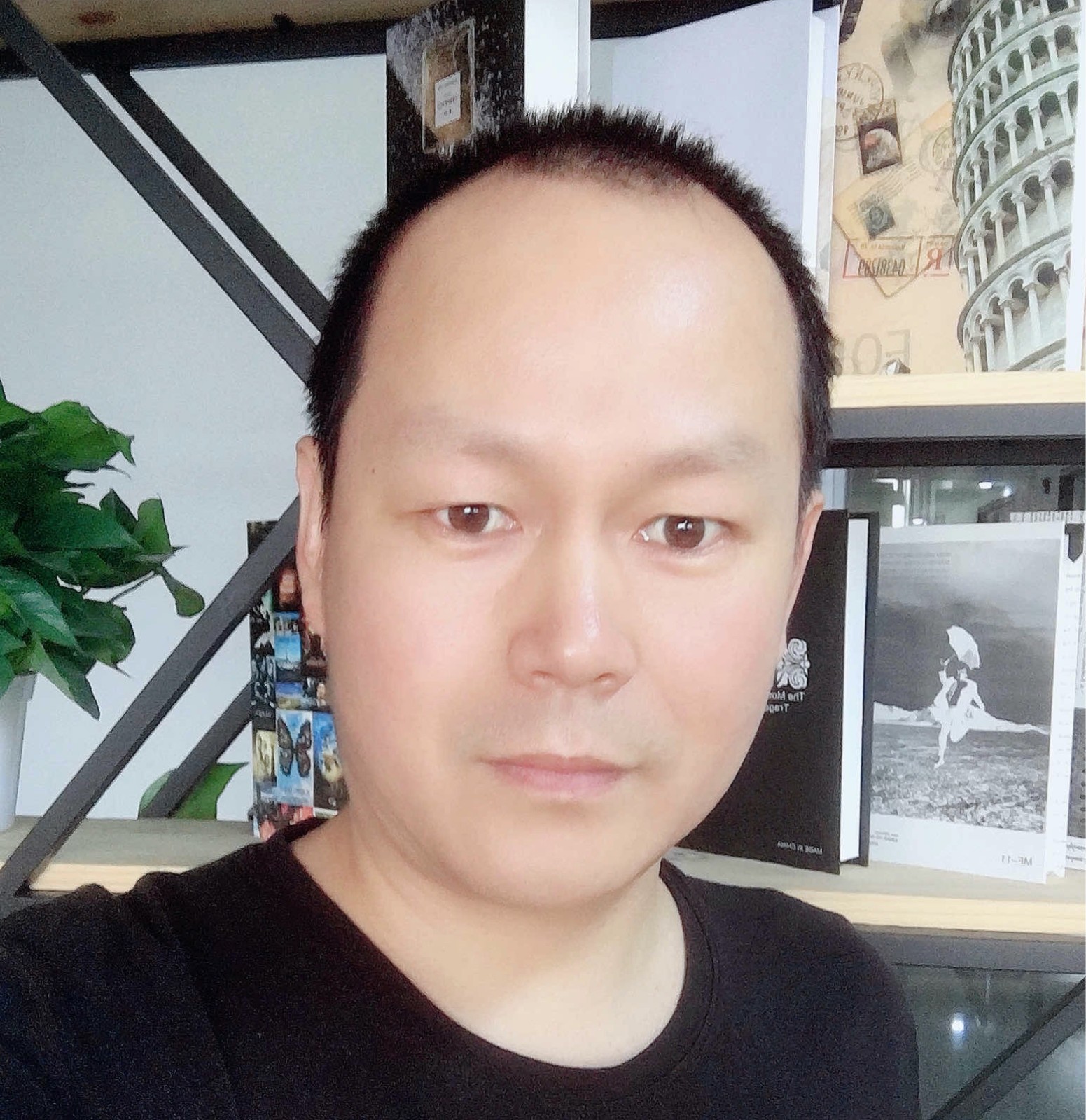
Correction status:qualified
Teacher's comments:
实例演示条件渲染, 计算属性和侦听器,并比较计算属性与侦听器之间的区别
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>条件渲染:v-if,v-show</title>
</head>
<body>
<div id="app">
<!-- 单分支 -->
<p v-if="flag">{{msg}}</p>
<button
@click="flag = !flag"
v-text="tips = flag ? `隐藏` : `显示`"
></button>
<!-- 多分支 -->
<p v-if="point > 0 && point < 60">{{grade[0]}}</p>
<p v-else-if="point >= 60 && point < 70">{{grade[1]}}</p>
<p v-else-if="point >= 80 && point < 90">{{grade[2]}}</p>
<p v-else-if="point >= 90 && point < 110">{{grade[3]}}</p>
<p v-else>{{grade[4]}}</p>
<!-- v-show: -->
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
// v-if: 元素是否添加到页面中
// v-show: 元素是否显示出来(元素已经在dom结构中)
const vm = new Vue({
el: document.querySelector("#app"),
data() {
return {
msg: "核心幕僚:拜登明白只有一个中国,首都在北京",
flag: true,
tips: "隐藏",
point: 120,
grade: [
"不及格!",
"合格",
"良好",
"优秀",
"超级学霸!",
],
status: true,
};
},
});
</script>
</body>
</html>
图示:
总结:
v-if的渲染方式:
会确保在切换过程中条件块内的事件监听器和子组件适当地被销毁和重建;
只有当条件第一次变为真时,才会开始渲染条件块;
切换开销比v-show高;
v-show的渲染方式:
不管初始条件是什么,元素总是会被渲染;
只是简单地基于 CSS 进行切换;
v-show的初始渲染开销比v-if高;
如果需要频繁的切换,则使用v-show;
如果运行时条件很少改变,则用v-if
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>计算属性</title>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
</head>
<body>
<div class="app">
<p>数量:<input type="number" v-model="num" style="width: 5em" min="0" /></p>
<p>单价: {{price}} 元</p>
<p>金额: {{amount}} 元</p>
</div>
<script>
const vm = new Vue({
el: document.querySelector(".app"),
data() {
return {
num: 0,
price: 80,
res: 0,
};
},
computed: {
amount: {
get() {
return this.num * this.price;
},
set(value) {
console.log(value);
if (value > 1000) this.price = 30;
},
},
},
});
vm.amount = 1001;
</script>
</body>
</html>
图示:
三、侦听器
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>侦听器</title>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
</head>
<body>
<div class="app">
<p>数量:<input type="number" v-model="num" style="width: 5em" min="0" :max="max"/></p>
<p>单价: {{price}} 元</p>
<p>金额: {{res}} 元</p>
</div>
<script>
const vm = new Vue({
el: document.querySelector(".app"),
data() {
return {
num: 0,
price: 50,
res: 0,
max: 100,
};
},
// 侦听器属性
watch: {
// 侦听的是某一个属性的值的变化,它的属性名与data中要监听的属性同名
num(newValut, oldValue) {
console.log(newValut, oldValue);
// this.res = this.num * this.price;
this.res = newValut * this.price;
// 监听库存
if (newValut >= 20) {
this.max = newValut;
alert("库存不足,请补货");
}
},
},
});
</script>
</body>
</html>
图示:
计算属性和侦听器区别:
watch:监测的是属性值, 只要属性值发生变化,其都会触发执行回调函数来执行一系列操作;
computed:监测的是依赖值,依赖值不变的情况下其会直接读取缓存进行复用,变化的情况下才会重新计算。
除此之外,有点很重要的区别是:计算属性不能执行异步任务,计算属性必须同步执行。也就是说计算属性不能向服务器请求或者执行异步任务。如果遇到异步任务,就交给侦听属性。Watch也可以检测computed属性。