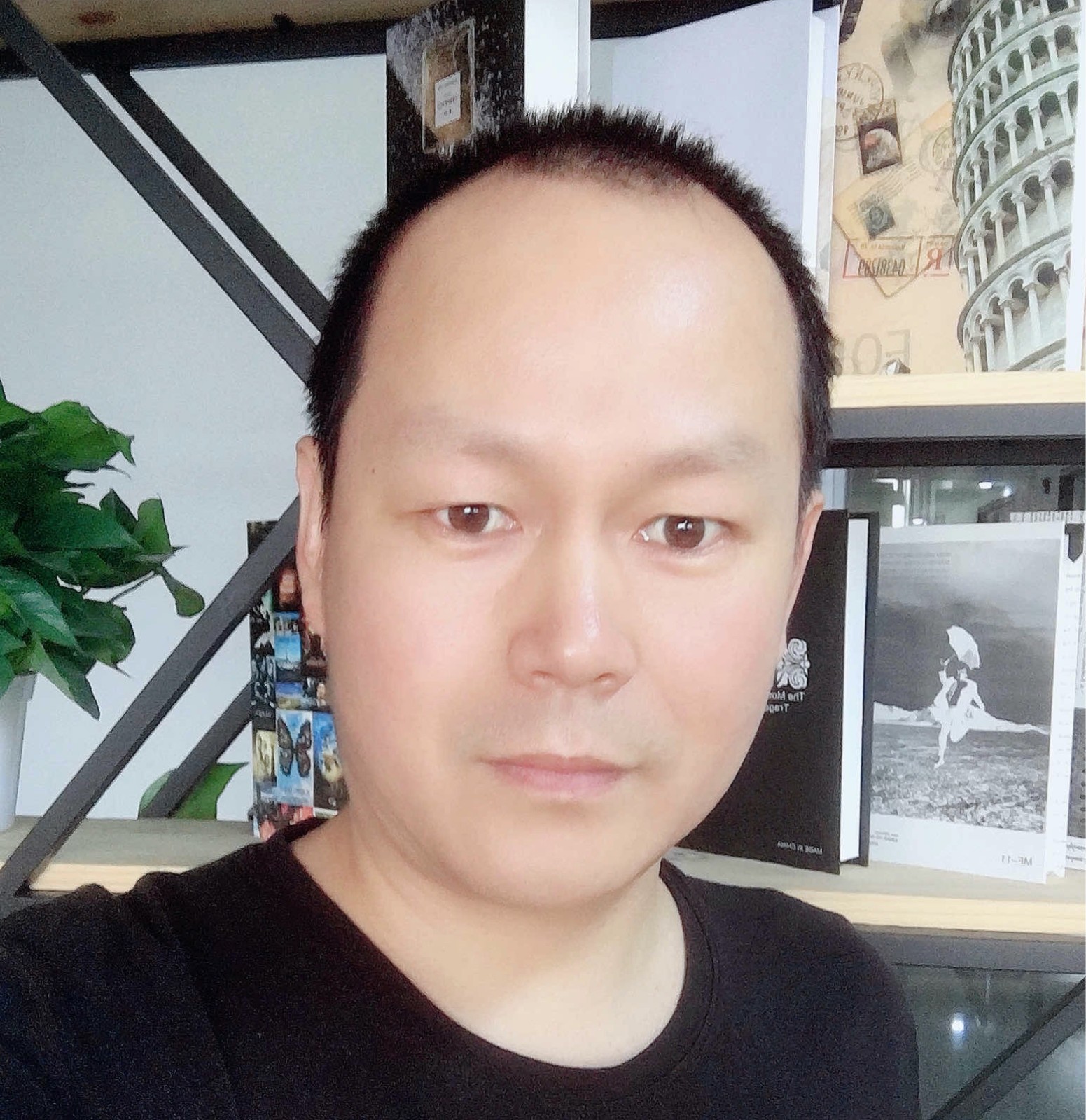
Correction status:qualified
Teacher's comments:
数组的常用方法如下代码所示
<script>
//栈方法,后进先出(在数组尾部进行的)push() pop();队:先进新出(在数组头部进行的)unshift() shift()
let arr = [];
// 在数组尾部进行操作添加数组
// console.log(arr.push(1, 2, 3, 4));
// 删除数组
// console.log(arr.pop());
// console.log(arr.pop());
// console.log(arr.pop());
// console.log(arr.pop());
console.log("%s", "-------------", arr);
// 在数组头部进行的操作,unshift() shift()添加和删除操作;先进先出,
console.log(arr.unshift(1, 2, 3, 4));
console.log(arr.shift());
console.log(arr);
// 可以push()和shift()结合使用,也可以unshift()和pop()结合使用、
// join()与字符串处理的split()相反,是将数组转化成字符串,并返回
console.log(arr.join("-"));
// concat()字符串组合函数
// slice()返回数组中的部分成员,返回的数组是一个新的数组
console.log(arr.slice(1));
// splice(开始索引,删除数量,插入数据);数组的增删改,它的本质是删除数组内容
let se = [1, 2, 34, 4, 5];
// 删除元素
console.log(se.splice(0, 2));
console.log(se);
// 更新
se.splice(0, 2, 88, 99);
console.log(se);
// 新增
se.splice(0, 0, 3, 4, 6);
console.log(se);
// 排序
// 默认按照自摸排序,sort();
let res = ["b", "a", "c", "f", "e"];
console.log(res.sort());
// 如果想要阿拉伯数字排正序需要参数a-b,降序相反
console.log(se.sort((a, b) => a - b));
// 遍历
arr.forEach((item) => console.log(item));
// map对数组的每个成员都调用回调进行处理并返回这个数组
let mp = se.map((item) => item * 2);
console.log(mp);
// 过滤
let gl = se.filter((item) => item > 10);
console.log(gl);
// 归内,求总数;reduce(callback(prev,curr));
let sum = se.reduce((prev, curr) => prev + curr, 100);
console.log(sum);
</script>
执行结果如下:
JSON常用的方法有JSON.stringify()(将JS数据转换成JSON数据),JSON 数据都是字符串,还有一个方法是JSON.parse()(将JSON数据装换成JS数据);
用法如下代码所示:
<script>
//JSON.stringify(data,replacer,space);将Js数据转为json
console.log(JSON.stringify(3.14), typeof JSON.stringify(3.14));
console.log(
JSON.stringify({ a: "a", b: "b" }),
typeof JSON.stringify({ a: "a", b: "b" })
);
//对json格式的字符串的特殊操作,主要通过后边连个参数,第二个参数自持数组和函数
// 数组
// 把属性a和属性b过滤出来
// 第三个参数是用来格式化json输出字符窜的样式
let str = JSON.stringify({ a: 1, b: 2, c: 3, d: 4, e: 5 });
console.log(JSON.stringify({ a: 1, b: 2, c: 3, d: 4, e: 5 }, ["a", "b"]));
// 函数
console.log(
JSON.stringify(
{ a: 1, b: 2, c: 3, d: 4, e: 5 },
(k, v) => {
if (v > 3) return undefined;
return v;
},
"****"
)
);
console.log(str);
// 把json数据转换成JS JSON.parse()
console.log(JSON.parse(str), typeof JSON.parse(str));
console.log(
JSON.parse(str, (k, v) => {
if (v === "") return v;
return v * 2;
})
);
</script>
执行结果:
ajax异步提交主要有四个步骤:
1.创建xhr对象const xhr=new XMLHttpRequest();
;
2.配置xhr参数xhr.open('get','url地址');``xhr.responseType='json';
;
3.处理xhr响应xhr.onload=()=>{};
;
4.发送xhr请求,get方式时参数为null;xhr.send(null);
具体代码如下:
<script>
const Btn = document.querySelector("button");
Btn.addEventListener("click", (ev) => {
//xhr 异步提交的步骤分为四步
// 1创建xhr对象
const xhr = new XMLHttpRequest();
// 2.配置xhr参数
xhr.open("get", "../php/test1.php?id=2");
// 这一步可以省略因为返回的数据都是json数据
xhr.responseType = "json";
// 3.处理xhr相应
// 成功
xhr.onload = () => {
console.log(xhr.response);
// DOM将响应的结果渲染到页面中去
let user = `${xhr.response.name}(${xhr.response.email})`;
document.querySelector("p").innerHTML = user;
};
// 失败时候
xhr.onerror = () => console.log("Error");
// 4.发送xhr请求 get请求时参数为空
xhr.send(null);
});
<button>ajax-get</button>
<p></p>
执行结果: