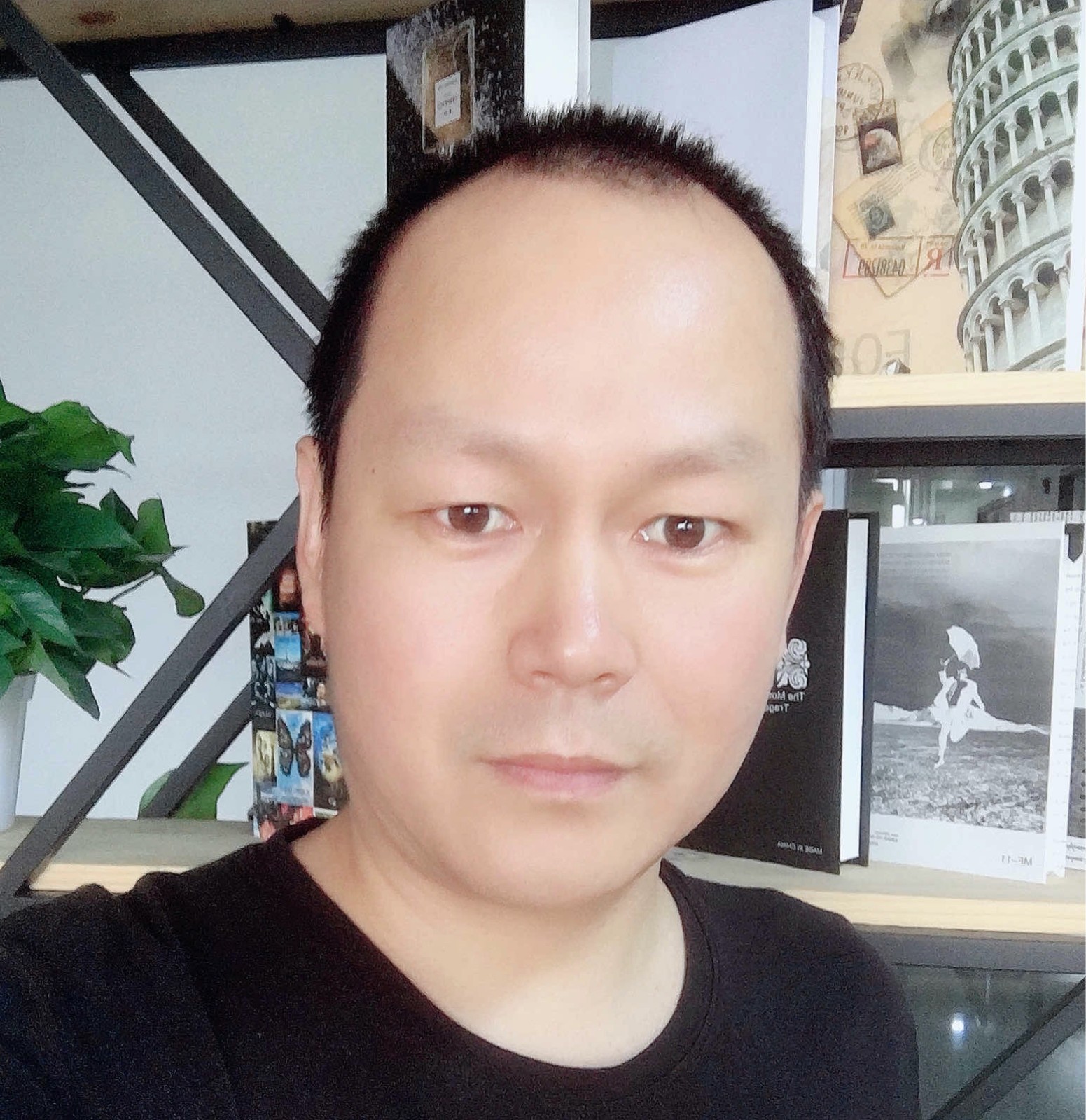
Correction status:qualified
Teacher's comments:
<?php
//声明一个类文件,使用关键字class 后面跟类名,建议首字母大写
class Customer
{
private $name;
private $phone;
private $adress;
//构造方法(系统自动调用的,作用是将属性实例化)
function __construct($name, $phone, $adress)
{
$this->name = $name;
$this->phone = $phone;
$this->adress = $adress;
}
function show()
{
return "{$this->name}的送货地址为{$this->adress},电话号码为{$this->phone}";
}
}
$zhangsan = new Customer('张三',136123456,'石家庄');
echo $zhangsan->show();
<?php
//声明一个类文件,使用关键字class
class Customer
{
static $name;
static $phone;
static $adress;
//利用构造方法给静态函数传参
public function __construct($name,$phone,$adress)
{
//在类内部调用类的静态成员要用self
self::$name = $name;
self::$phone = $phone;
self::$adress = $adress;
}
static function show(){
return'顾客'.self::$name .'的地址为'.self::$adress.',电话号码为'.self::$phone;
}
}
$user = new Customer('张三','123456','石家庄');
//在类的外部访问静态成员,需要使用类名的方式访问
echo Customer::show();
<?php
//声明一个类文件,使用关键字class 后面跟类名,建议首字母大写
class Customer
{
protected $name;
protected $phone;
//构造方法(系统自动调用的,作用是将属性实例化)
function __construct($name, $phone)
{
$this->name = $name;
$this->phone = $phone;
}
function show()
{
return "{$this->name}的电话号码为{$this->phone}";
}
}
//声明一个子类
class SonCustomer extends Customer
{
private $adress;
//类的功能扩展1:重写父类的方法 __construct()(构造器)
function __construct($name, $phone, $adress)
{
parent::__construct($name, $phone);
$this->adress = $adress;
}
//类的功能扩展1:重写父类的方法show()
function show()
{
return parent::show().',送货地址为'.$this->adress;
}
//类的功能扩展2:添加新功能
function adress(){
return '送货地址为'.$this->adress;
}
}
//子类实例化
$lisi = new SonCustomer('李四','456789','北京');
echo $lisi->show();
echo $lisi->adress();
类似于函数库(公共方法集),功能是代码复用。借用了类的方法,但是不能实例化。
在需要引用trait的类中,用关键字use引用它即可。
当trait中的成员名与父类、子类重名时
优先级 子类>trait>父级
<?php
class Mm{
function name(){
return '这里是父类的name方法';
}
}
trait A1{
function name(){
return '这里是trait A1的name方法';
}
}
class Xingming extends Mm
{
use A1;
}
// 输出 这里是trait A1的name方法
echo (new Xingming)->name();
class Xingming2
{
use A1;
function name(){
return '这里是子类的name方法';
}
}
// 输出 这里是trait A1的name方法
echo (new Xingming2)->name();
当多个trait成员引用时,发生命名冲突,解决方法:使用优先级+别名
<?php
trait A1{
function name(){
return '这里是trait A1的name方法';
}
}
trait A2{
function name(){
return '这里是trait A2的name方法';
}
}
class Xingming{
use A1,A2{
//给A1的name()方法设置优先级
A1::name insteadOf A2;
//给A2的name()方法名设置别名
A2::name as a2name;
}
}
// 输出 这里是trait A1的name方法
echo (new Xingming)->name();
// 输出 这里是trait A2的name方法
echo (new Xingming)->a2name();