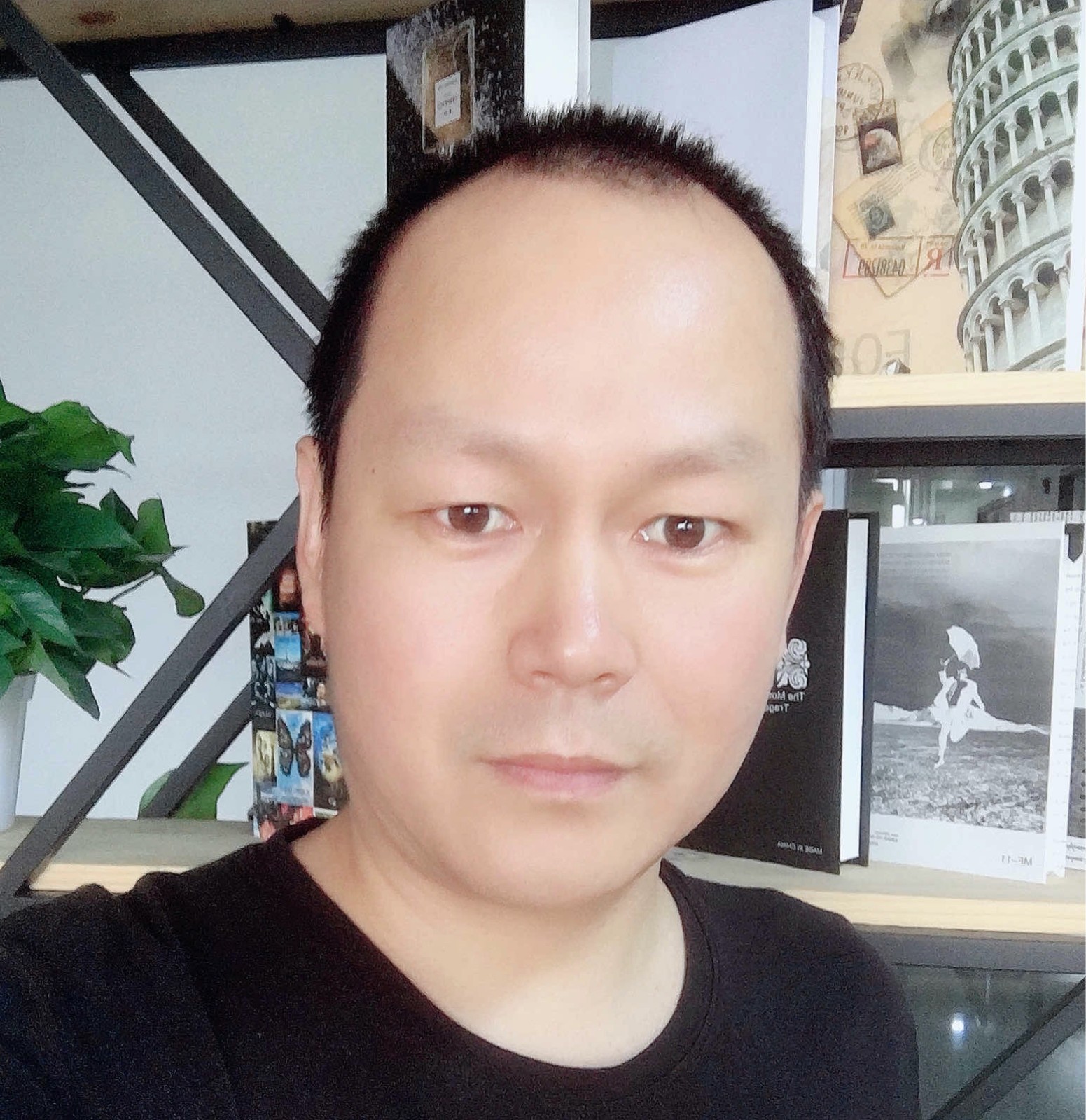
Correction status:qualified
Teacher's comments:
<script>
//while循环,入口判断
const colors=["red","grenn","blue"]
let i=0;
//length是数组的长度
while(i<colors.length){
console.log("%c%s", "color:red",colors[i]);
//更新变量
i++
}
//for循环
for(i=0;i<colors.length;i++){
console.log("%c%s", "color:blue", colors[i]);
}
//以上对象不能用,对象用for-in
const less={name:"js",score:80};
for(let key in less){
console.log(less[key]);
}
//其中的key可以是变量名,也可以遍历数组
for(let key in colors){
console.log(coloes[key]);
}
//for-of:遍历的终结者,都可以用这个来遍历
for (let item of colors){
console.log(irem);
}
//但是在遍历自定义对象的时候回报错
for(let item of less){
console.log(item);
}
//因为自定义对象没有代送器接口
</script>
<script>
//声明构造函数,一般是首字母大写,里面的可以传参数
function User(name,email){
this.name=name;
this.email=email;
}
//声明对象,给构造函数里面的参数传参
const user=new User("马","ma@qq.com");
console.log(user);
//给user的父级原型里面加点东西
user._proto_.salary=8899;
//访问,它这个访问会先出自由属性上找,找不到会去原型里面找,这叫原型链
console.log(user.salary);
//再声明一个对象,给构造函数的参数传参
const user1=new User("张","za@qq.com");
//访问这个对象的salary属性
console.log(user1.salary);
//访问发现user._proto_的salary共享给了user1
//让对象的_proto_和构造函数的prototype后返回true,发现完全相等
console.log(user1.__proto__ === User.prototype);
//可以通过prototype添加值和属性来共享给下面的子对象
//构造函数的自由属性比原型链权限高,会覆盖
</script>
<script>
//先声明一个类
const User=function(name,email){
this.name=name;
this.email=email;
};
//然后给这个类的原型链上加上匿名函数
User.prototype.show=function(){
return{name:this.name,email:this.email};
};
//然后声明变量赋值
const user=new User("小马","ma@qq.com");
//然后调用原型链上的show方法
console.log(user.show());
//es6中改写方法
class User1{
constructor(name,email){
this.name=name;
this.email=email;
}
show(){
return{name:this.name,email:this.email}
}
}
const user1=new User1("小马","ma@qq.com");
console.log(user1.show());
//静态方法
class User2{
//static是关键字,后面加上静态方法
static fetch(){
return this.hello(this.userName);
}
//静态属性
static userName="小王";
//静态方法
static hello(name){
return"hello"+name;
}
}
console.log(User2.fetch());
//私有变量,只能在本类中使用,类外,子类中不能用,重名没关系,外面比里面的大,会覆盖,不知道为啥火狐不支持私有变量语法
class User3{
//前面加个#就是私有变量
#age=40;
get age(){
return this.#age;
}
set age(value){
if(value>=18 && value<=60){
this.#age=value;
}elsr{
throw new Error("必须在18-60之间")
}
}
}
console.log(User3.get);
console.log(User3.age);
//类的继承,叫子类
//子类继承类的一切,处了私有的
//用extends来关联两者
class Child extends User1{
constructor(name,email,gender){
//如果要调用父级的变量,必须用super俩调用,要是直接用回报错
super(name,email);
this.gender=gender;
}
show() {
return { name: this.name,
email: this.email,
gender: this.gender
};
}
}
const child = new Child("老师", "a@qq.con", "男");
console.log(child.show());
</script>
<body>
<ul id="list">
<li class="item">item1</li>
<li class="item">item2</li>
<li class="item">item3</li>
<li class="item">item4</li>
<li class="item">item5</li>
</ul>
<form action="">
<input type="text" />
</form>
<script>
//querSelectorALL获取满足所以的条件的元素
const lis = document.querSelectorALL("#list li");
console.log(lis);
//用forEach()来遍历获取到的值
lis.forEach((item)=>console.log(item));
//获取第一个,直接用伪类选择器来选中
let first=document.querySelectorALL("#list li:first-of-type");
console.log(first[0]);
//可以通过用document.querySelector来直接选择第一个
first=document.querySelector("#list li")
console.log(first);
</script>
</body>
<body>
<ul id="list">
<li>item1</li>
<li>item2</li>
<li>item3</li>
<li>item4</li>
<li>item5</li>
</ul>
<script>
//增加
const ul=document.querySelector("#list");
const li=document.createElement("li");
ul.appendChild(li);
li.innerText="新添加的";
//li.innerHTML = '<li style="color:red">红色</li>';
</script>
</body>
<body>
<ul id="list">
<li>item1</li>
<li>item2</li>
<li>item3</li>
<li>item4</li>
<li>item5</li>
</ul>
<script>
const ul = document.querySelector("#list");
//批量增加
const Frag=new DocumentFragment();
for(let i=0;i<4;i++){
const li=document.createElement("li");
li.innerText="批量添加";
Frag.appendChild(li);
}
ul.appendChild(Frag);
</script>
</body>
<body>
<ul id="list">
<li>item1</li>
<li>item2</li>
<li>item3</li>
<li>item4</li>
<li>item5</li>
</ul>
<script>
const ul = document.querySelector("#list");
//更新插入一个值
let h3=document.createElement("h3");
h3.innerText="晚上好";
//先把h3代替第三位
document.querySelector("li:nth-of-type(3)").replaceWith(h3);
//然后把h3代替到第一位
ul.replaceChild(h3, document.querySelector("li:nth-of-type(1)"));
</script>
</body>
<body>
<ul id="list">
<li>item1</li>
<li>item2</li>
<li>item3</li>
<li>item4</li>
<li>item5</li>
</ul>
<script>
const ul = document.querySelector("#list");
//更新插入一个值
let h3=document.createElement("h3");
h3.innerText="晚上好";
//先把h3代替第三位
document.querySelector("li:nth-of-type(3)").replaceWith(h3);
//然后把h3代替到第一位
ul.replaceChild(h3, document.querySelector("li:nth-of-type(1)"));
//删除h3
ul.removeChild(document.querySelector("#list h3"));
</script>
</body>
<body>
<ul id="list">
<li>item1</li>
<li>item2</li>
<li>item3</li>
<li>item4</li>
<li>item5</li>
</ul>
<script>
const ul = document.querySelector("#list");
//批量增加
const Frag=new DocumentFragment();
for(let i=0;i<4;i++){
const li=document.createElement("li");
li.innerText="批量添加";
Frag.appendChild(li);
}
ul.appendChild(Frag);
//遍历,用于查询
//获取所以的子元素
console.log(ul.children);
//获取子元素的数量,就是现实子元素的长度
console.log(ul.children.length);
//更优雅的,第二种方式好
console.log(ul.childElementCount);
//第一个子元素
console.log(ul.firstElementChild);
//最后一个
console.log(ul.lastElementChild);
//获取父节点
console.log(ul.lastElementChild.parentElement);
//前一个兄弟
const jiu = document.querySelector("#list li:nth-of-type(9)");
//给他加个样式
jiu.style.color = "yellow";
//那到前一个兄弟看看内容
const ba = jiu.previousElementSibling;
ba.style.color = "green";
//拿到后一个兄弟
console.log(jiu.nextElementSibling.innerHTML);
console.log((jiu.nextElementSibling.style.color = "green"));
</script>
</body>