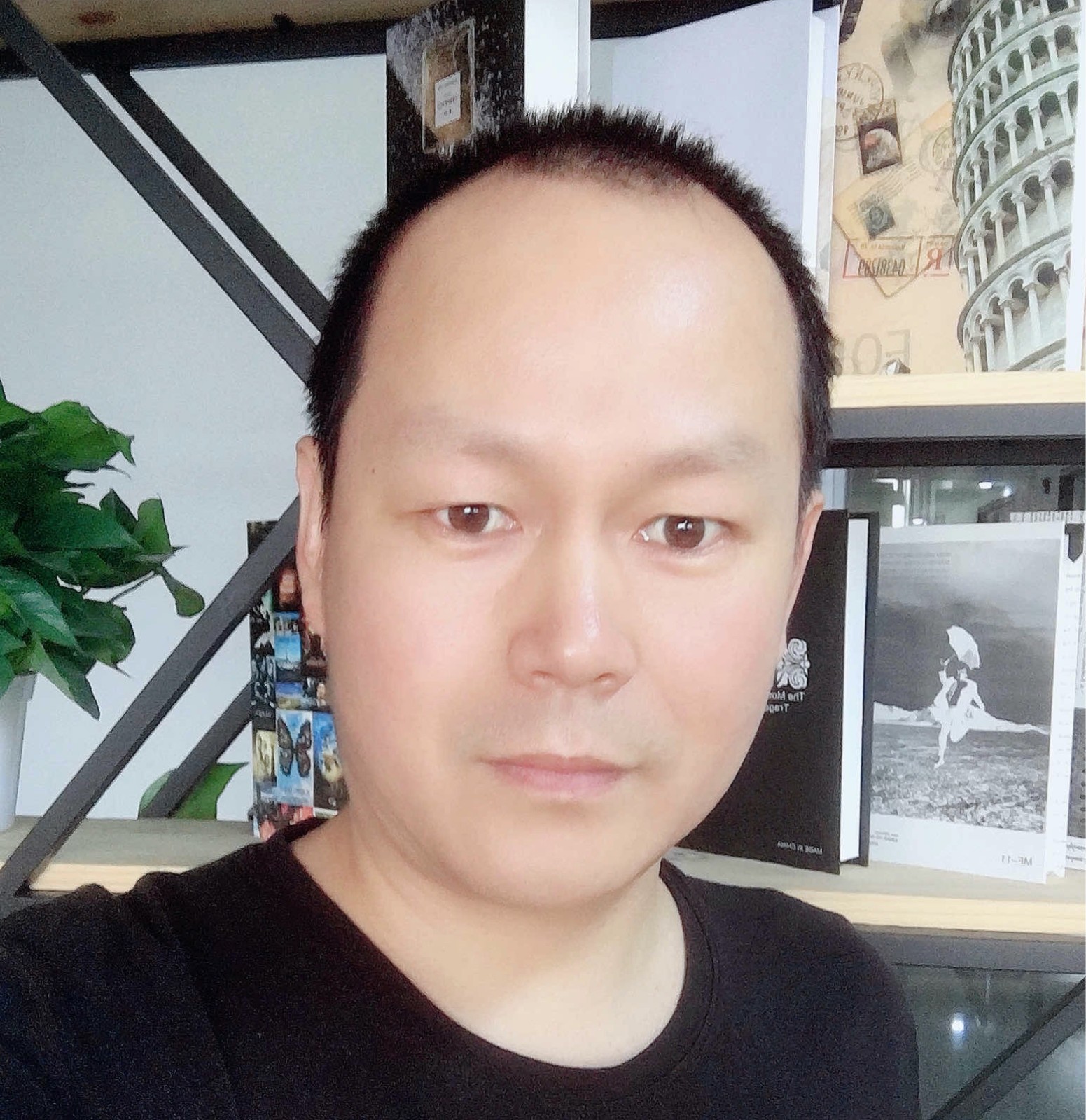
Correction status:qualified
Teacher's comments:
Vue 是一套用于构建用户界面的渐进式框架,可以自底向上逐层应用。Vue 的核心库只关注视图层,完全能够为复杂的单页应用提供驱动
<h2 class="title">{{words}}</h2>
<!-- 1. 原生 -->
<!-- <script>
document.querySelector(".title").textContent = "hello world";
</script> -->
<!-- 2. jQuery -->
<!-- <script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.5.1/jquery.js"></script>
<script>
$(".title").text("hello world...");
</script> -->
<!-- 3. vue -->
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
const vm = new Vue({
el:document.querySelector('.title'),
data:{
words: 'hello world~~~',
}
})
//vue 需要在页面上用两个{{}}做挂载点
</script>
vue 不能挂载到html和body上
<!-- 创建一个vue根节点 -->
<div class="app">
<!-- 这里的所有内容都可以用vue实例进行管理 -->
<!-- 插值就是一个数据占位符 -->
<!-- <p>用户名:{{插值表达式}}</p> -->
<p>用户名:{{username}}</p>
<!-- 插值表达式可以使用表达式 -->
<p>{{username+', 同学你吃了吗?'}}</p>
<p>10 + 30 = {{10 + 30}}</p>
<!-- 还支持三元表达式 -->
<p>{{flag ? '上午好' : '下午好'}}</p>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
const vm = new Vue({
//当前vue的配置项
//挂载点
// el: ".app",
el: document.querySelector(".app"),
//数据注入
data: {
username:'tp',
flag: true,
},
});
// 如何拿到data中的数据呢,有时会做其他处理, 在变量前面加$
// console.log(vm.$data.username);
// 在data中声明的所有的变量都自动成为vue实例的属性
// 以上的过程叫数据注入;就是将vm实例中的数据(变量)添加到dom的数据占位符中操作(过程)。
// console.log(vm.username);
// vm.username = 'TJ';
</script>
<div class="app">
<p>用户名:{{username}}</p>
<!-- v-text在HTML中不存在这样的属性,应该叫自定义属性,
专门由vue实例管理的 -->
<!-- v-text 等价于 innerText,textContent -->
<!-- <p v-text="username"></p> -->
<!-- 如果只想渲染一次需要使用v-once -->
<!-- <p v-once="username">只渲染一次</p> -->
<!-- v-html 等价于 innerHTML -->
<p v-html="username"></p>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
const vm = new Vue({
el: document.querySelector(".app"),
data: {
username: "TJ",
},
});
vm.username = '<em style="color:red">xm</em>';
</script>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>v-bind,v-on</title>
<style>
.active {
color: violet;
}
.bigger {
font-size: 2rem;
}
</style>
</head>
<body>
<div class="app" onclick="alert(this.tagName)">
<!-- 一般写法 -->
<p style="color: red">普通行内style:{{site}}</p>
<p v-bind:style="style1">style 表达式:{{site}}</p>
<!-- v-bind:是高频指令,可以简写为 : -->
<p :style="'color:red'">style 字符串:{{site}}</p>
<!-- 推荐使用反引号,便于字符串拼接 可以直接解析变量-->
<p :style="`color:red`">style 反引号:{{site}}</p>
<!-- 绑定类 -->
<!-- 1. 如果没有v-bind指令,表达式只能是字符串 -->
<!-- <p v-bind:class="表达式"></p> -->
<!-- <p :class="`active bigger `">class1:{{site}}</p> -->
<!-- <p :class="class1">类变量(表达式):{{site}}</p>
<p :class="{active: isActive,bigger: isBigger}">
类变量(优化,使用true,false控制状态):{{site}}
</p>
<p :class="[`active`,`bigger`]">绑定类-支持数组:{{site}}</p>
<p :class="[{active},{bigger}]">绑定类-支持数组内变量:{{site}}</p> -->
<!-- 绑定事件 v-on-->
<p>
<a href="https://php.cn" v-on:click="show">显示网站名称(可以跳转)</a>
</p>
<!-- 阻止a标签的默认跳转行为 -->
<!-- 事件修饰符prevent:阻止元素的默认行为 -->
<!-- v-on:也是高频指令,可以简写为 @ -->
<p>
<a href="https://php.cn" v-on:click.prevent="show"
>显示网站名称(阻止默认跳转)</a
>
</p>
<p>
<a href="https://php.cn" @click.prevent="show"
>显示网站名称(阻止默认跳转-使用v-on简写@)</a
>
</p>
<p>
<a href="https://php.cn" @click.prevent.stop="show"
>显示网站名称(阻止默认跳转-阻止冒泡)</a
>
</p>
<!-- 仅允许执行一次 -->
<p>
<a href="https://php.cn" @click.prevent.stop.once="show"
>显示网站名称(仅一次)</a
>
</p>
<!-- 事件方法的传参 -->
<!-- 事件对象的参数名必须是$event -->
<button @click.stop="handle($event, 100, 200)">click</button>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
const vm = new Vue({
el: document.querySelector(".app"),
data: {
site: "php",
style1: "color:red",
class1: "active bigger ",
isActive: false,
isBigger: true,
active: "active",
bigger: `bigger`,
},
methods: {
show() {
// this: 就是当前的vue实例
alert(this.site);
},
handle(ev, a, b) {
console.log(ev.type, ev.target);
console.log("a + b = %d", a + b );
console.log(`${a} + ${b} = ${a + b} ` );
},
},
});
</script>
</body>
</html>
v-bind: 简写为 “:” ,绑定元素的属性 :style,:class等
v-on: 简写为 “@”,@click, @input, @change等 ,修饰符 @click.prevent.stop.once
<div class="app">
<p>模型数据:{{site}}</p>
<p>单向绑定(模型数据改变页面数据跟着一起变):{{site}}</p>
<p>
双向绑定(模型数据改变页面数据跟着一起变,页面数据改变模型数据一起变化):
<input type="text" :value="site" @input="site=$event.target.value" />
</p>
<!-- 使用v-model实现双向绑定,不用书写以上 :value="site" @input="site=$event.target.value" -->
<!-- 双向绑定修饰符 lazyLoad-->
<p>
双向绑定(模型数据改变页面数据跟着一起变,页面数据改变模型数据一起变化,v-model.lazy):
<input type="text" v-model.lazy = "site" />
</p>
<p>
双向绑定(模型数据改变页面数据跟着一起变,页面数据改变模型数据一起变化,v-model.number):
<input type="text" v-model.number = "num" />
</p>
<p>{{typeof num}}</p>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
const vm = new Vue({
el: document.querySelector(".app"),
data: {
site: "PHP",
num: 0,
},
});
</script>
注意v-model修饰符 v-model.lazy,v-model.number
<div class="app">
<ul>
<!-- key:可以干预diff算法 -->
<!-- vue通过稳定且唯一的key值判断这个节点是否需要重新渲染,以提升效率 -->
<!-- 只要用了v-for 就一定要绑定key:不重复的字符串或者整数 -->
<li v-for="(item,index) in items" :key="index">
{{index}}----{{item}}
</li>
</ul>
<ul>
<li v-for="(item,prop,index) in user" :key="prop">
{{prop}}----{{index}}----{{item}}
</li>
</ul>
<ul>
<li v-for="(user,index) in users" :key="user.id">{{user.id}}----{{user.name}}----{{user.email}}</li>
</ul>
<span v-for="(n,i) in 10" :key="i">{{i}}--{{n}}<br></span>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
const vm = new Vue({
el: ".app",
data: {
// 数组
items: ["合肥", "苏州", "杭州"],
// 对象
user: {
name: "tp",
email: "tp@php.cn",
},
// 对象数组,数据表的查询结果就是一个这样的二维json
users: [
{ id: 1, name: "tp", email: "tp@php.cn" },
{ id: 2, name: "mj", email: "mj@php.cn" },
{ id: 3, name: "xm", email: "xm @php.cn" },
],
},
});
</script>
注意:使用v-for一定要绑定:key,key要求不重复的字符串或者整数;另外需要注意参数的顺序
数组:v-for=”(item,index) in items” :key=”index”
对象:v-for=”(item,prop,index) in user” :key=”prop”
对象数组:v-for=”(user,index) in users” :key=”user.id”