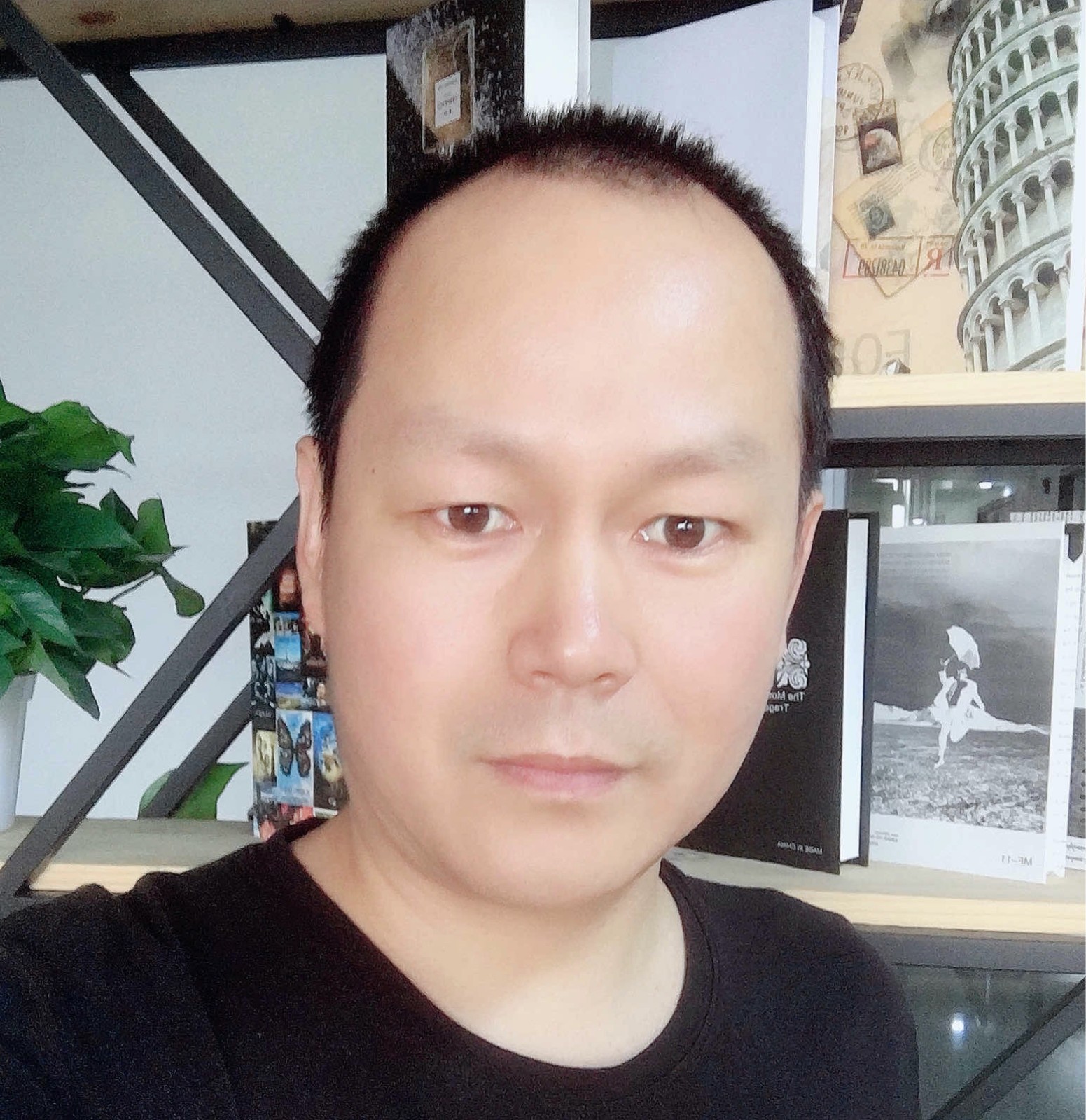
Correction status:qualified
Teacher's comments:
<div class="app">
<!-- v-for of 遍历数组 -->
<ul>
<li v-for="(item,index) of courses" :key="index">{{item}}</li>
</ul>
<!-- 对象只能用v-for in -->
<ul>
<li v-for="(item,index) in products" :key="index">
{{item.id}}-{{item.name}}-{{index}}
</li>
</ul>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
const vm = new Vue({
data: {
courses: ["html5", "css3", "es6"],
products: [
{ id: 1, name: "手机", price: 4599 },
{ id: 2, name: "大衣", price: 499 },
],
product: {
id: 1,
name: "手机",
price: 4599,
},
},
}).$mount(".app");
</script>
vue在渲染元素时候,出于效率考虑,会尽可能复用已有的元素而非重新渲染,如果不希望这样,可以使用vue提供的key属性,可以让你自己决定是否要复用元素,key的值必须是唯一的
<div class="app">
<label for="">
新老师:
<input type="text" v-model="name"
/></label>
<button @click="add">增加</button>
<ul>
<li v-for="item in list" :key="item.id">
<input type="radio" />
{{item.name}}
</li>
</ul>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
const vm = new Vue({
el: document.querySelector(".app"),
data() {
return {
name: "",
list: [
{ id: 1, name: "tp" },
{ id: 2, name: "mj" },
{ id: 3, name: "xm" },
],
};
},
methods: {
add() {
this.list.unshift({id: this.list.length+1,name:this.name})
},
},
});
</script>
v-show不能用到template上
<div class="app">
<!-- 单分支 -->
<p v-if="flag">{{msg}}</p>
<button @click="flag = !flag" v-text="tips = flag ? `隐藏` : `显示`">
隐藏
</button>
<!-- 多分支 -->
<p v-if="point > 1000 && point < 2000">{{grade[0]}}</p>
<p v-else-if="point >= 2000 && point < 3000">{{grade[1]}}</p>
<p v-else-if="point >= 3000 && point < 4000">{{grade[2]}}</p>
<p v-else-if="point >= 4000 ">{{grade[3]}}</p>
<p v-else>{{grade[4]}}</p>
<!-- v-show -->
<p v-show="status">牛年大吉</p>
</div>
<h2 style="visibility: hidden">hello world</h2>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
// v-if: 元素是否添加到页面中
// v-show:元素是否显示出来(元素已经在dom结构中)
const vm = new Vue({
el: document.querySelector(".app"),
data() {
return {
msg: "明天晴天",
flag: false,
tips: "隐藏",
point: 4000,
grade: [
"青铜会员",
"白银会员",
"黄金会员",
"钻石会员",
"仅对会员开放",
],
status:true,
};
},
});
</script>
<!-- <input type="text" />
<ul></ul>
<script>
const input = document.querySelector('input[type="text"]');
const ul = document.querySelector("ul");
input.onkeyup = (ev) => {
if (ev.key === "Enter") {
let str = `<li>${input.value}</li>`;
ul.insertAdjacentHTML("afterbegin", str);
input.value = null;
}
};
</script> -->
<div class="app">
<input type="text" @keyup.enter="submit($event)" />
<ul v-if="list.length >0">
<li v-for="(item,index) of list" :key="index">{{item}}</li>
</ul>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
const vm = new Vue({
el: document.querySelector(".app"),
data() {
return {
list: [],
};
},
methods: {
submit(ev) {
// if (ev.key === 'Enter') {
// this.list.unshift(ev.target.value);
// ev.target.value=null;
// }
// 使用键盘修饰符 .enter
this.list.unshift(ev.target.value);
ev.target.value = null;
},
},
});
</script>
其他的修饰符:
复习下事件修饰符
.stop 阻止事件继续传播(冒泡)
.prevent 阻止标签默认行为
.capture 使用事件捕获模式,即元素自身触发的事件先在此处处理,然后才交由内部元素进行处理
.self 只当在 event.target 是当前元素自身时触发处理函数
.once 事件将只会触发一次
.passive 告诉浏览器你不想阻止事件的默认行为
v-model的修饰符
.lazy 默认情况下,v-model同步输入框的值和数据。可以通过这个修饰符,转变为在change事件再同步。
.number 自动将用户的输入值转化为数值类型
.trim 自动过滤用户输入的首尾空格
键盘事件的修饰符
.enter 等效于 .13 <input v-on:keyup.13="submit">
<input @keyup.enter="submit">
.tab
.delete (捕获“删除”和“退格”键)
.esc
.space
.up
.down
<div class="app">{{words}}</div>
<script>
// vue实例从创建到销毁的全过程
// 像一个人从出生到死亡的完整流程
// vue生命周期是函数为每个阶段提供一个编程接口/钩子,开放给用户自定义
const vm = new Vue({
el: document.querySelector(".app"),
data() {
return {
words: "晚上好",
};
},
methods: {},
beforeCreate() {
console.log("实例beforeCreate:", this.$el, this.words);
},
created() {
console.log("实例created:", this.$el, this.words);
},
//挂载完成,类似 load
mounted() {
console.log("实例mounted:", this.$el, this.words);
},
//更新完成
updated() {
console.log("实例updated:", this.$el, this.words);
},
});
vm.words = "早上好";
</script>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<div class="app">
<p>
数量:
<!-- <input type="number" v-model="num" min="0" @change="calc" /></p> -->
<input type="number" v-model="num" min="0" />
</p>
<p>单价:{{price}} 元</p>
<!-- <p>金额:{{num*price}} 元</p> -->
<!-- <p>金额:{{res}} 元</p> -->
<!-- 计算属性,本质上就是原生的访问器属性 -->
<p>金额:{{amount}} 元</p>
</div>
<script>
const vm = new Vue({
el: document.querySelector(".app"),
data() {
return {
num: 0,
price: 50,
res: 0,
};
},
// 计算属性:最终会和data合并,所以不要和data中已有的属性重名
computed: {
// 访问器属性有 getter/ setter
// 计算属性默认是getter
// amount() {
// return this.num * this.price;
// },
amount: {
get() {
return this.num * this.price;
},
set(value) {
if (value > 1000) this.price *=.95;
},
},
},
methods: {
calc() {
this.res = this.num * this.price;
},
},
});
vm.amount = 1000;
</script>
<div class="app">
<p>
数量:
<input type="number" v-model="num" min="0" :max="max" />
</p>
<p>单价:{{price}} 元</p>
<p>金额:{{res}} 元</p>
</div>
<script>
const vm = new Vue({
el: document.querySelector(".app"),
data() {
return {
num: 0,
price: 50,
res: 0,
max: 100,
};
},
// 侦听器属性
watch: {
// 侦听的是某一个属性的变化,它的属性名与data中的要侦听的属性同名
num(newVal, oldVal) {
this.res = newVal * this.price;
// 监听库存()
if (newVal >= 20) {
this.max = newVal;
alert("库存不足,请补货");
}
},
},
});
// 用计算属性完成的功能,侦听器属性几乎都能完成。
</script>
重点:计算属性,侦听器属性及应用场景