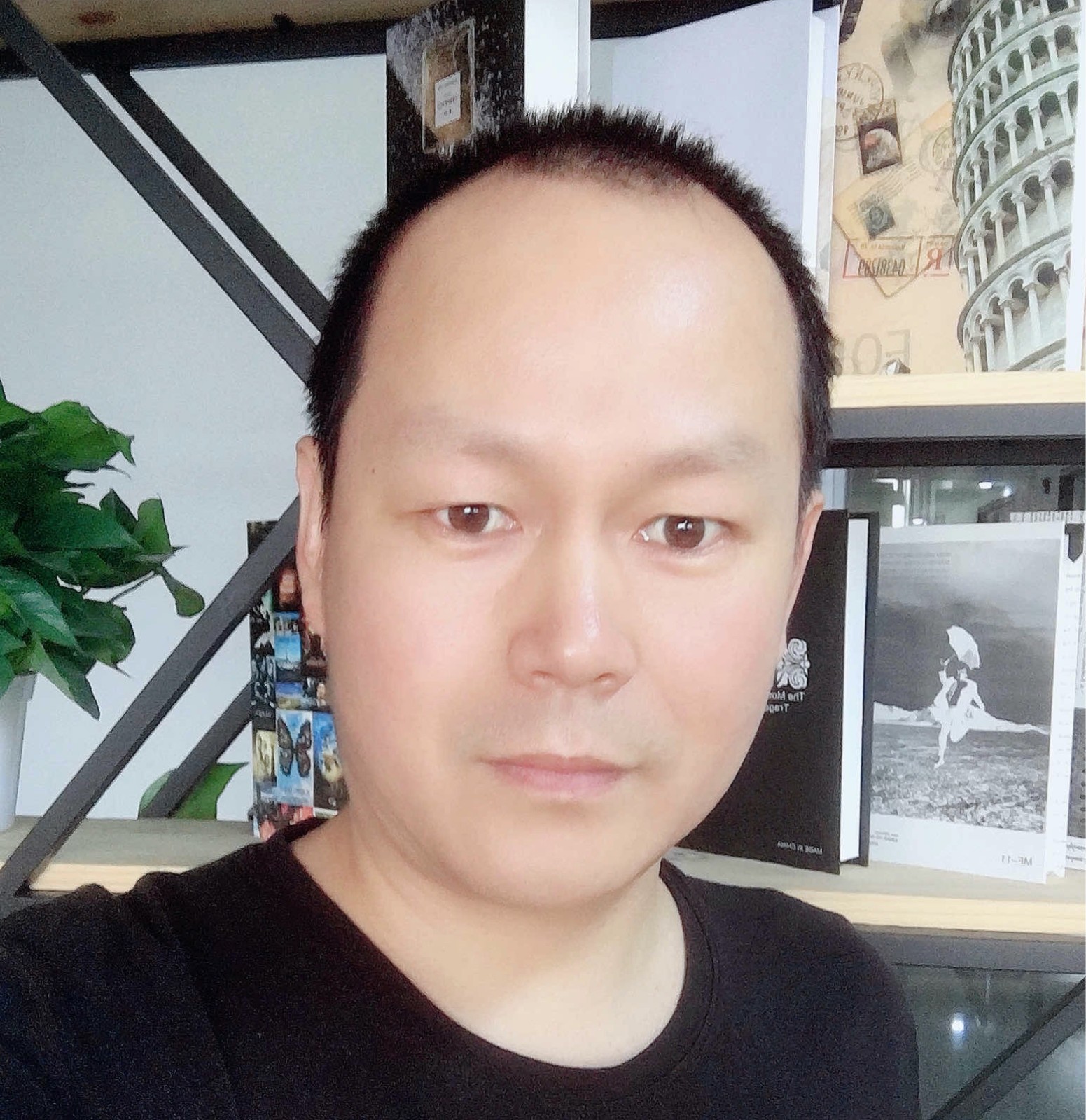
Correction status:qualified
Teacher's comments:
v-if:元素知否添加到页面中
为true时添加,为false时不添加
<p v-if='flag'>{{msg}}</p>
<script>
const vm = new Vue({
el: '#app',
data() {
return {
msg: "晚上好",
flag: true,
}
}
})
</script>
<p v-if='flag'>{{msg}}</p>
<button @click="flag=!flag" v-text="tips=flag?`隐藏`:`显示`"></button>
<script>
const vm = new Vue({
el: '#app',
data() {
return {
msg: "晚上好",
flag: true,
tips: '隐藏',
}
}
})
</script>
v-if v-else-if v-else
<p v-if="score > 100 && score < 2000">{{level[0]}}</p>
<p v-else-if="score >= 2001 && score < 3000">{{level[1]}}</p>
<p v-else-if="score >= 3001 && score < 4000">{{level[2]}}</p>
<p v-else>{{level[3]}}</p>
<script>
const vm = new Vue({
el: '#app',
data() {
return {
msg: "晚上好",
flag: true,
tips: '隐藏',
score: 10000,
level: ["铜牌", "银牌", "金牌", "王牌"],
}
}
})
</script>
v-show:元素是否显示出来(元素已经在dom中存在)
<p v-show='status'>这是v-show</p>
<script>
const vm = new Vue({
el: '#app',
data() {
return {
status: true,
}
}
})
</script>
计算属性,本质上就是原生的访问属性
计算属性最终会和data合并,所以不要和data中已有属性重名
<div id="app">
<p>数量: <input type="number" v-model='num' min="0"></p>
<p>单价:{{price}}元</p>
<p>金额:{{amout}}元</p>
</div>
<script>
const vm = new Vue({
el: '#app',
data() {
return {
num: 0,
price: 50,
res: 0
}
},
computed: {
amout: {
get() {
return this.num * this.price
},
set(value) {
console.log(value);
if (value > 1000) {
this.price = 40
}
}
}
}
})
vm.amout = 1001;
</script>
侦听的是某一个属性的值的变化,它的属性名与data中要监听的属性同名
<div id="app">
<p>数量: <input type="number" v-model='num' min="0" :max='max'></p>
<p>单价:{{price}}元</p>
<p>金额:{{res}}元</p>
</div>
<script>
const vm = new Vue({
el: '#app',
data() {
return {
num: 0,
price: 50,
res: 0,
max: 100,
}
},
watch: {
num(newValue, oldValue) {
console.log("new:" + newValue, 'old' + oldValue);
// this.res = this.num * this.price
this.res = newValue * this.price;
//监听库存
if (newValue >= 20) {
this.max = newValue;
alert('库存不足');
}
}
}
})
</script>