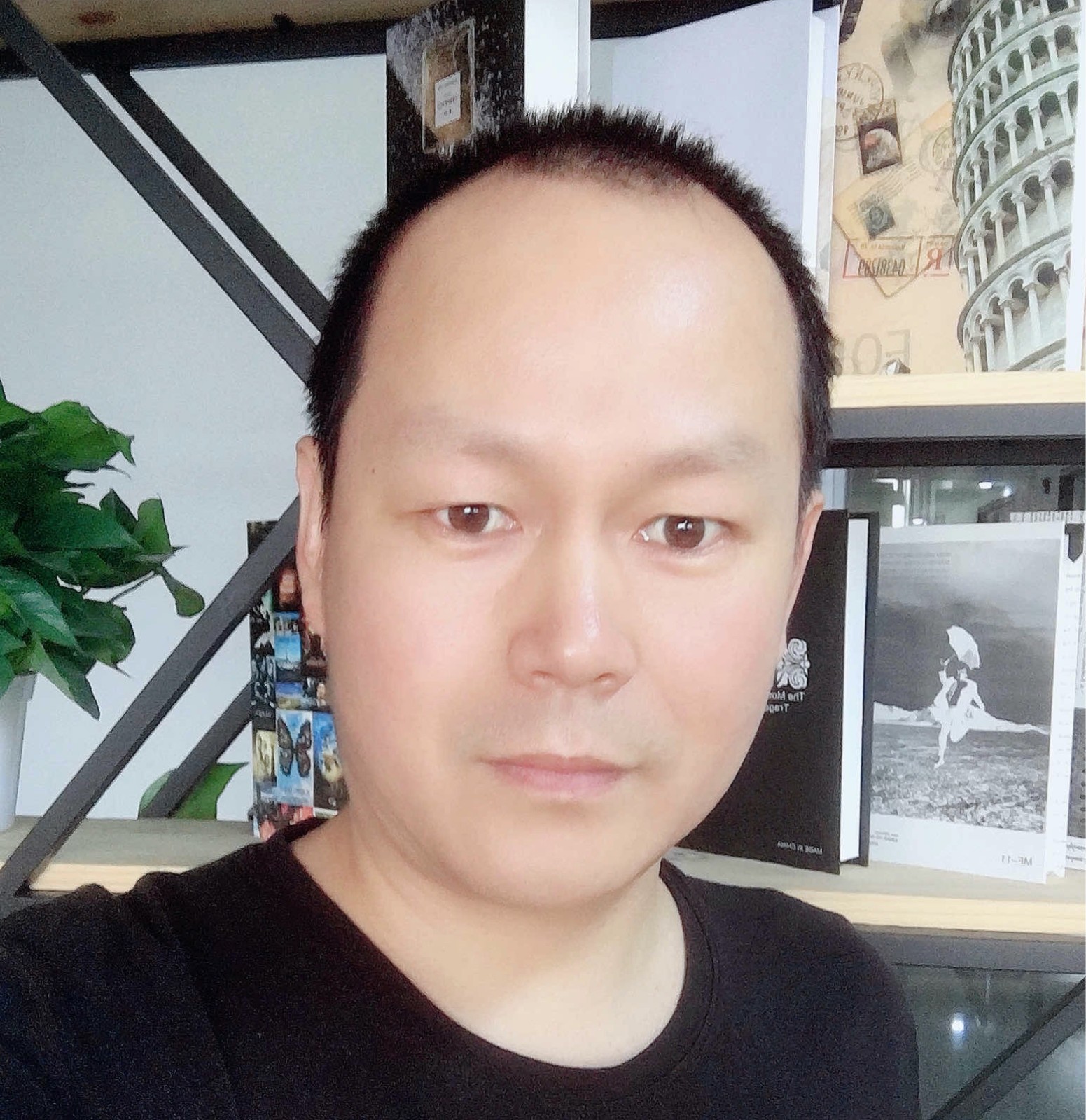
Correction status:qualified
Teacher's comments:
// 栈方法
// 1 栈 :最后进最先出
// 2 队 :先进先出
let arr = []
// 栈 puse() pop() 在尾部进行增删操作
// console.log(arr.push(5));
// console.log(arr.pop());
// 队 unshift() shift() 在数组的头部进行增删
// console.log(arr);
// console.log(arr.unshift(1, 2, 3, 4));
// console.log(arr.unshift(10));
// console.log(arr);
// console.log(arr.shift());
// console.log(arr.shift());
// push()+shift(): 模拟队列, 尾部进入,头部出去
// console.log(arr);
// console.log(arr.push(1, 2, 3));
// console.log(arr);
// console.log(arr.shift());
// console.log(arr.shift());
// console.log(arr.shift());
// pop()+unshift(): 模拟队列, 头部进入,尾部出去
// console.log(arr);
// console.log(arr.unshift(1, 2, 3));
// console.log(arr);
// console.log(arr.pop());
// console.log(arr.pop());
// console.log(arr.pop());
// 2。join() :与字符串的split()相反,它能将数组转为字符串。
// arr = ["道德经", "论语", "庄子"];
// let res = arr.join("/")
// console.log(res);
// 在js中字符串就是数组
// let str = "hello";
// console.log(str[0], str[1], str[2], str[3], str[4]);
// 3.concat()数组合并
// console.log("hello".concat("word"));
// 也可以合并其他元素
// console.log([1, 2, 3].concat(123, ["php", true], { x: 1, y: 2 }));
// 4. slice(): 返回数组中的部分成员(也可以用来创造一个数组的副本)
// arr = [1, 2, 3, 4, 5];
// let arr1 = arr;
// console.log((arr1[1] = 800));
// console.log(arr);
// 创建数组副本
// res = arr.slice(0);
// console.log(res);
// res[1] = 888;
// console.log(arr);
// console.log(res);
// 取最后二个
// console.log(arr.slice(3));
// console.log(arr.slice(-2));
// 5. splice(开始索引,删除的数量,插入的数据...): 数组的增删改,它的本职工作是删除元素
arr = [1, 2, 3, 4, 5];
// 5.1 删除
res = arr.splice(2);
// 返回被删除的元素
// console.log(res);
// 当前数组中仅有1,2
// console.log(arr);
// 5.2 更新
arr = [1, 2, 3, 4, 5];
// 最好传入单值
res = arr.splice(1, 2, ...[88, 99]);
// console.log(res);
// console.log(arr);
// 5.3 新增
arr = [1, 2, 3, 4, 5];
// 将第二个参数设置为0,这样就不会有元素被删除
res = arr.splice(1, 0, ...[88, 99]);
// console.log(res);
// console.log(arr);
// 6. sort() 排序
arr = ["p", "b", "a"];
console.log(arr);
// 默认按字母表顺序
arr.sort();
console.log(arr);
arr = [10, 9, 22, 4];
console.log(arr);
// arr.sort();
arr.sort((a, b) => a - b);
console.log(arr);
// 7. 遍历
arr = [1, 2, 3, 4, 5];
// 没有返回值
arr.forEach(item => console.log(item));
console.log(res);
// map对数组每个成员都调用回调进行处理并返回这个数组
res = arr.map(item => item * 2);
console.log(res);
// 8. 过滤
arr = [1, 2, 3, 4, 5];
// 奇数取反
res = arr.filter(item => !(item % 2));
console.log(res);
// 9. 归内
// reduce(callback(prev,curr))
arr = [1, 2, 3, 4, 5];
res = arr.reduce((prev, curr) => {
// console.log(prev, curr);
return prev + curr;
});
// 简写
res = arr.reduce((prev, curr) => prev + curr);
// 第二个参数是累加的初始值,字符串从空字符串开始
res = arr.reduce((prev, curr) => prev + curr, 5000);
console.log(res);
<script>
// 1.JSON.stringify(data,replacer,space):讲js数据转为json
// 在json中都是字符串
console.log(JSON.stringify(3.55), typeof JSON.stringify(3.55));
console.log(JSON.stringify("php.cn"), typeof JSON.stringify("php.cn"));
// 以下不加双引号
console.log(JSON.stringify(true), typeof JSON.stringify(true));
console.log(JSON.stringify(null), typeof JSON.stringify(null));
// array, object (数组,对象)
// json对象的属性必须加双引号,字符串也必须加双引号
console.log(
JSON.stringify({ x: "a", y: "b" }),
typeof JSON.stringify({ x: "a", y: "b" })
);
console.log(JSON.stringify([1, 2, 3]), typeof JSON.stringify([1, 2, 3]));
// json其实不是数据类型,只是一个具有特殊格式的字符串而已
// 会对json格式字符串的特殊操作,主要通过后面二个参数
// 第二个参数支持数组 和 函数
// 数组
console.log(JSON.stringify({ a: 1, b: 2, c: 3 }));
console.log(JSON.stringify({ a: 1, b: 2, c: 3 }, ["a", "b"]));
// 函数
console.log(
JSON.stringify({ a: 1, b: 2, c: 3 }, (k, v) => {
// 将需要过滤掉的数据直接返回undefined(json不支持undefined)
if (v < 2) return undefined;
return v;
})
);
// 第三个参数,用来格式化输出的json字符串
console.log(JSON.stringify({ a: 1, b: 2, c: 3 }, null, 2));
console.log(JSON.stringify({ a: 1, b: 2, c: 3 }, null, "****"));
// 2. JSON.parse(str,reviver),将json转为js对象
let obj = JSON.parse(`{"a":1,"b":2,"c":3}`);
console.log(obj, typeof obj);
console.log(obj.a, obj.b, obj.c);
// 第二个参数可以对json的数据进行处理后再返回
obj = JSON.parse(`{"a":1,"b":2,"c":3}`, (k, v) => {
// json对象是由内向外解析
if (k === "") return v;
return v * 2;
});
console.log(obj);
</script>
get请求
<button>ajax-get</button>
<p></p>
<script>
const bth = document.querySelector("button");
bth.onclick = () => {
// 1. 创建 xhr 对象
const xhr = new XMLHttpRequest();
// 2. 配置 xhr 参数
xhr.open("get", "test1.php?id=1");
xhr.responseType = "json";
// 3. 处理 xhr 响应
// 成功
xhr.onload = () => {
console.log(xhr.response);
// dom:将响应结果渲染到页面
let user = `${xhr.response.name} ( ${xhr.response.email} )`;
document.querySelector("p").innerHTML = user;
};
xhr.onerror = () => console.log("Error");
// 4. 发送 xhr 请求:
xhr.send(null);
}
</script>
post请求
<script>
// 拿到数据
const form = document.querySelector(".login form");
const btn = document.querySelector(".login button");
const tips = document.querySelector(".tips");
// 通过FormData() 拿数据
// let data = new FormData();
// data.append("email", form.email.value);
// data.append("password", form.password.value);
// console.log(data.get("email"), data.get("password"));
btn.onclick = ev => {
ev.preventDefault();
// 1。 创建 xhr 对象:
const xhr = new XMLHttpRequest();
// 2。 配置 xhr 参数:
xhr.open("post", "test2.php");
// 3。 处理 xhr 响应:
xhr.onload = () => (tips.innerHTML = xhr.response)
// 4。 发送 xhr 请求:
xhr.send(new FormData(form))
}
</script>
它允许浏览器向跨源服务器,发出XMLHttpRequest请求,从而克服了AJAX只能同源使用的限制。
js
<body>
<button>ajax-post-cors</button>
<p class="tips"></p>
<script>
const btn = document.querySelector("button");
const tips = document.querySelector(".tips");
btn.onclick = ev => {
// 1. 创建 xhr 对象:
const xhr = new XMLHttpRequest();
// 2. 配置 xhr 参数:
xhr.open("post", "http://world.io/cors2.php");
// 3. 处理 xhr 响应:
xhr.onload = () => (tips.innerHTML = xhr.response);
// 4. 发送 xhr 请求:
let formData = new FormData();
formData.append("email", "admin@php.cn");
formData.append("password", "123456");
xhr.send(formData);
};
</script>
php
<?php
// 在服务器端开启跨域许可。 *: 代表任何来源
// header('Access-Control-Allow-Origin: http://hello.io');
header('Access-Control-Allow-Origin: *');
echo 'CORS: 跨域请求成功';
js
const btn = document.querySelector("button");
const tips = document.querySelector(".tips");
btn.onclick = ev => {
// 1. 创建 xhr 对象:
const xhr = new XMLHttpRequest();
// 2. 配置 xhr 参数:
xhr.open("post", "http://world.io/cors2.php");
// 3. 处理 xhr 响应:
xhr.onload = () => (tips.innerHTML = xhr.response);
// 4. 发送 xhr 请求:
let formData = new FormData();
formData.append("email", "admin@php.cn");
formData.append("password", "123456");
xhr.send(formData);
};
php
<?php
header('Access-Control-Allow-Origin: *');
// 返回前端post提交的数据
print_r($_POST);
js
function getUser(data) {
console.log(data);
let user = data.name + ": " + data.email;
document.querySelector("p").innerHTML = user;
}
const btn = document.querySelector("button");
btn.onclick = () => {
// 1. 动态生成一个允许跨域请求的html元素
let script = document.createElement("script");
// 2. 将跨域请求的url添加到src属性上
script.src = "http://world.io/cors3.php?callback=getUser";
// 3. 将script挂载到页面中
document.body.insertBefore(script, document.body.firstElementChild);
};
php
<?php
// jsonp 不需要授权给前端
// 只要返回一个使用json做为参数的函数调用语句就可以
// 将前端需要的数据以json格式放到这个函数的参数中就行了
// 必须要知道前端js要调用 的函数名称
$callback = $_GET['callback'];
// 服务器中需要返回的数据
$data = '{ "name": "天蓬", "email": "tp@php.cn" }';
$data = '{ "name": "灭绝", "email": "mj@php.cn" }';
// 在后端生成一条js函数调用语句: getUser({ name: "天蓬老师", email: "tp@php.cn" });
echo $callback . '(' .$data. ')';