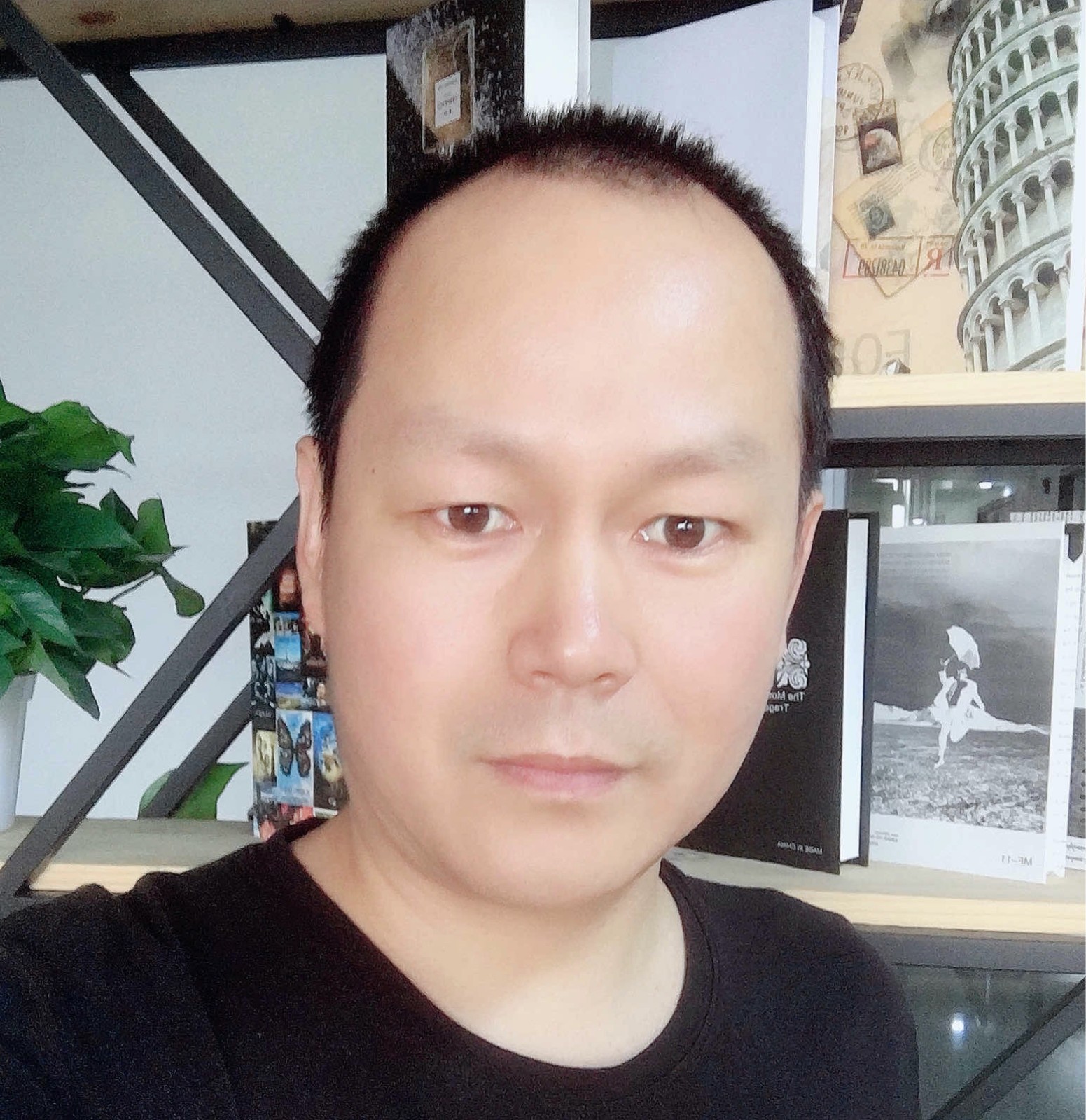
Correction status:qualified
Teacher's comments:
<ul class="course">
<li class="item">item1</li>
<li class="item">item2</li>
<li class="item">item3</li>
</ul>
let ul = document.querySelector(".course");//获取到ul元素
let liList = document.querySelector(".course .item");//获取item类第一个元素
//当需要获取到所有相同属性的元素集合时,可以使用querySelectorAll
let liList = document.querySelectorAll(".course > .item");//获取所有li
//里面元素可以使用【下标】来提取
console.log(liList[0]); //第一个,等同于querySelector();
//拿到元素集合后,可以通过foreach来遍历
liList.foreach((item,index,Array){
console.log(item,index);//index代表下标索引,item代表元素
});
//也可以将liList 转化为数组,下面提供两种转化方法
let lisArr = Array.from(liList);
let lisArr = [...liList];
//需要增加的元素li
const li = document.createElement("li");
//parent.appendChild(li); //将li元素添加到html页面中,最后位置
//想将li添加到ul中,有三种方法:
//方法1:子元素扩充,li成为ul的最后一个子元素
ul.appendChild(li);
//方法2:定义新增元素的html代码语句,用insertAdjacentHTML方法,可以指定位置
let addLiHtml = "<li style='color:red'>新增元素li</li>";
//insertAdjacentHTML方法的第一个参数:
// beforeBegin:开始之前,即已经不属于ul了
// afterBegin:开始之后,此时新增li变成ul的第一个子元素
// beforeEnd:结束之前,此时新增li变成ul的最后一个子元素
// afterEnd:结束之后,即已经不属于ul了
ul.insertAdjacentHTML("beforeEnd",addLiHtml);
//方法3:增加元素,可以指定位置
ul.insertAdjacentElement("afterBegin",li);
//需要大量添加元素时,如果一个一个加,页面就会频繁刷新,此时可以考虑用文档片断完成
const frag = new DocumentFragment();
//const frag = document.createDocumentFragment(); 这种方法创建片断也可以
for(let i=0;i<5;i++){
const li = document.createElement("li");
li.textContent = 'hello' + (i+1);
frag.appendChild(li);
}
//将片断挂到ul后面,成为ul的子元素
ul.appendChild(flag);
const h3 = document.createElement("h3");
h3.innerHTML = "晚上好";
//先查找被替换的旧元素,然后用replaceWith()将,新元素替换旧元素
document.querySelector('li:nth-of-type(3)').replaceWith(h3);、
//如果要在父元素上进行操作replaceChild(新元素,旧元素)
ul.replaceChild(h3,document.querySelector("li:nth-of-type(3)"));
//在父元素进行操作,删除子元素
ul.removeChild(document.querySelector("#list h3"));
//获取所有子元素
console.log(ul.children);
//获取所有子元素的数量
console.log(ul.children.lenght);
console.log(ul.childElementCount);
//获取第一个子元素
console.log(ul.firstElementChild);
//获取最后一个子元素
console.log(ul.lastElementChild);
//找前一个兄弟,先定位
const nine = document.querySelector("li:nth-of-type(2)");
//从定位出发,找前一个
console.log(nine.previousElementSibling.innerHTML);
//从定位出发,找后一个
console.log(nine.nextElementSibling.innerHTML);