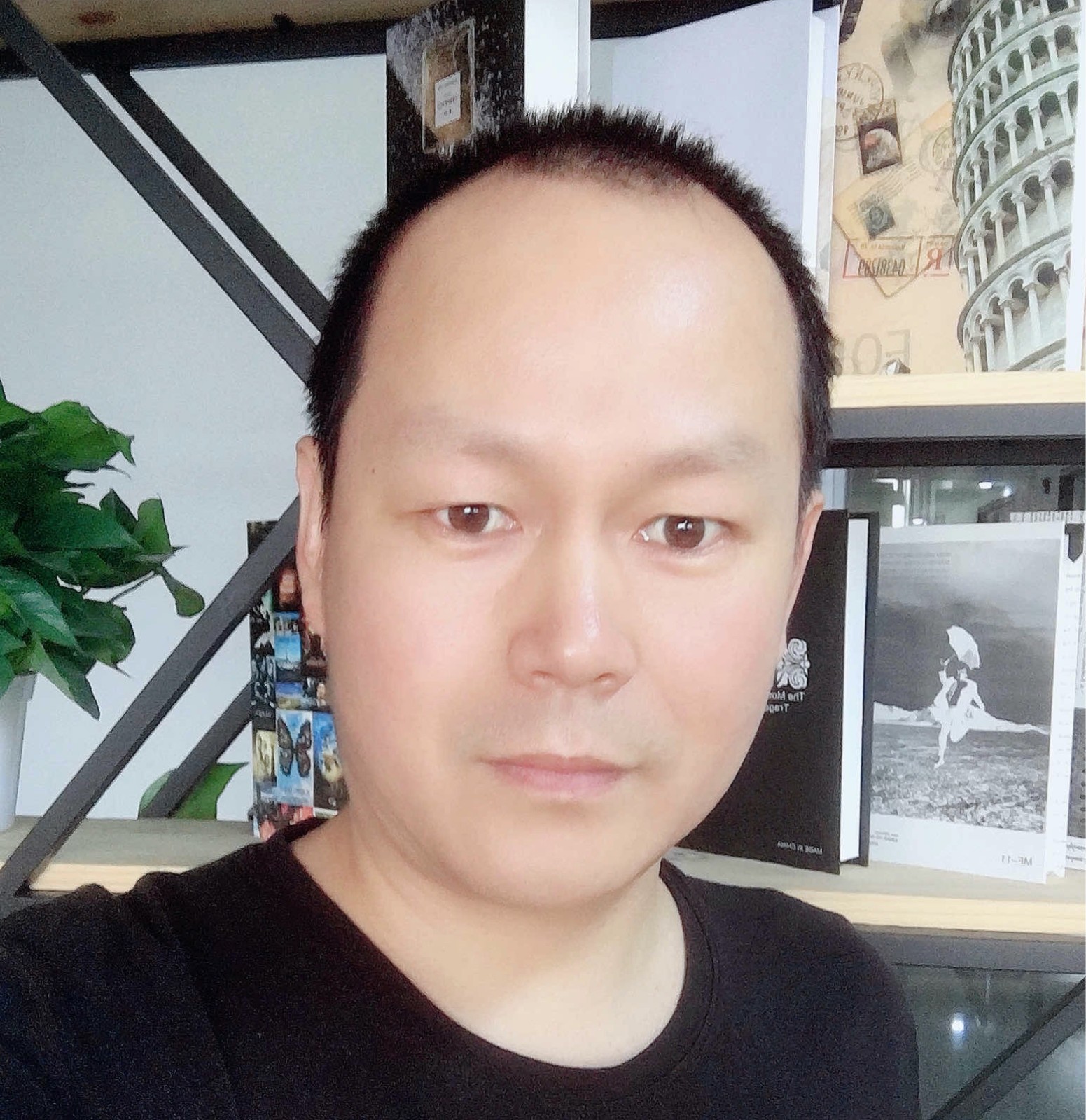
Correction status:qualified
Teacher's comments:
html 文件:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>留言板</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<form class="comment">
<label for="content">请留言:</label>
<!-- maxlength="100" 设置文本可输入的最大长度 -->
<textarea name="content" id="content" placeholder="不要超过100个字符" maxlength="100" cols="30" rows="5""></textarea>
<span class="notice">还可以输入100字</span>
<button class="submit" type="button" name="submit">提交</button>
</form>
<ul class="list"></ul>
<script>
// 获取元素
const comment = document.querySelector(".comment");
const content = comment.content;
const submitBtn = comment.submit;
const commentList = document.querySelector(".list");
const notice = document.querySelector(".notice");
// 提交按钮的点击事件
submitBtn.onclick = (ev) => {
// 获取用户的留言内容
let value = content.value;
// 创建留言元素样式
const newComment = document.createElement("li");
newComment.textContent = value;
newComment.style.borderBottom = "1px solid white";
newComment.style.minHeight = "3em";
// 添加删除留言的功能,为每条留言添加删除按钮
const deleteBtn = document.createElement("button");
deleteBtn.textContent = "删除";
deleteBtn.style.float = "right";
deleteBtn.classList.add("del-btn");
// 用户点击删除留言后的操作
deleteBtn.onclick = function(){
if(confirm('是否删除?')){
// 确定:true,取消: false
this.parentNode.remove();
alert("删除成功!")
content.focus();
return false;
}
}
// 将留言控制在 0~100 字
if(value.length > 0 && value.length <= 100){
// 将删除按钮追加到新留言的后面
newComment.append(deleteBtn);
// 将留言追加到页面中显示
commentList.prepend(newComment);
alert('留言成功');
content.value=null;
notice.textContent = `还可以输入100字`;
content.focus();
}else{
alert("没有内容或内容超出规定长度");
content.focus();
return false;
}
}
// oninput 监控输入, 提示信息
content.oninput=function(){
let textLength = content.value.trim().length;
notice.textContent = `还可以输入${100 - textLength}字`;
}
</script>
</body>
</html>
css 文件:
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
li {
list-style: none;
}
body {
background-color: rgb(174, 236, 236);
color: #555;
}
.comment {
width: 85%;
margin: 1em auto;
display: grid;
gap: 0.5em;
}
.comment #content {
resize: none;
border: none;
padding: 0.5em;
outline: none;
}
.comment #content:focus,
.comment #content:hover {
box-shadow: 0 0 8px steelblue;
transition: 0.6s;
}
.comment .submit {
width: 30%;
margin-left: auto;
background-color: lightseagreen;
border: none;
outline: none;
color: white;
height: 2.5em;
}
.comment .submit:hover {
background-color: seagreen;
transition: 0.6s;
cursor: pointer;
}
.list {
width: 80%;
/* background-color: yellow; */
margin: auto;
padding: 1em;
}
.del-btn {
background-color: wheat;
color: #000;
padding: 0.5em 1em;
/* height: 2.2em; */
border: none;
outline: none;
}
.del-btn:hover {
cursor: pointer;
background-color: rgb(31, 230, 163);
}
1)查找子字符串位置
var str = "The full name of China is the People's Republic of China.";
// indexOf():返回子字符串在字符串中第一次出现的位置(从 0 开始计算),不存在返回-1。对大小写敏感
console.log(str.indexOf("China")); // 17
// lastIndexOf():返回子字符串abc在字符串中最后一次出现的位置,对大小写敏感
console.log(str.lastIndexOf("China")); // 51
// search():方法搜索特定值的字符串,并返回匹配的位置
console.log(str.search("locate")); // -1
indexOf() 与 search() 的区别?
search() 方法无法设置第二个开始位置参数。
indexOf() 方法无法设置更强大的搜索值(正则表达式)。
2)截取
let str = "Apple, Banana, Mango";
// substr(起始位置[,截取长度]):截取长度不写则代表截取到字符串未尾
console.log(str.substr(7, 6)); // Banana
// substring(起始位置,结束位置) :提取字符串中介于两个指定下标间的字符
console.log(str.substring(7, 13)); // Banana
// slice():提取字符串的某个部分并在新字符串中返回被提取的部分
console.log(str.slice(7, 13)); // Banana
3)替换
str = "Please visit Microsoft and Microsoft!";
// replace('子字符串 1','子字符串 2'):将字符串中子字符串1,替换为子字符串2
// 默认只替换首个匹配, 对大小写敏感
console.log(str.replace("Microsoft", "MDN")); //Please visit MDN and Microsoft!
4)获取指定位置的字符
var str = "HELLO WORLD";
// charAt() 方法返回字符串中指定下标(位置)的字符串
console.log(str.charAt(0)); // H
5)转换大小写
var text = "Hello World!";
// toUpperCase() 把字符串转换为大写
console.log(text.toUpperCase()); // HELLO WORLD!
// toLowerCase() 把字符串转换为小写
console.log(text.toLowerCase()); // hello world!
6)将字符串分割为数组
var txt = "a,b,c,d,e";
// split() 将字符串转换为数组
console.log(txt.split(",")); // ["a", "b", "c", "d", "e"]
7)拼接字符串
var text1 = "Hello";
var text2 = "World";
// concat() 连接两个或多个字符串
console.log(text1.concat(" ", text2)); // Hello World
1)转为字符串
toString() //将数组转换为字符串,以逗号连接
join(‘连接符’) //将数组元素连接成字符串
// 转为字符串
let arr = [0, 1, 2, 3, 4];
console.log(arr.toString()); //0,1,2,3,4
console.log(arr.join(":")); //0:1:2:3:4
2)连接多个数组,返回新数组
concat(数组 1,数组 2,…….) //连接多个数组返回新数组
// 连接多个数组
let arr1 = [0, 1, 2, 3, 4];
let arr2 = ["true", "apple"];
console.log(arr1.concat(arr1, arr2));
//[0, 1, 2, 3, 4, 0, 1, 2, 3, 4, "true", "apple"]
3)追加元素,并返回新数组长度
unshift(值,……) //在数组的开头增加 n 个元素,并将数组的长度加 n
push(值,……) //尾部追加
let arr = [0, 1, 2, 3];
// 追加元素,并返回新数组长度
// unshift(值,值,...): 在数组开头添加元素
console.log(arr.unshift("true")); // 5
console.log(arr); //["true", 0, 1, 2, 3]
// push(值,值,...): 在数组尾部添加元素
console.log(arr.push("true")); // 5
console.log(arr); //[0, 1, 2, 3, "true"]
4)删除元素并返回该元素
shift() //删除第一个元素
pop() //删除最后一个元素
let arr = [0, 1, 2, 3];
// shift()删除第一个元素
console.log(arr.shift()); // 0
// pop()删除最后一个元素
console.log(arr.pop()); // 3
5)删除元素或删除并插入新元素
splice(开始位置,长度,[新元素,新元素,……]) //返回包括删除元素的数组
let arr = [0, 1, 2, 3, 4];
// splice(开始位置,长度,[新元素,新元素,......]):返回包括删除元素的数组
console.log(arr.splice(1, 2)); // [1, 2]
console.log(arr); // [0, 3, 4]
console.log(arr.splice(1, 2, "false")); //[1, 2]
console.log(arr); // [0, "false", 3, 4]
6)将数组元素升序排序
sort():将数组元素升序排序
let arr = [1, 2, 4, 6, 2, 5];
// sort():将数组元素升序排序
console.log(arr.sort()); // [1, 2, 2, 4, 5, 6]
7)颠倒数组中的元素
reverse():颠倒数组中的元素
let arr = [1, 2, 3, 4];
// reverse():颠倒数组中的元素
console.log(arr.reverse()); // [4, 3, 2, 1]