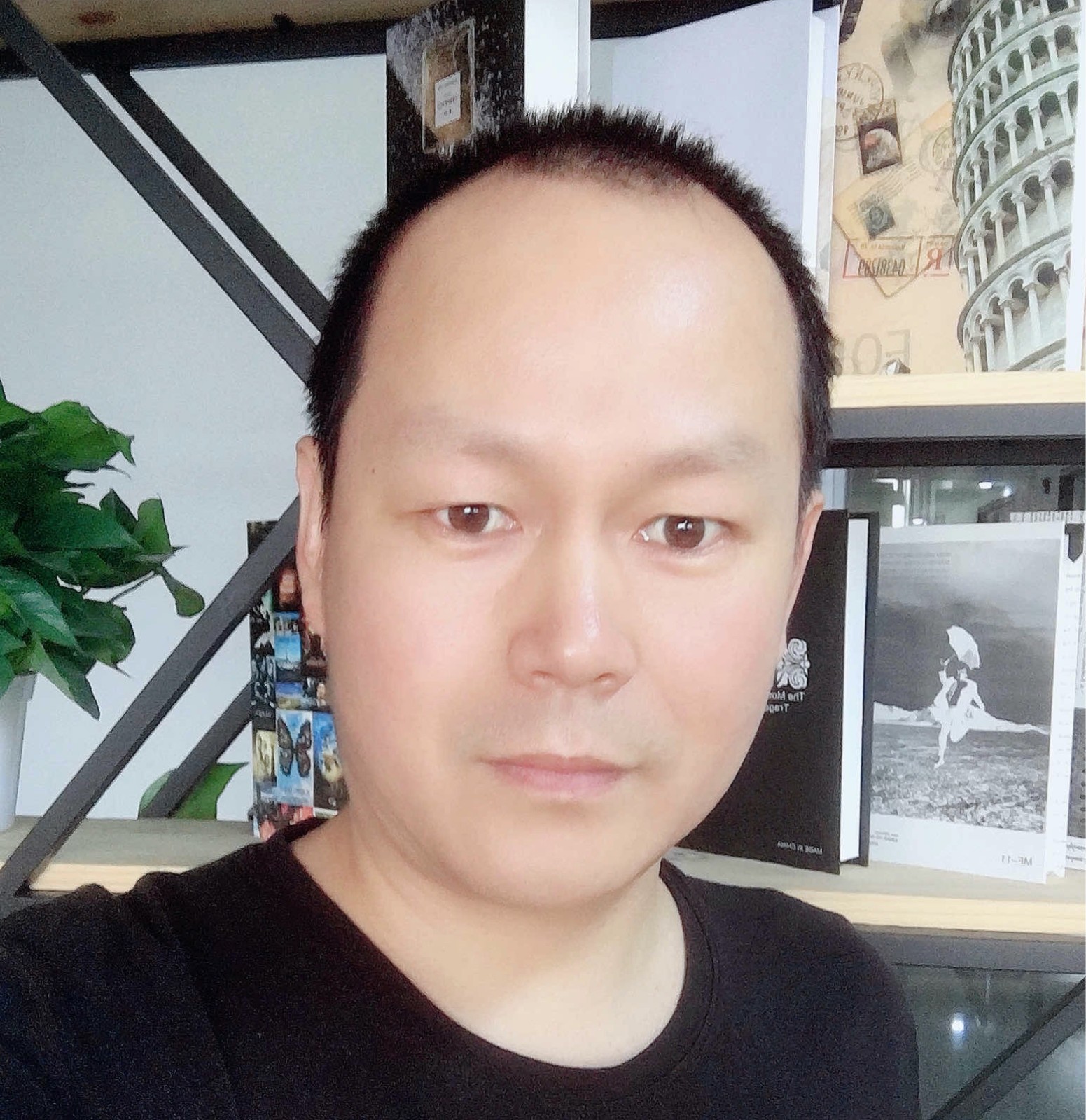
Correction status:qualified
Teacher's comments:
<!-- <div class="app" style="color: red" onclick="alert(this.textContent)">hello</div> -->
<div class="app">
<p v-bind:style="style1">{{site}}</p>
<!-- v-bind: 可简单化为":", 为元素绑定普通属性 -->
<p v-bind:style="style1, style2">{{site}}</p>
<p v-bind:style="['background: yellow', 'color: red']">{{site}}</p>
<!-- v-on: 添加事件指令 -->
<!-- <p><a href="https://php.cn" v-on:click="show">网站名称-1</a></p> -->
<!-- v-on: @ -->
<!-- prevent: 修饰符, 防止默认行 -->
<p onclick="console.log(this.tagName)"><a href="https://php.cn" @click.prevent="show">网站名称-1</a></p>
<!-- stop: 防止冒泡 -->
<p onclick="console.log(this.tagName)"><a href="https://php.cn" @click.prevent.stop="show">网站名称-1</a></p>
<!-- 事件传参 -->
<button @click.stop="calc($event, 40, 200)">计算40 + 200 = ?</button>
{{result}}
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
const vm = new Vue({
el: ".app",
data: {
site: "php中文网",
style1: "color: red",
style2: "background: yellow",
result: 0,
},
// 事件方法
methods: {
show() {
// this --> vue实例
console.log(this.site);
// debugger;
// ev.preventDefault();
},
calc(ev, x, y) {
this.result = `${x} + ${y} = ${x + y}`;
},
},
});
</script>
<div class="app">
<p>模型中的数据: {{site}}</p>
<p>
双向绑定
<input type="text" :value="site" @input="site=$event.target.value" />
</p>
<!-- vue提供一个语法糖来快速实现这个功能: v-model -->
<hr />
<!-- 实时更新绑定 -->
<p>
双向绑定/实时更新绑定
<input type="text" :value="site" v-model="site" />
</p>
<hr />
<!-- 延迟更新绑定, 修饰符: lazy -->
<p>
双向绑定/延迟更新绑定
<input type="text" :value="site" v-model.lazy="site" />
</p>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
const vm = new Vue({
el: ".app",
data: {
site: "php中文网",
},
});
// vm.site = "php.cn";
</script>
<!-- 挂载点 -->
<div class="app">
<!-- 遍历数组 -->
<ul>
<!-- <li>{{items[0]}}</li>
<li>{{items[1]}}</li>
<li>{{items[2]}}</li> -->
<!-- v-for : for of -->
<!-- :key 自定义键属性,主要是功能是干扰diff算法 -->
<li v-for="(item,index) of items" :key="index">[ {{index}} ] => {{item}}</li>
</ul>
<!-- 遍历对象 -->
<ul>
<!-- prop: 字符属性, index: 数值属性 -->
<li v-for="(item,prop, index) of user" :key="index">{{prop}}: {{index}} => {{item}}</li>
</ul>
<!-- 遍历对象数组 -->
<ul>
<li v-for="(user, index) of users" :key="user.id">{{user.id}}: {{user.name}} : [{{user.email}}]</li>
</ul>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
new Vue({
el: ".app",
data: {
// 数组
items: ["合肥", "苏州", "杭州"],
// 对象
user: {
name: "朱老师",
email: "laozhu@qq.com",
},
// 对象数组
users: [
{ id: 1, name: "朱老师", email: "laozhu@qq.com" },
{ id: 2, name: "西门老师", email: "ximeng@qq.com" },
{ id: 3, name: "灭绝老师", email: "miejue@qq.com" },
],
},
});
// v-for : (循环变量, 索引/键) in/of 数组/对象 :key
// for - of
// for - in
// for , while
</script>
<div class="app">
<!-- 单分支 -->
<p v-if="flag">{{msg}}</p>
<button @click="flag = !flag" v-text="tips = flag ? `隐藏`: `显示`"></button>
<!-- 多分支 -->
<p v-if="point > 1000 && point < 2000">{{grade[0]}}</p>
<p v-else-if="point >= 2000 && point < 3000">{{grade[1]}}</p>
<p v-else-if="point >= 3000 && point < 4000">{{grade[2]}}</p>
<p v-else-if="point >= 4000">{{grade[3]}}</p>
<p v-else>{{grade[4]}}</p>
<!-- v-show: 元素依然在源码中,只是display:none -->
<p v-show="state">前端马上就要结束了</p>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
new Vue({
el: ".app",
data() {
return {
msg: "明晚我请大家吃饭,好吗?",
flag: false,
tips: "隐藏",
grade: ["纸片会员", "木头会员", "铁皮会员", "金牌会员", "非会员"],
point: 500,
state: false,
};
},
});
</script>
<div class="app">
<p>
数量:
<input type="number" v-model="num" style="width: 5em" min="0" />
</p>
<p>单价: {{price}} 元</p>
<!-- <p>金额: {{num * price}}</p> -->
<!-- <p>金额: {{res}}</p> -->
<p>金额: {{amount}}</p>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
const vm = new Vue({
el: ".app",
data() {
return {
num: 0,
price: 50,
res: 0,
};
},
// 计算属性
computed: {
amount: {
get() {
return this.num * this.price;
},
set(value) {
console.log(value);
if (value >= 2000) this.price = 40;
},
},
},
methods: {},
});
vm.amount = 2000;
</script>
<div class="app">
<p>
数量:
<input type="number" v-model="num" style="width: 5em" min="0" :max="max" />
</p>
<p>单价: {{price}} 元</p>
<p>金额: {{res}}</p>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
const vm = new Vue({
el: ".app",
data() {
return {
num: 0,
price: 50,
res: 0,
max: 100,
};
},
// 侦听器属性
watch: {
// 是用来监听某一个属性的值的变化,属性名要和data字段中的变量名相同
// 例如,我要监听"num"变量的变化
// num(新的值,原来的值)
num(newVal, oldVal) {
console.log(newVal, oldVal);
// this.res = this.num * this.price;
// this.num --> newVal, this.price
this.res = newVal * this.price;
// 监听库存
if (newVal >= 20) {
this.max = newVal;
alert("库存不足, 请进货");
}
},
},
});
</script>