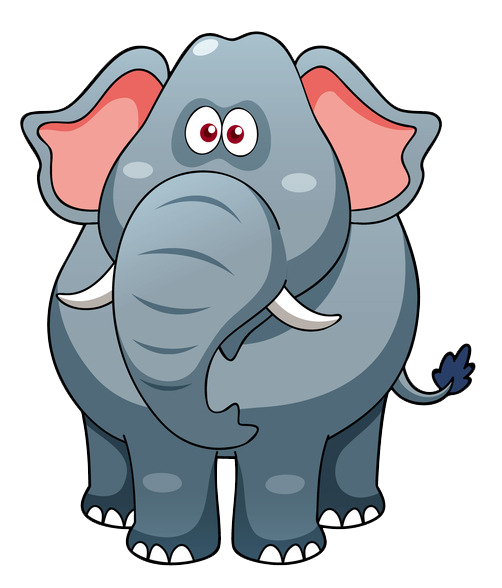
Correction status:qualified
Teacher's comments:
语法:slice(start,end)。
作用:提取字符串中指定的一部分字符。
参数:start,字符串位置索引,用于指定开始位置;end字符串位置索引,用于指定结束位置。
返回值:返回被开始位置到结束位置的字符串。原字符串不变。
示例:
<script>
let str1 = "I love China, I love Chinese people!";
let res = str1.slice(0, 3);
console.log(str1);
console.log(res);
</script>
语法:concat(string1[,string2,…])。
作用:连接两个或多个字符串。
参数:string1,要被链接的字符串,可以连接多个字符串,除了第一个字符串,后面是可选参数。
返回值:返回连接后的字符串。
示例:
<script>
let str1 = "hello";
let res = str1.concat(" China");
console.log(str1);
console.log(res);
</script>
语法:substr(start,length)。
作用:截取字符串中指定的一部分字符。
参数:start,字符串位置索引,用于指定开始位置;length要截取的字符串长度。
返回值:返回截取后的字符串。
示例:
<script>
let str1 = "I love China, I love Chinese people!";
let res = str1.substr(2, 6);
console.log(str1);
console.log(res);
</script>
语法:trim()。
作用:删除字符串两端的空白符。
参数:strging,待处理的字符串。
返回值:删除两端空白符之后的字符串。
示例:
<script>
let str1 = " hello ";
let res = str1.trim();
console.log(str1);
console.log(res);
</script>
语法:split(string)。
作用:将字符串用指定的字符串分割成数组。
参数:strging,用来分割原始字符串的“刀”。
返回值:分割之后形成的数组。
示例:
<script>
let str1 = "I love China, I love Chinese people!";
let res = str1.split(" ");
console.log(str1);
console.log(res);
</script>
语法:push(item1,[item2,…])。
作用:在数组尾部插入一些新的元素。
参数:item11,待插入的新元素,可插入多个,除了第一个元素,后面是可选参数。
返回值:新数组的长度
示例:
<script>
let arr1 = ["a", "b", "c", "d"];
console.log(arr1);
let res = arr1.push("e", "f");
console.log(arr1);
console.log(res);
</script>
语法:pop()。
作用:从数组中删除最后一个元素。
参数:无。
返回值:被删除的元素。
示例:
<script>
let arr1 = ["a", "b", "c", "d"];
console.log(arr1);
let res = arr1.pop();
console.log(arr1);
console.log(res);
</script>
语法:pop()。
作用:从数组中删除第一个元素。
参数:无。
返回值:被删除的元素。
示例:
<script>
let arr1 = ["a", "b", "c", "d"];
console.log(arr1);
let res = arr1.shift();
console.log(arr1);
console.log(res);
</script>
语法:unshift(item1,[item2,…])。
作用:在数头部插入一些新的元素。
参数:item11,待插入的新元素,可插入多个,除了第一个元素,后面是可选参数。
返回值:新数组的长度
示例:
<script>
let arr1 = ["a", "b", "c", "d"];
console.log(arr1);
let res = arr1.unshift("e", "f");
console.log(arr1);
console.log(res);
</script>
语法:splice(index,howmany,item1,…..,itemX)。
作用:在数组中添加或删除元素。
参数:index指定添加/删除项目的位置;howmany指定删除元素的个数,如果为0则不删除;item1,…..,itemX可选。向数组添加的新项目。
返回值:被删除的元素组成的新数组,如果没有删除元素则返回空数组。
示例:
<script>
let arr1 = ["a", "b", "c", "d"];
// 添加内容
let res = arr1.splice(1, 0, "e", "f", "g");
console.log(arr1);
console.log(res);
console.log("---------------------------------------");
// 删除内容
res = arr1.splice(1, 1);
console.log(arr1);
console.log(res);
</script>
<script>
let str1 = "hello";
let text = "";
for (let a = 0; a < str1.length; a++) {
text += str1[a];
}
console.log(text);
</script>
<script>
let str1 = "hello";
let text = "";
let a = 0;
while (a < str1.length) {
text += str1[a];
a++;
}
console.log(text);
</script>
<script>
let str1 = "hello";
let text = "";
let a = 0;
do {
text += str1[a];
a++;
} while (a < str1.length - 1);
console.log(text);
</script>
<script>
let a = 1;
if (a == 1) {
console.log("a =", a);
}
</script>
<script>
let a = 2;
if (a == 1) {
console.log("a =", 1);
} else {
console.log("a ≠", 1);
}
</script>
<script>
let a = new Date();
d = a.getDay();
if (d == 1) {
console.log("今天星期六");
} else if (d == 2) {
console.log("今天星期天");
} else {
console.log("今天是工作日");
}
</script>
<script>
let a = new Date();
d = a.getDay();
switch (d) {
case 6:
console.log("今天星期六");
break;
case 0:
console.log("今天星期天");
break;
default:
console.log("今天是工作日");
break;
}
</script>