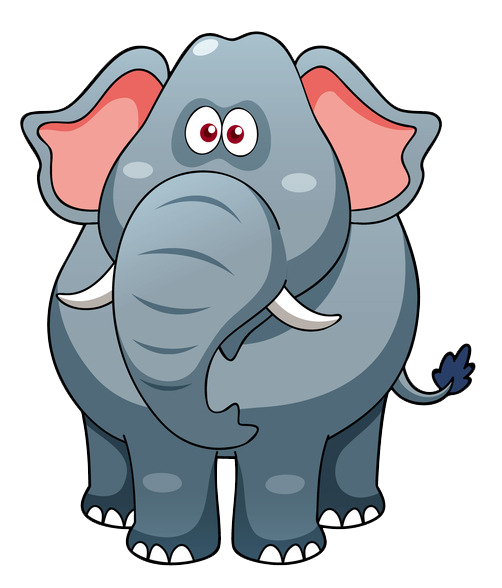
Correction status:qualified
Teacher's comments:
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>模板字面量/标签函数</title>
</head>
<body>
<script>
let username = "admin";
// 模板字面量,es6中引入的
let str = `Hello
${username}`;
// console.log(str);
// 1. 模板字面量: 支持"插值"的字符串
let nav = ["首页", "教学视频", "文章"];
let html = `
<nav>
<a href="">${nav[0]}</a>
<a href="">${nav[1]}</a>
<a href="">${nav[2]}</a>
</nav>
`;
// console.log(html);
// document.body.insertAdjacentHTML("beforeend", html);
// 2. 标签函数: 自定义模板字面量的行为
let hello = name => alert("Hello " + name);
// hello("朱老师");
// 换一种方式来调用
// hello(`朱老师`);
// hello`朱老师`;
// 其实这就是"标签函数"
// show: 将三个参数打印出来看一眼
let show = (strs, a, b) => {
console.log(strs);
console.log(a);
console.log(b);
console.log(a + b + c);
};
show = (strs, ...args) => {
console.log(strs);
console.log(args);
console.log(args[0] + args[1] + args[2]);
};
// show("10+80", 10, 80);
// 计算10+80=?
let a = 10;
let b = 80;
let c = 20;
show`${a} + ${b} + ${c}=`;
</script>
</body>
</html>
// 将一个数组中每个元素,分别创建一个变量来存储它
// let arr = [10, 20, 30];
// let a = arr[0];
// let b = arr[1];
// let c = arr[2];
// console.log(a, b, c);
// 用解构进行简化
// 等号左边是右边的模板,必须一模一样
// let [a, b, c] = [10, 20, 30];
// console.log(a, b, c);
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>对象字面量的简化</title>
</head>
<body>
<script>
let person = {
name: "朱老师",
email: "498668472@qq.com",
getInfo: function () {
// 在对象中使用 this 来访问自边的成员
return `${this.name} : ( ${this.email} )`;
},
};
console.table(person.getInfo());
// 对象解构
let { name, email } = person;
console.log(name, email);
// name = '朱老师';
// email = '498668472@qq.com'
let user = {
name: name,
email: email,
getInfo: function () {
// 在对象中使用 this 来访问自边的成员
return `${this.name} : ( ${this.email} )`;
},
};
console.log(user);
// 对象字面量中的属性值,如果引用了相同作用域中的"同名变量",则可以省去不写
user = {
// 当属性名与变量同名时,只写一个就可以了
name,
email,
getInfo: function () {
// 在对象中使用 this 来访问自边的成员
return `${this.name} : ( ${this.email} )`;
},
};
console.log(user);
user = {
name,
email,
// 将方法后面的冒号和'function'删除
getInfo() {
return `${this.name} : ( ${this.email} )`;
},
};
user = {
name,
email,
// 将方法后面的冒号和'function'删除
getInfo: () => `${this.name} : ( ${this.email} )`,
};
console.log(user);
</script>
</body>
</html>