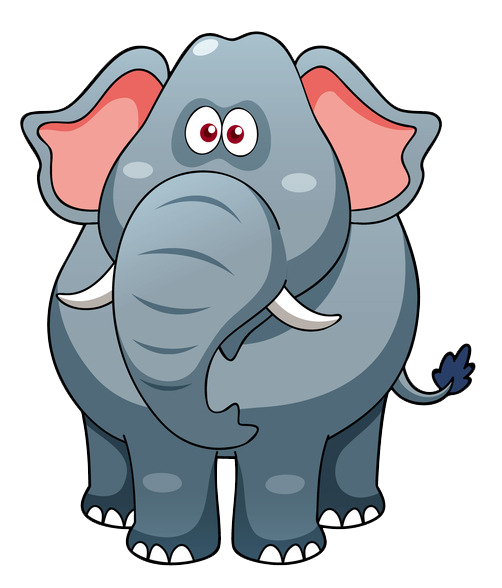
Correction status:qualified
Teacher's comments:
/* 首页 + 删除 */
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="http://libs.baidu.com/jquery/2.1.4/jquery.min.js"></script>
<title>Document</title>
<style>
* {
text-align: center;
font-size: 20px;
}
.title {
text-align: center;
}
.width {
width: 1200px;
}
tr {
height: 50px;
}
</style>
</head>
<body>
<h2 class="title">省份表</h2>
<div style="margin: 20px 200px; text-align: right">
<button id="add">添加</button>
</div>
<table class="width" id="tableId" border="1" align="center" cellspacing="0">
<thead>
<tr>
<th>编号</th>
<th>名称</th>
<th>首字母</th>
<th>拼音</th>
<th>精度</th>
<th>纬度</th>
<th>修改</th>
<th>删除</th>
</tr>
</thead>
<tbody></tbody>
</table>
<div style="margin-top: 20px">
<button id="lower">更多</button>
</div>
<script>
window.onload = function(){
onloads()
}
function onloads(){
$.get("http://admin.ouyangke.cn/index.php/api/index/prov_list",
{
// page:7,
limit:220
},function(data){
let html_data = "";
// console.log(data);
// console.log(data.data.length);
for(let i = 0;i < data.data.length; i++){
html_data += "<tr>"
html_data += "<td>" + data.data[i].area_id + "</td>";
html_data += "<td>" + data.data[i].area_name + "</td>";
html_data += "<td>" + data.data[i].pinyin + "</td>";
html_data += "<td>" + data.data[i].first_pinyin + "</td>";
html_data += "<td>" + data.data[i].lat + "</td>";
html_data += "<td>" + data.data[i].lng + "</td>";
html_data += "<td><a href='updata.html?id=" + data.data[i].area_id + "'>修改</a></td>";
html_data += "<td><button onclick='del("+ data.data[i].area_id +")'>删除</button></td>";
html_data += "</tr>"
}
$('tbody').append(html_data);
},'JSON');
$('#add').click(function(){
window.location.href = "add.html"
});
}
/* 删除 start */
function del(id){
if(confirm('确定删除?','取消')){
$.ajax({
url:"http://admin.ouyangke.cn/index.php/api/index/prov_del",
type:"post",
data:{
area_id:id,
},
dataType:"json",
success(data,status){
window.location.href = "index.html"
},
error(e){
console.log("出错啦!!:" + e);
}
});
}
}
/* 删除 end */
</script>
</body>
</html>
/* 添加 */
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Add</title>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.js"></script>
</head>
<body>
<style>
* {
background-color: #d4edda;
text-align: center;
font-size: 20px;
}
.form-control {
width: 500px;
padding: 0.375rem 0.75rem;
font-size: 1rem;
font-weight: 400;
line-height: 1.5;
color: #495057;
background-color: #fff;
background-clip: padding-box;
border: 1px solid #ced4da;
border-radius: 0.25rem;
transition: border-color 0.15s ease-in-out, box-shadow 0.15s ease-in-out;
}
button {
width: 600px;
color: #fff;
background-color: #28a745;
border-color: #28a745;
}
.select {
width: 265px;
height: 47px;
}
</style>
<h2 class="title">添加省</h2>
<form action="" onsubmit="return false;">
<p>
省名称:
<input type="text" class="form-control" id="area_name" /><span></span>
</p>
<p>
首字母:
<input type="text" class="form-control" id="pinyin" /><span></span>
</p>
<p>
拼音:
<input type="text" class="form-control" id="first_pinyin" /><span></span>
</p>
<p>
精度:
<input type="text" class="form-control" id="lng" /><span></span>
</p>
<p>
维度:
<input type="text" class="form-control" id="lat" /><span></span>
</p>
<button>按钮</button>
<div id="msg" style="margin-top: 20px; color: Red; display: none"></div>
</form>
</body>
<script>
/* 非空判断 start */
let area_name = null;
let pinyin = null;
let first_pinyin = null;
let lng = null;
let lat = null;
$('#area_name').blur(function(){
if($(this).val() != "") {
$('#area_name').next().text("");
area_name = $('#area_name').val();
// console.log(area_name)
}else{
$('#area_name').next().text("省份名称不能为空!").css('color','red');
$('#area_name').focus();
}
});
$('#pinyin').blur(function(){
if($(this).val() != "") {
$('#pinyin').next().text("");
pinyin = $('#pinyin').val();
}else{
$('#pinyin').next().text("首字母不能为空!").css('color','red');
$('#pinyin').focus();
}
});
$('#first_pinyin').blur(function(){
if($(this).val() != "") {
$('#first_pinyin').next().text("");
first_pinyin = $('#first_pinyin').val();
}else{
$('#first_pinyin').next().text("拼音不能为空!").css('color','red');
$('#first_pinyin').focus();
}
});
$('#lng').blur(function(){
if($(this).val() != "") {
$('#lng').next().text("");
lng = $('#lng').val();
}else{
$('#lng').next().text("精度不能为空!").css('color','red');
$('#lng').focus();
}
});
$('#lat').blur(function(){
if($(this).val() != "") {
$('#lat').next().text("");
lat = $('#lat').val();
}else{
$('#lat').next().text("纬度不能为空!").css('color','red');
$('#lat').focus();
}
});
/* 非空判断 end */
/* 添加 start */
$('button').click(function(){
if(area_name != null && pinyin != null && first_pinyin != null && lng != null && lat != null){
$.ajax({
url:"http://admin.ouyangke.cn/index.php/api/index/prov_add",
type:"post",
data:{
area_name: area_name,
first_pinyin: first_pinyin,
lat: lat,
lng: lng,
pinyin: pinyin
},
dataType:"json",
success(data,status){
alert('添加成功!');
console.log(status);
window.location.href = "index.html"
},
error(e){
console.log(e);
}
});
}else{
alert('请检查填写是否又空值!!!')
}
});
/* 添加 end */
</script>
</html>
/* 修改 */
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>updata</title>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.js"></script>
</head>
<body>
<style>
* {
background-color: #d4edda;
text-align: center;
font-size: 20px;
}
.form-control {
width: 500px;
padding: 0.375rem 0.75rem;
font-size: 1rem;
font-weight: 400;
line-height: 1.5;
color: #495057;
background-color: #fff;
background-clip: padding-box;
border: 1px solid #ced4da;
border-radius: 0.25rem;
transition: border-color 0.15s ease-in-out, box-shadow 0.15s ease-in-out;
}
button {
width: 600px;
color: #fff;
background-color: #28a745;
border-color: #28a745;
}
.select {
width: 265px;
height: 47px;
}
</style>
<h2 class="title">修改省</h2>
<form action="" onsubmit="return false;">
<p>
省名称:
<input type="text" class="form-control" id="area_name" /><span></span>
</p>
<p>
首字母:
<input type="text" class="form-control" id="pinyin" /><span></span>
</p>
<p>
拼音:
<input type="text" class="form-control" id="first_pinyin" /><span></span>
</p>
<p>
精度:
<input type="text" class="form-control" id="lng" /><span></span>
</p>
<p>
维度:
<input type="text" class="form-control" id="lat" /><span></span>
</p>
<button>按钮</button>
<div id="msg" style="margin-top: 20px; color: Red; display: none"></div>
</form>
</body>
<script>
// 1、修改省 -
// 获取省份:http://admin.ouyangke.cn/index.php/api/index/prov_one -
// 修改接口:http://admin.ouyangke.cn/index.php/api/index/prov_edit
// 2、删除省 -
// 删除接口:http://admin.ouyangke.cn/index.php/api/index/prov_del
/* 非空判断 start */
$('#area_name').blur(function(){
if($(this).val() != "") {
$('#area_name').next().text("");
area_name = $('#area_name').val();
// console.log(area_name)
}else{
$('#area_name').next().text("省份名称不能为空!").css('color','red');
$('#area_name').focus();
}
});
$('#pinyin').blur(function(){
if($(this).val() != "") {
$('#pinyin').next().text("");
pinyin = $('#pinyin').val();
}else{
$('#pinyin').next().text("首字母不能为空!").css('color','red');
$('#pinyin').focus();
}
});
$('#first_pinyin').blur(function(){
if($(this).val() != "") {
$('#first_pinyin').next().text("");
first_pinyin = $('#first_pinyin').val();
}else{
$('#first_pinyin').next().text("拼音不能为空!").css('color','red');
$('#first_pinyin').focus();
}
});
$('#lng').blur(function(){
if($(this).val() != "") {
$('#lng').next().text("");
lng = $('#lng').val();
}else{
$('#lng').next().text("精度不能为空!").css('color','red');
$('#lng').focus();
}
});
$('#lat').blur(function(){
if($(this).val() != "") {
$('#lat').next().text("");
lat = $('#lat').val();
}else{
$('#lat').next().text("纬度不能为空!").css('color','red');
$('#lat').focus();
}
});
/* 非空判断 end */
let id = window.location.href.split("=")[1]; // 获取首页传递的id
/* 修改绑定 start */
$.ajax({
url:"http://admin.ouyangke.cn/index.php/api/index/prov_one",
type:"post",
data:{
area_id:id,
},
dataType:"json",
success(data,status){
$('#area_name').val(data.data.area_name);
$('#pinyin').val(data.data.pinyin);
$('#first_pinyin').val(data.data.first_pinyin);
$('#lat').val(data.data.lat);
$('#lng').val(data.data.lng);
},
error(e){
console.log("出错啦!!:" + e);
}
});
/* 修改绑定 end */
/* 修改 start */
$('button').click(function(){
$.ajax({
url:"http://admin.ouyangke.cn/index.php/api/index/prov_edit",
type:"post",
data:{
area_id:id,
area_name : $('#area_name').val(),
pinyin : $('#pinyin').val(),
first_pinyin : $('#first_pinyin').val(),
lng : $('#lng').val(),
lat : $('#lat').val()
},
dataType:"json",
success(data){
console.log(data)
window.location.href = "index.html"
},
error(e){
console.log("出错啦!!:" + e);
}
});
})
/* 修改 end */
</script>
</html>