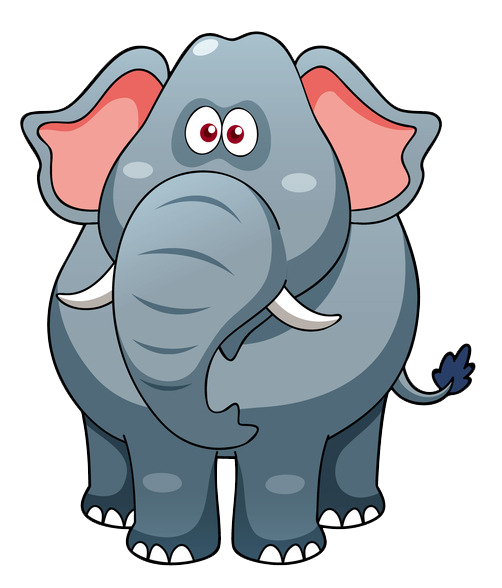
Correction status:qualified
Teacher's comments:
引入 Jquer CDN:
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.js"></script>
HTML 代码片段
<table border="1">
<tr>
<th>编号</th>
<th>名称</th>
<th>首字母</th>
<th>拼音</th>
<th>纬度</th>
<th>经度</th>
</tr>
</table>
<a href="#" id="up" >上一页</a>
<a href="#" id="lower" >下一页</a>
JS代码
<script>
var js_page = 1; //当前页
var js_pageline = 10; //每页行数
var js_count = 0; //总页数
page(js_page);
//下一页
$("#lower").click(function(){
if (js_page<js_count) {
js_page = js_page + 1;
console.log(js_page);
page(js_page);
}
})
//上一页
$("#up").click(function(){
if (js_page>1) {
js_page = js_page - 1;
console.log(js_page);
page(js_page);
}
})
//分页方法
function page(js_page){
if(js_page == 1){
$("#up").hide();
}else{
$("#up").show();
}
if(js_page == js_count){
$("#lower").hide();
}else{
$("#lower").show();
}
$.post(
"http://admin.ouyangke.cn/index.php/api/index/prov_list/",
{
page:js_page,
limit:js_pageline,
fields:"area_id",
order:"asc",
},
function(data,status){
let html_data = "";
for(let i = 0; i< data.data.length; i++){
html_data += "<tr><td>"+data.data[i].area_id+"</td><td>"+data.data[i].area_name+"</td><td>"+data.data[i].pinyin+"</td><td>"+data.data[i].first_pinyin+"</td><td>"+data.data[i].lng+"</td><td>"+data.data[i].lat+"</td></tr>";
}
$("table").html(html_data);
js_count = Math.ceil(data.count/js_pageline);
},
"json"
)
}
</script>
$.ajax 是jquery底层封装的一个方法
异步处理:ajax代码,其他代码不会受它影响,会直接执行。
URL数据接口
http://admin.ouyangke.cn/index.php/api/index/prov_add
引入 Jquer CDN:
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.js"></script>
html代码片段
<form action="" onsubmit="return false">
<table border="1">
<tr><th>名称</th><td><input type="text" id="area_name"></td></tr>
<tr><th>首字母</th><td><input type="text" id="pinyin"></td></tr>
<tr><th>拼音</th><td><input type="text" id="first_pinyin"></td></tr>
<tr><th>纬度</th><td><input type="text" id="lng"></td></tr>
<tr><th>经度</th><td><input type="text" id="lat"></td></tr>
</table>
<button id="submit" >提交</button>
</form>
JS 代码
//添加
$("form").submit(function(){
$.ajax({
url:"http://admin.ouyangke.cn/index.php/api/index/prov_add",
type:"POST",
data:{
area_name:$("#area_name").val(),
pinyin:$("#pinyin").val(),
first_pinyin:$("#first_pinyin").val(),
lng:$("#lng").val(),
lat:$("#lat").val(),
},
dataType:"json",
success(data,status){
alert(data.msg);
if (data.code!=1) {
window.location.href ="ajax_list.html"; //跳转到列表页面
}
},
})
})
URL数据接口
http://admin.ouyangke.cn/index.php/api/index/prov_edit
引入 Jquer CDN:
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.js"></script>
html代码片段
<form action="" onsubmit="return false">
<table border="1">
<tr><th>编号</th><td><span id="area_id"></span></td></tr>
<tr><th>名称</th><td><input type="text" id="area_name"></td></tr>
<tr><th>首字母</th><td><input type="text" id="pinyin"></td></tr>
<tr><th>拼音</th><td><input type="text" id="first_pinyin"></td></tr>
<tr><th>纬度</th><td><input type="text" id="lng"></td></tr>
<tr><th>经度</th><td><input type="text" id="lat"></td></tr>
</table>
<button id="submit" >修改提交</button>
</form>
JS 代码
<script>
//获取参数
var areaid = getUrlVars();
var aid = areaid["id"];
//填充数据
$.ajax({
url:"http://admin.ouyangke.cn/index.php/api/index/prov_one",
type:"POST",
data:{area_id:aid},
dataType:"json",
success(data,status){
$("#area_id").text(aid);
$("#area_name").val(data.data["area_name"]);
$("#pinyin").val(data.data["pinyin"]);
$("#first_pinyin").val(data.data["first_pinyin"]);
$("#lng").val(data.data["lng"]);
$("#lat").val(data.data["lat"]);
}
})
//修改操作
$("form").submit(function(){
$.ajax({
url:"http://admin.ouyangke.cn/index.php/api/index/prov_edit",
type:"POST",
data:{
area_id:aid,
area_name:$("#area_name").val(),
pinyin:$("#pinyin").val(),
first_pinyin:$("#first_pinyin").val(),
lng:$("#lng").val(),
lat:$("#lat").val(),
},
dataType:"json",
success(data,status){
alert(data.msg);
if (data.code!=1) {
window.location.href ="ajax_list.html";
}
},
})
})
//获取链接参数的方法
function getUrlVars()
{
var vars = [], hash;
var hashes = window.location.href.slice(window.location.href.indexOf('?') + 1).split('&');
for(var i = 0; i < hashes.length; i++)
{
hash = hashes[i].split('=');
vars.push(hash[0]);
vars[hash[0]] = hash[1];
}
return vars;
}
</script>
URL数据接口
http://admin.ouyangke.cn/index.php/api/index/prov_del
引入 Jquer CDN:
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.js"></script>
HTML 代码片段:
<table border="1">
<tr>
<th>编号</th>
<th>名称</th>
<th>首字母</th>
<th>拼音</th>
<th>纬度</th>
<th>经度</th>
<th colspan="2">操作</th>
</tr>
</table>
<a id="more" >更多</a>
<a href="ajax_add.html">增加</a>
JS代码:
<script>
var js_page = 1; //当前页
var js_pageline = 10; //每页行数
var js_count = 0; //总页数
page(js_page);
//下一页
$("#more").click(function(){
if (js_page<js_count) {
js_page = js_page + 1;
console.log(js_page);
page(js_page);
}
})
//分页方法
function page(js_page){
if(js_page == js_count){
$("#more").hide();
}else{
$("#more").show();
}
$.ajax({
url:"http://admin.ouyangke.cn/index.php/api/index/prov_list/",
data:{
page:js_page,
limit:js_pageline,
fields:"area_id",
order:"desc",
},
type:"POST",
async:true,
dataType:"json",
success(data,status){
let html_data = "";
for(let i = 0; i< data.data.length; i++){
html_data += "<tr><td>"+data.data[i].area_id+"</td><td>"+data.data[i].area_name+"</td><td>"+data.data[i].pinyin+"</td><td>"+data.data[i].first_pinyin+"</td><td>"+data.data[i].lng+"</td><td>"+data.data[i].lat+"</td><th><button onclick='toEdit("+data.data[i].area_id+")'>修改</button></th><th><button onclick='del("+data.data[i].area_id+")'>删除</button></th></tr>";
}
$("table").append(html_data);
js_count = Math.ceil(data.count/js_pageline);
}
})
}
//删除方法
function del(id){
$.ajax({
url:"http://admin.ouyangke.cn/index.php/api/index/prov_del",
data:{area_id:id},
type:"POST",
async:true,
dataType:"json",
success(data,status){
if (data.code == 0) {
alert("删除成功");
window.location.href ="ajax_list.html";
}else{
alert(data.msg);
}
}
})
}
//跳转修改页面
function toEdit(areaid) {
$.ajax({
data:{area_id:areaid},
type:"PSOT",
async:true,
dataType:"json",
complete:function(){
location.href = "ajax_edit.html?id=" + areaid;
}
})
}
</script>