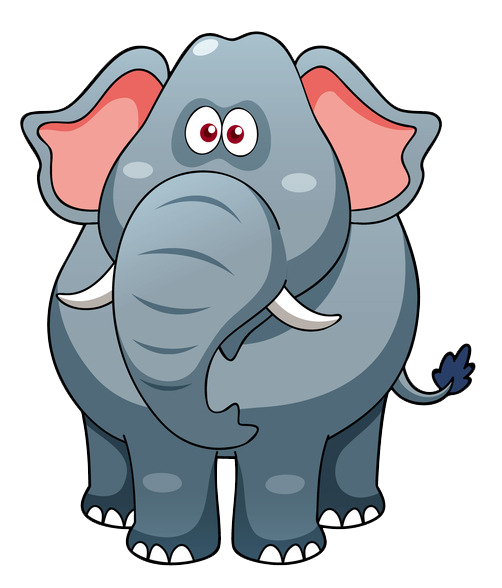
Correction status:qualified
Teacher's comments:
<?php
// 类里面定义一系列的属性和操作的方法
// 而对象就是要把属性进行实例化,然后交给类里面的方法去处理
class Persons{
public $name;
public $age;
public $height;
public $weight;
public function whoName(){
echo $this->name.' is '.$this->age.' years old and '.$this->height.' m ';
}
}
$student = new Persons;
$student->name = 'Jack';
$student->age = 20;
$student->height = 1.80;
$student->whoName();
?>
<?php
// 类里面定义一系列的属性和操作的方法
// 而对象就是要把属性进行实例化,然后交给类里面的方法去处理
// 封装是隐藏对象中的属性或方法
class Persons{
public $name;
public $age;
public $height;
// 受保护的,外部不能直接访问
protected $weight=45;
public function whoName(){
// 把受保护的属性封装在方法里面,从而外部可以看到
echo $this->name.' is '.$this->age.' years old and '.$this->height.' m <br/>';
echo $this->name.' is '.$this->weight .'kg';
}
}
$student = new Persons;
$student->name = 'Jack';
$student->age = 20;
$student->height = 1.80;
$student->whoName();
?>
<?php
class Persons{
public $name;
public $age;
public $height;
// 受保护的,外部不能直接访问
protected $weight=45;
// _constructor 构造函数
// 作用
// 1. 初始化类成员 让类/实例化的状态稳定下来
// 2. 给对象属性进行初始化赋值
// 3. 可以给私有成员,受保护的成员初始化赋值
public function __construct($name,$age,$height,$weight){
$this->name = $name;
$this->age = $age;
$this->height = $height;
$this->weight = $weight;
}
public function whoName(){
// 把受保护的属性封装在方法里面,从而外部可以看到
echo $this->name . ' is '.$this->age.' years old and '.$this->height.' m <br/>';
echo $this->name . ' is '.$this->weight .'kg';
}
}
$person = new Persons('Jack',18,1.85,80);
echo $person->whoName();
?>