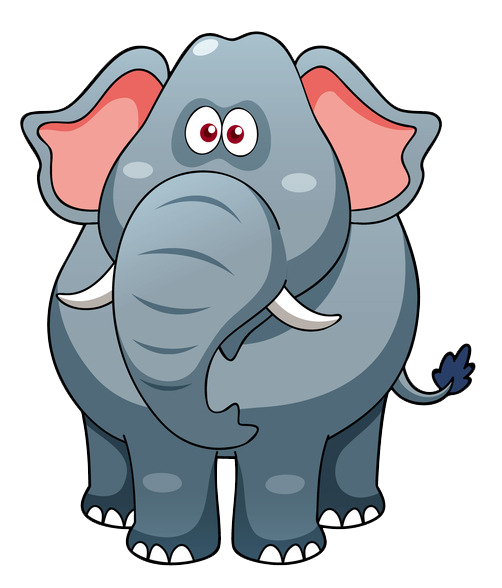
Correction status:qualified
Teacher's comments:
前端
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<form action="userUpload.php" method="post" enctype="multipart/form-data">
单文件上传<input type="file" name="pic" id="">
<input type="submit" value="上传">
</form>
<form action="userUpload.php" method="post" enctype="multipart/form-data">
多文件上传<input type="file" name="pic[]" multiple id="">
<input type="submit" value="上传">
</form>
</body>
</html>
<?php
// 文件上传类
/**
* 1 判断上传过程中是否有错误
* 2 验证上传文件的类型是否符合要求
* 3 验证上传文件的大小是否符合要求
* 4 判断上传路径和上传成功后要保存的文件名新名称
* 5 将图片从垃圾目录中移除
* 注意:
* 如果是单文件上传$_FILES['表单名称']则是一个一维数组
* 如果是多文件上传$_FILES['表单名称']则是一个二维数组,数组中name对应着每个上传文件的名称
*/
class Upload{
//成员属性
//将是检测上传过程的数组变量
public $file;
public $pic;
public $path;
public $size;
public $type;
public $newImg;
public $pathInfo = array();
//成员方法
//构造方法 初始化成员属性值
function __construct($pic,$path='./upload',$size = 5000000,array $type = array('image/jpeg','image/jpg','image/gif','image/png')){
//初始化赋值
$this->pic = $pic;
$this->file= $_FILES[$pic];
$this->path= $path;
$this->size =$size;
$this->type = $type;
}
//需要一个方法可以在外部调用进行上传文件操作
public function do_upload($mutiple = false){
if($mutiple === false){
//用户使用单文件上传方式
return $this->myExec();
}else{
$return = [];
//用户使用多文件上传方式
foreach($_FILES[$this->pic]['name'] as $k=>$v){
$this->file['name'] = $v;
$this->file['type'] = $_FILES[$this->pic]['type'][$k];
$this->file['error'] = $_FILES[$this->pic]['error'][$k];
$this->file['tmp_name'] = $_FILES[$this->pic]['tmp_name'][$k];
$this->file['size'] = $_FILES[$this->pic]['size'][$k];
$return[]= $this->myExec();
}
return $return;
}
}
//6 制作一个方法将文件上传的过程调用执行
private function myExec(){
//需要将文件上传的5步都执行一次判断是否成功
if($this->fileError() !==true){
return $this->fileError();
}elseif($this->patternType() !== true){
return $this->patterType();
}elseif($this->patternSize() !== true){
return $this->patternSize();
}elseif($this->reNameImage() !== true){
return $this->reNameImage();
}elseif($this->moveImg() !== true){
return $this->moveImg();
}else{
return $this->moveImg();
}
}
// 1 判断上传过程中是否有错误
private function fileError(){
//错误判断
if($this->file['error']){
switch($this->file['error']>0){
case 1:
return '超出PHP.INI 配置文件中的upload_max_filesize设置的值';
case 2:
return '超过了HTML表单中设置的max_file_size的值';
case 3:
return '只有部分文件被上传';
case 4:
return '没有文件上传';
case 6:
return '找不到临时目录';
case 7:
return '写入失败';
}
}
return true;
}
//2 验证上传文件的类型是否符合要求
private function patternType(){
if(!in_array($this->file['type'],$this->type)){
return '类型不合法';
}
return true;
}
// 3 验证上传文件的大小是否符合要求
private function patternSize(){
if($this->file['size']>$this->size){
return '上传文件大小超过了预设的'.$this->size.'byte大小';
}
return true;
}
// 4 判断上传路径和上传成功后要保存的文件名新名称
private function reNameImage(){
//创建保存目录,可能会遇到upload/pic/nihao/
if(!file_exists($this->path)){
mkdir($this->path,0777,true);
}
//获取图片后缀名
$suffix = strrchr($this->file['name'],'.');
//判断名称是否重复
do{
//制作新名称
$this->newImg = md5(time().mt_rand(1,1000).uniqid()).$suffix;
}while(file_exists($this->path.$this->newImg));
return true;
}
// 5 将图片从垃圾目录中移除
private function moveImg(){
if(move_uploaded_file($this->file['tmp_name'],$this->path.$this->newImg)){
$this->pathInfo['pathInfo']= $this->path.$this->newImg;
$this->pathInfo['name']= $this->newImg;
$this->pathInfo['path'] = $this->path;
return $this->pathInfo;
}else{
return '未知错误,请重新上传';
}
}
}
?>
<?php
//包含上传类,创建上传文件对象
echo '<pre>';
var_dump($_FILES);
include './upload.php';
$upload = new Upload('pic');
$res=$upload->do_upload(true);
var_dump($res);