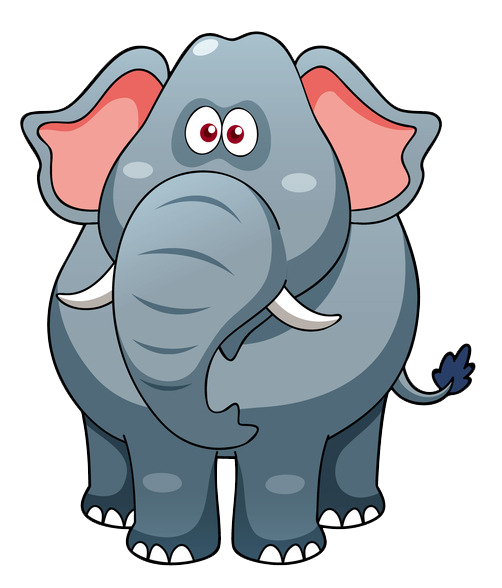
Correction status:qualified
Teacher's comments:
<?php
namespace view_demo;
//视图类
class View
{
public function fetch($data)
{
$table = '<table>';
$table.='<caption>员工信息表</caption>';
$table.='<tr><th>ID</th><th>姓名</th><th>性别</th>
<th>职务</th><th>手机号</th><th>入职时间</th></tr>';
foreach ($data as $staff) {
$table.= '<tr>';
$table.='<td>'.$staff['id'].'</td>';
$table.='<td>'.$staff['name'].'</td>';
$table.='<td>'.($staff['sex']?'男':'女').'</td>';
$table.='<td>'.$staff['position'].'</td>';
$table.='<td>'.$staff['mobile'].'</td>';
$table.='<td>'.date('Y年m月d日',$staff['hiredate']).'</td>';
$table.='</tr>';
}
$table.='</table>';
return $table;
}
}
echo '<style>
table{
border-collapse:collapse;
border:1px solid;
text-align:center;
width:500px;
height:150px;
}
caption{
font-size:1.2rem;
margin-bottom:10px;
}
tr:first-of-type{
background-color:wheat;
}
td.th{
border:1px solid;
padding:5px;
}
</style>';
require 'Model.php';
// class Model
// {
// public function getData()
// {
// return (new \PDO('mysql:host=localhost;dbname=16','root','root'))
// ->query('SELECT * FROM `staffs`')
// ->fetchAll(\PDO::FETCH_ASSOC);
// }
// }
(new \mvc_demo\model)->getData();
//echo (new View)->fetch();
<?php
namespace mvc_demo;
//模型类:通常用于数据库操作
class Model
{
public function getData()
{
return (new \PDO('mysql:host=localhost;dbname=16','root','root'))
->query('SELECT * FROM `staffs`')
->fetchAll(\PDO::FETCH_ASSOC);
}
}
<?php
namespace mvc_demo;
//加载模型类
require 'Model.php';
//加载视图类
require 'View.php';
//创建控制
class Controller2
{
public function index(Model $model,View $view)
{
//1.获取数据
$data = $model->getData();
//2.渲染视图
return $view->fetch($data);
}
public function index2(Model $model,View $view)
{
}
}
//客户端
$model = new Model;
$view = new View;
//实例化控制类
$controller = new Controller2;
echo $controller->index($model,$view);
<?php
namespace mvc_demo;
//加载模型类
require 'Model.php';
//加载视图类
require 'View.php';
//创建控制
class Controller3
{
private $model;
private $view;
public function __construct(Model $model,View $view)
{
$this->model = $model;
$this->view = $view;
}
public function index()
{
//1.获取数据
$data = $this->$model->getData();
//2.渲染视图
return $this->$view->fetch($data);
}
public function index2()
{
}
}
//客户端
$model = new Model;
$view = new View;
//实例化控制类
$controller = new Controller3($model,$view);
echo $controller->index($model,$view);
<?php
namespace mvc_demo;
//加载模型类
require 'Model.php';
//加载视图类
require 'View.php';
//服务容器
class Container1
{
//对象容器
protected $instances = [];
//bind:将对象容器中添加一个实例
public function bind($alias,\Closure $process)
{
$this->instances[$alias] = $process;
}
//make:从容器中取出一个类实例(new)
public function mark($alias,$params = [])
{
return call_user_func_array($this->instances[$alias],[]);
}
}
$container = new Container1;
$container->bind('model',function(){return new Model;});
$container->bind('view',function(){return new View;});
//创建控制器
class Controller4
{
public function index(Container1 $container)
{
//1.获取数据
$data = $container->mark('model')->getData();
//2.渲染视图
return $container->mark('view')->fetch($data);
}
}
//客户端
//实例化控制类
$controller = new Controller4();
echo $controller->index($container);
<?php
namespace mvc_demo;
//加载模型类
require 'Model.php';
//加载视图类
require 'View.php';
//服务容器
class Container2
{
//对象容器
protected $instances = [];
//bind:将对象容器中添加一个实例
public function bind($alias,\Closure $process)
{
$this->instances[$alias] = $process;
}
//make:从容器中取出一个类实例(new)
public function mark($alias,$params = [])
{
return call_user_func_array($this->instances[$alias],[]);
}
}
$container = new Container2;
$container->bind('model',function(){return new Model;});
$container->bind('view',function(){return new View;});
//Facede[]门面技术:可以接管对容器中对象的访问
class Facede
{
//容器实例属性
protected static $container = null;
//初始化方法:从外部接收一个依赖对象:服务容器的实例
public static function initialize(Container2 $container)
{
static::$container = $container;
}
}
//模型成员静态化
class Model extends Facede
{
protected static $data = [];
public static function getData()
{
static::$data = static::$container->mark('model')->getData();
}
}
//视图成员静态化
class View extends Facede
{
protected static $data = [];
public static function fetch()
{
static::$data = static::$container->mark('view')->fetch($data);
}
}
//////////////////////////////////////////////////////////////////////////////////////
//创建控制器
class Controller5
{
//构造方法:调用门面的初始化方法
public function __construct(Container2 $container)
{
Facede::initialize($container);
}
public function index(Container2 $container)
{
//1.获取数据
$data = Model::getData();
//2.渲染视图
return View::fetch($data);
}
}
//客户端
//实例化控制类
$controller = new Controller5($container);
echo $controller->index($container);