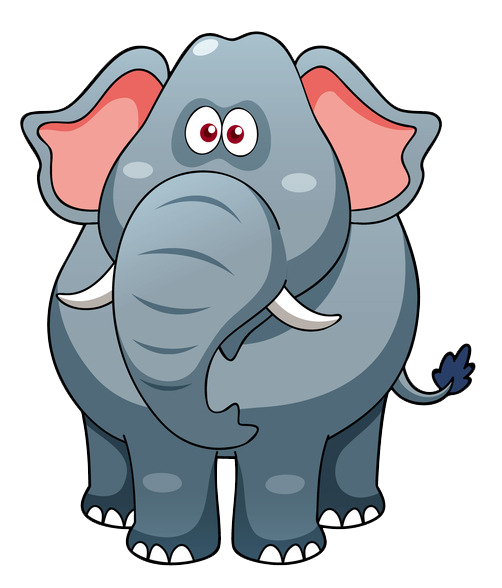
Correction status:qualified
Teacher's comments:
<?php
//运算符
$x = 88;
$y = 66;
//加
echo $x + $y;
echo '<hr>';
//减
echo $x - $y;
echo '<hr>';
//乘
echo $x * $y;
echo '<hr>';
//除
echo $x / $y;
echo '<hr>';
//取余
echo $x % $y;
echo '<hr>';
//连接
echo $x.'+'.$y.'='.$x+$y;
echo '<hr>';
//自增
$a = 99;$b = 99;
echo $a++;
echo '<br>';
echo $a;
echo '<br>';
echo ++$b;
echo '<br>';
echo $b;
echo '<hr>';
//自减
$c = 99;$d = 99;
echo $c--;
echo '<br>';
echo $c;
echo '<br>';
echo --$d;
echo '<br>';
echo $d;
echo '<hr>';
?>
结果图:
<?php
//赋值运算符
//赋值,左边等于右边,x=y
$a = 100;
echo $a;
echo '<hr>';
//加等,x+=y>>>x=x+y
$b = 1;
echo $b += 99;
echo '<hr>';
//减等,x-=y>>>x=x-y
$c = 199;
echo $c -= 99;
echo '<hr>';
//乘等,x*=y>>>x=x*y
$d = 10;
echo $d *= 10;
echo '<hr>';
//除等,x/=y>>>x=x/y
$e = 1000;
echo $e /= 10;
echo '<hr>';
//取余等于,x%=y>>>x=x%y
$f = 100;
echo $f %= 4;
echo '<hr>';
//连接
$g="Hello";
$g .= " world!";
echo $g;
?>
结果图:
<?php
//字符串函数
$nickName = 'shiro';
//strtoupper()将字符串小写字母转为大写
echo strtoupper($nickName);
echo '<hr>';
//strlen()获取字符串长度
echo strlen($nickName);
echo '<hr>';
//strtolower()将字符串大写字母转化为小写
$nickName = 'NEKO';
echo strtolower($nickName);
echo '<hr>';
//trim()去掉字符串两边的空白
echo ' study ';
echo '<br>';
echo trim(' study ');
echo '<hr>';
//strrev()反转字符串,只能反转英文
echo strrev("Hello world!");
echo '<hr>';
//strpos()函数用于检索字符串内指定的字符或文本
echo strpos("Hello world!",'w');
echo '<hr>';
//ucfirs()首字母大写
echo ucfirst("program files");
echo '<hr>';
//ucwords()把字符串中每个单词的首字符转换为大写
echo ucwords("program files");
echo '<hr>';
//str_repeat()把字符串重复指定的次数
echo str_repeat("php",3);
echo '<hr>';
//str_replace() 替换字符串中的一些字符为指定字符(区分大小写)
echo str_replace("World","php中文网","Hello World!");
echo '<hr>';
?>
结果图:
<?php
$purchase = [
[
'name' => '笔记本',
'price' => 5 ,
'number' => 200 ,
],
[
'name' => '钢笔',
'price' => 5 ,
'number' => 200 ,
],
[
'name' => '显示器',
'price' => 500 ,
'number' => 20 ,
],
[
'name' => '电脑主机',
'price' => 6000 ,
'number' => 20 ,
],
[
'name' => '键鼠套装',
'price' => 55 ,
'number' => 20 ,
],
[
'name' => '桌子',
'price' => 550 ,
'number' => 25 ,
]
];
$thead = [
'序号',
'名称',
'数量',
'单价',
'金额'
];
function table($purchase,$thead){
$table = '<table>';
$table .='<thead>'.'<tr>';
foreach ($thead as $thead_k => $thead_v) {
$table .= '<th>'.$thead_v.'</th>';
}
$table .= '</tr>'.'</thead>';
$table .= '<tbody>';
foreach ($purchase as $item_k => $items) {
$table .= '<tr>';
$table .= '<td>'.($item_k + 1).'</td>';
// $table .= '<td>'.$items['name'].'</td>';
// $table .= '<td>'.$items['price'].'</td>';
// $table .= '<td>'.$items['number'].'</td>';
// $table .= '<td>'.($items['price'] * $items['number']).'</td>';
foreach ($items as $item) {
$table .= '<td>'.$item.'</td>';
}
$table .= '<td>'.($items['price'] * $items['number']).'</td>';
$table .= '<tr>';
}
$table .= '</tbody>';
$table .= '</table>';
return $table;
}
?>
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>采购单</title>
<style>
table{
border: 1px solid black;
border-collapse: collapse;
}
td,
th {
width: 120px;
border: 1px solid black;
text-align: center;
}
</style>
</head>
<body>
<?php echo table($purchase ,$thead); ?>
</body>
</html>
结果图: