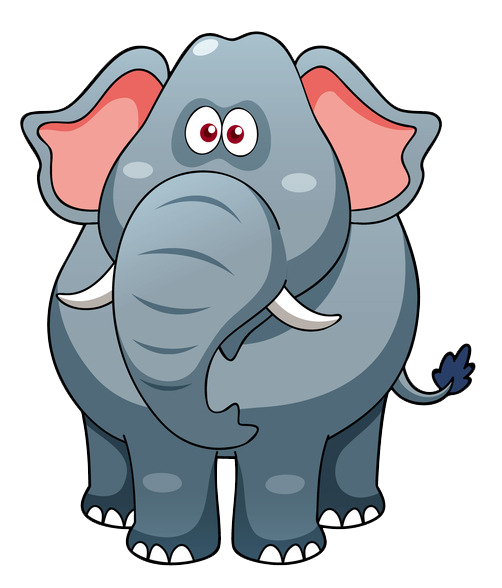
Correction status:qualified
Teacher's comments:
$int = 1;
while($int < 10){
echo '第'.$int.'次';
echo '<hr>';
$int++;
}
$int = 1;
do{
echo '第'.$int.'次';
echo '<hr>';
}while($int < 10);
continue 其中一条数据不能输出
例 break ,循环5次
for($int=1;$int<10;$int++){
echo '第'.$int.'遍';
echo '<hr>';
if(isset($_GET['num']) && $int=$_GET['num']){
break;
}
}
输出
例 continue 第5天休息
for($int=1;$int<10;$int++){
if(isset($_GET['num']) && $int=$_GET['num']){
break;
}
echo '第'.$int.'次';
echo '<hr>';
}
例 随机颜色验证码示例php代码
$code = '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ';
for($i=0;$i<4;$i++){
echo '<span style="color: rgb( ' . mt_rand(0, 255) . ' , ' . mt_rand(0, 255) . ' ,' . mt_rand(0, 255) . ' );">' . $code[mt_rand(0, strlen($code) - 1)] . '</span>';
}
输出随机不同颜色的4位验证码
echo '<table border="1">';
for ($i = 1; $i <= 9; $i++) {
echo '<tr>';
for ($y = 1; $y <= $i; $y++) {
echo '<td>';
echo $y . '*' . $i . '=' . $i * $y;
echo '</td>';
}
echo '</tr>';
}
echo '</table>';
$_REQUEST: 一维数组:包含了$_POST ,$_GET, $_COOKIE
$GLOBALS 全部全局变量的组合,是二维数组
function get_url($url, $data, $is_post = 0)
{
$ch = curl_init();
if ($is_post == 0) {
if (!empty($data)) {
$url .= '?';
foreach ($data as $k => $v) {
$url .= $k . '=' . $v . '&';
}
}
}
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_TIMEOUT, 30); // 设置curl允许执行的时间
curl_setopt($ch, CURLOPT_FOLLOWLOCATION, 1); // 爬取重定向页面
curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 3); // 在发起连接前等待的时间,如果设置为0 则无限等待
curl_setopt($ch, CURLOPT_AUTOREFERER, 1); // 自动设置referer,防止盗链
curl_setopt($ch, CURLOPT_HEADER, 0); // 显示返回 header 区域内容
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); // 要求结果保存到字符串中还是输出到屏幕上
curl_setopt($ch, CURLOPT_USERAGENT, 'Data'); // 在HTTP请求中包含一个 "User-Agent:"头的字符串
curl_setopt($ch, CURLOPT_HTTP_VERSION, CURL_HTTP_VERSION_1_1); // 强制使用 HTTP/1.1
if ($is_post == 1) {
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, $data);
}
$html = curl_exec($ch); // 去执行curl 并且打印出来,但是如果关闭了,就不会打印出来了
if (curl_errno($ch)) {
return curl_errno($ch);
}
curl_close($ch); // 关闭
return $html;
}
$data = [
'key' => '385b74677e7c5538e5fdebcf3f4e7664',
'postcode' => '646200',
'pagesize' => '5'
];
echo get_url('http://v.juhe.cn/postcode/query', $data);