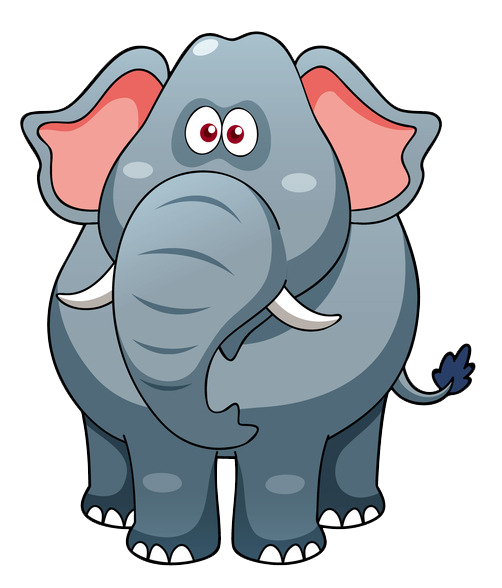
Correction status:qualified
Teacher's comments:
1. 同步任务:主线程
2. 异步任务:任务队列,使用事件循环来调度。有定时任务,事件,IO操作(input,output),http请求
定时任务:setTimeout(函数,等待时间);
3.在代码执行过程中dom渲染为同步任务,事件为异步任务,如输入框输入内容总是获取上一个输入的内容,
解决方案:
给事件套多一个定时任务,等同步的dom渲染完再执行。
使用 oninput 事件同步输入内容
// 1、事件属性
<button onclick="alert('hell')">事件属性</button>
// 2.元素对象
<button>元素对象</button>
<script>
const btn2 = document.querySelector("button:nth-of-type(2)");
btn2.onclick = () => console.log(111);
btn2.onclick = null; // 移除事件
</script>
// 3.事件监听器
<button>事件监听器</button>
<script>
const btn3 = document.querySelector("button:nth-of-type(3)");
// btn3.addEventListener(事件类型, 事件回调,是否冒泡false/true)
btn3.addEventListener("click", () => console.log(111));
let show = () => console.log(111);
btn3.addEventListener("click", show);
//移除
btn3.removeEventListener("click", show);
</script>
// 4.事件派发
<button>事件派发</button>
<script>
const btn4 = document.querySelector("button:nth-of-type(4)");
let i = 0;
btn4.addEventListener("click", () =>{
console.log("恭喜你,又赚了:" + i + "元");
i += 0.5;
});
// 创建一个自定义事件
// setTimeout: 定时器,用于执行一次性的定时任务
// setInterval: 定时器,用于执行间歇性的定时任务
const myclick = new Event("click");
setInterval(() => btn4.dispatchEvent(myclick),3000);
</script>
// 事件冒泡
<script>
function show(ev){
// ev: 事件对象
// console.log(ev.type);
// ev中有二个重要属性
// 1. 事件绑定者(主体)
console.log(ev.currentTarget);
// 2.事件触发者(目标)
console.log(ev.target);
console.log(ev.target === ev.currentTarget);
}
const lis = document.querySelectorAll("li");
// 循环给每一个li添加点击事件
lis.forEach(li => (li.onclick = ev => console.log(ev.currentTarget)));
// onclick这种通过事件属性的添加的事件,是冒泡事件
// 冒泡:子元素的同名事件,会沿着dom树向上逐级触发上级元素的同名事件
// document.querySelector("ul").onclick = ev => console.log(ev.currentTarget);
// document.querySelector("body").onclick = ev => console.log(ev.currentTarget);
// document.documentElement.onclick = ev => console.log(ev.currentTarget);
document.querySelector("ul").onclick = ev =>{
// 1.事件绑定者
// console.log(ev.currentTarget);
// 2.事件触发者
// console.log(ev.target);
console.log(ev.target.textContent);
}
</script>
<form action="" method="POST" id="login" onsubmit="return false"> <!--onsubmit:禁用表单事件-->>
<label class="title">用户登录</label>
<label for="email">邮箱:</label>
<input type="email" id="email" name="email" value="" autofocus>
<label for="password">密码:</label>
<input type="password" id="password" name="password">
<button name="submit">登录</button>
</form>
<script>
const login = document.forms.login;
// submit: 提交事件
// login.onsubmit = () => console.log("提交了");
// login.onsubmit = ev.preventDefault();
login.submit.onclick = ev =>{
// 禁止冒泡
ev.stopPropagation();
// 每个表单元素都有一个form属性,与所属的表单绑定
// console.log(ev.currentTarget.form);
// 非空验证
isEmpty(ev.currentTarget.form);
};
function isEmpty(form){
console.log(form.email.value.length);
console.log(form.password.value.trim().length);
if(form.form.email.value.length === 0){
alert("邮箱不能为空");
form.email.focus();
return false;
}else if(form.password.value.trim().length === 0){
alert("密码不能为空");
form.email.focus();
return false;
}else{
alert("验证通过");
}
}
</script>
<script>
// json字符串
let jsonstr = `{
"id" : 111,
"name" : "js",
"price" : 99
}`;
// 将json数据转换为js对象
let book = JSON.parse(jsonstr);
console.log(book);
let html = `
<ul>
<li>书名:${book.id}</li>
<li>名称:${book.name}</li>
<li>价格:${book.price}</li>
</ul>
`;
// 渲染到HTML页面
document.body.insertAdjacentHTML("afterbegin",html);
</script>
<script>
// 使用链式操作
// fetch(url).then(响应回调).then(结果处理)
// console.log(fetch("https://php.cn/"));
// response :成功响应回调的响应值
fetch("data.json")
.then(response => response.json())
.then(data => console.log(data));
//json测试数据网站
fetch("https://jsonplaceholder.typicode.com/todos")
.then(response => response.json())
.then(data => console.log(data));
// async 异步函数,返回 Promise
// await : 等待,用来执行耗时操作,完成异步任务
async function f1(){
return await console.log("aaa");
}
</script>
<button onclick="getList()">查看留言列表</button>
<div id="box">
<!-- 这里用来显示全部数据 -->
</div>
<script>
// 创建异步函数
async function getList(){
// 请求数据
// 使用 await 请求数据,避免数据过多影响主线程,造成阻塞,使页面加载数据卡顿
const response = await fetch("https://jsonplaceholder.typicode.com/comments");
// 将数据转化为json格式
const comments = await response.json();
console.log(comments);
// 返回,将数据渲染到页面中
render(comments);
}
function render(data){
const box = document.querySelector('#box');
const li = document.createElement('lo');
data.forEach(item =>{
console.log(item);
const li = document.createElement("li");
li.textContent = item.body.slice(0,120) + "...";
// textContent : 只支持存文本
// innerHTML : 支持html标签,并解析
li.innerHTML = `<span style="color:green">${item.body.slice(0,120)}...</span><hr>`;
ol.append(li);
});
box.append(ol);
}
</script>