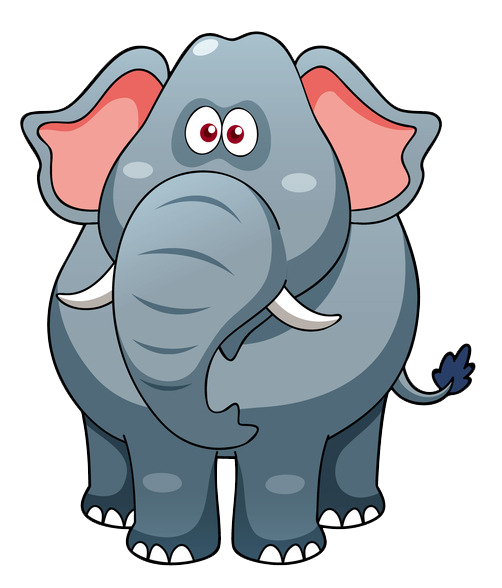
Correction status:qualified
Teacher's comments:
class Father{
//可继承
public $name = 'admin';
protected $gender = 'male';
//不可继承
private $age = 18;
public function __construct($n,$g,$a)
{
//在构造函数内都可赋值
$this->name=$n;
$this->gender=$g;
$this->age=$a;
}
public function info(){
//在本类方法内调用
return "father-uname:{$this->name},father-gender:{$this->gender},father-age:{$this->age}";
}
}
class Son extends Father{
//由于父类的$age无法继承过来,我们给子类重新加一个$son_age
private $son_age = 18;
//构造方法重写
public function __construct($n,$g,$a)
{
$this->name=$n;
$this->gender=$g;
$this->son_age=$a;
}
//普通方法重写
public function info(){
//由于父类的$age无法继承过来,我们给子类重新加一个$son_age
return "son-uname:{$this->name},son-gender:{$this->gender},son-age:{$this->son_age}";
}
}
$father = new Father('admin1','male',28);
echo $father->name;
echo '<hr>';
echo $father->info();
echo '<hr>';
echo '<hr>';
$son = new Son('admin','male',18);
echo $son->name;
echo '<hr>';
echo $son->info();
构造方法除了可以给共有变量重新赋值,也可以给受保护的活私有变量重新赋值
//禁止父类被继承
final class Father{
public $name = 'admin';
//禁止方法被重写
final public function uname(){
echo 'admin222';
}
}
spl_autoload_register(
function($classname){
require_once $className .'php';
}
);
self::$username
,类似于普通属性调用中的$this
class Father{
public $name;
static $uname = '静态admin';
public $num1 = 1;
static $num2 = 1;
public function add1(){
$this->num1++;
self::$num2++;
return '通过计数查看静态成员为所有实例共享,我是普通成员的数字'.$this->num1.',我是静态成员的数字'.self::$num2;
}
public function add2(){
//静态属性无法销毁,如果想做到与普通属性一样的效果,只能在开头给其重新赋值
self::$num2 = 1;
$this->num1++;
self::$num2++;
return '通过计数查看静态成员为所有实例共享,我是普通成员的数字'.$this->num1.',我是静态成员的数字'.self::$num2;
}
//静态方法由于也是不用实例化就能调用,因此内部没法使用$this调用的普通成员
public static function sta(){
return '我只能调取静态的变量'.self::$uname;
}
}
$fa1 = new Father();
$fa2 = new Father();
$fa3 = new Father();
$fa4 = new Father();
$fa5 = new Father();
$fa6 = new Father();
echo $fa1->add1();
echo '<hr>';
echo $fa2->add1();
echo '<hr>';
echo $fa3->add1();
echo '<hr>';
echo $fa4->add1();
echo '<hr>';
echo $fa5->add1();
echo '<hr>';
echo $fa6->add1();
echo '<hr>';
echo $fa1->add2();
echo '<hr>';
echo $fa2->add2();
echo '<hr>';
echo $fa3->add2();
echo '<hr>';
echo $fa4->add2();
echo '<hr>';
echo $fa5->add2();
echo '<hr>';
echo $fa6->add2();