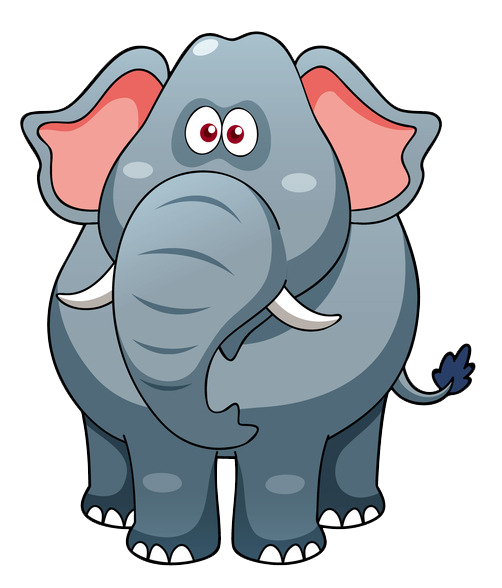
Correction status:qualified
Teacher's comments:
<?php require_once "header.php" ?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>显示用户信息</title>
<link rel="stylesheet" href="bootstrap.css">
<style>
/* 1. 添加表格线,添加给单元格 */
tbody td,
th {
border: 1px solid;
}
/* 2. 折叠表格线 */
table {
border-collapse: collapse;
width: 90%;
/* 对齐 */
text-align: center;
margin: 2em auto;
}
/* 标题 */
table thead {
background-color: lightskyblue;
line-height: 80px;
}
table tbody{
line-height: 80px;
}
tbody tr:nth-of-type(2n){
background-color: #ddd;
}
.show h2{
text-align: center;
}
</style>
</head>
<body>
<?php
require_once "auto.php";
$p=$_GET['p']??1;
$count=5; //每页显示的记录条数
$data=UserContr::GetAllUserPage($p,$count);
//总记录行数
$rowCount=UserContr::GetRowCount();
$pages=ceil($rowCount/$count);
?>
<div class="show">
<h2>用户信息</h2>
<table>
<thead>
<tr>
<th><input type="checkbox" id="check_all"></th>
<th>ID</th>
<th>用户名</th>
<th>姓名</th>
<th>电话</th>
<th>邮箱</th>
<th>创建时间</th>
<th>最后登录时间</th>
<th>状态</th>
</tr>
</thead>
<tbody>
<?php foreach($data as $user): ?>
<tr>
<td><input type="checkbox" class="chk_item"></td>
<td class="id"><?=$user->id?></td>
<td><a href="edituser.php?id=<?=$user->id?>" title="点击修改用户信息" ><?=$user->account?></a></td>
<td><?=$user->name?></td>
<td><?=$user->phone?></td>
<td><?=$user->email?></td>
<td><?=date('Y-m-d',$user->createtime)?></td>
<td><?=date('Y-m-d',$user->updatetime)?></td>
<td><?=$user->state==1?'开启':'关闭'?></td>
</tr>
<?php endforeach ?>
</tbody>
<tfoot>
<tr>
<td colspan="9">
<nav aria-label="...">
<ul class="pagination">
<li class="page-item <?=$p==1?'disabled':''?>">
<a class="page-link" href="showuser.php?p=<?=$p-1 ?>" tabindex="-1" aria-disabled="true">上一页</a>
</li>
<?php for($i=1;$i<=$pages;$i++): ?>
<li class="page-item <?=$p==$i?'active':''?>"><a class="page-link" href="showuser.php?p=<?=$i ?>"><?=$i ?></a></li>
<?php endfor ?>
<li class="page-item <?=$p==$pages?'disabled':''?>">
<a class="page-link " href="showuser.php?p=<?=$p+1 ?>">下一页</a>
</li>
</ul>
</nav>
</td></tr>
<tr><td colspan="3"><button onclick="del()">删除</button></td>
<td><a href="adduser.php">添加用户</a></td>
</tr>
</tfoot>
</table>
</div>
<script>
const chkAll=document.querySelector("#check_all");
const chkItems=document.querySelectorAll(".chk_item");
chkAll.onchange=ev=>chkItems.forEach(item=>item.checked=ev.target.checked);
chkItems.forEach(item=>item.onchange=()=>chkAll.checked=[...chkItems].every(item=>item.checked));
function del(){
if(confirm('您确定要删除这些用户吗?')){
const chkItems=document.querySelectorAll(".chk_item");
chkItems.forEach(item=>{
if(item.checked){
const id=item.parentElement.nextElementSibling.textContent;
let isDel=delOpt(id);
if(isDel){
// console.log( item.parentElement.parentElement);
item.parentElement.parentElement.remove();
}
}
})
}else{
alert("NO");
}
}
async function delOpt(id){
const response = await fetch("del_user.php?id=" + id);
const comments = await response.json();
return comments;
}
</script>
<script src="./js/jquery-3.5.1.min.js"></script>
<script src="./js/bootstrap.bundle.js"></script>
</body>
</html>
<?php
require_once "auto.php";
class UserContr{
// 分页显示
public static function GetAllUserPage($p,$count){
$start=($p-1)*$count;
$sql="SELECT * FROM `php_user` LIMIT $start ,$count";
$pdo =CreatePDO::Create();
$pre=$pdo->prepare($sql);
$exec=$pre->execute();
$data = $pre -> fetchAll(PDO::FETCH_OBJ);
return $data;
}
//记录行数
public static function GetRowCount(){
$sql="SELECT count(*) as rowCount FROM `php_user`";
$pdo=CreatePDO::Create();
$pre=$pdo->prepare($sql);
$exec=$pre->execute();
$res=$pre->fetch(PDO::FETCH_ASSOC);
return $res['rowCount'];
}
}
<?php
class CreatePDO{
public static function Create(){
try{
$pdo = new PDO('mysql:host=127.0.0.1;dbname=prodb','root','123456');
}catch(PDOException $e){
// 抛出错误,错误是你可以定义的
echo json_encode(['status'=>9,'msg'=>'数据库链接失败']);
exit;
}
return $pdo;
}
}