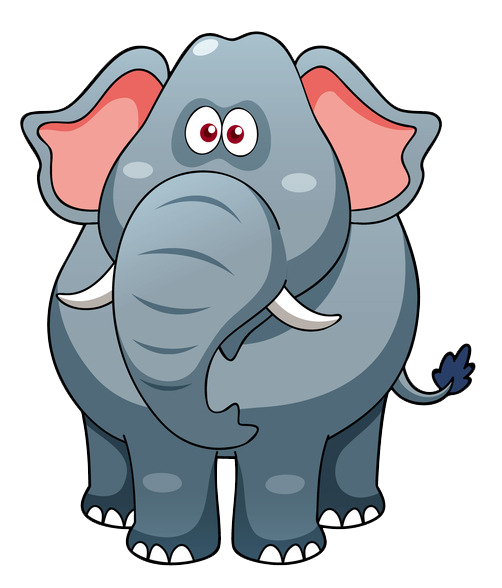
Correction status:qualified
Teacher's comments:
/*作用:
1、use 引用彼得命名空间到当前空间
引用别的命名空间的类到当前空间
2、as给重复命名空间改别名
*/
namespace app\admin\model
{
class Index{
public static function index(){
return '我是model空间里的Index类的index方法';
}
}
}
namespace app\admin\controller {
class Index
{
public static function index()
{
return '我是one空间里的Index类的index方法';
}
}
// 引入model后 app\admin\ 可以去掉
// use \app\admin\model;
// echo Index::index();
// echo '<hr>';
// echo model\Index::index();
use \app\admin\model\Index as mIndex;
echo mIndex::index();
}
//\index.php
namespace box;
require_once 'app\admin\autoload.php';
//use 引入的命名空间要跟硬盘的目录结构对应上
use \app\admin\controller\User;
$user=new User();
echo $user->index();
//调用类或文件不存在救会抛出错误
//系统异常:文件名不合法或不存在
// \app\admin\autoload.php
<?php
spl_autoload_register(function($class){
$file= str_replace('\\',DIRECTORY_SEPARATOR,$class). '.php';
if(!is_file($file) || !file_exists($file)){
try{
throw new \Exception('文件名不合法或不存在');
}catch (\Exception $e){
echo '系统异常:'.$e->getMessage();
}
exit;
}else{
require_once $file;
}
});
// \app\admin\controller\User.php
<?php
//命名空间也哦跟目录结构匹配
namespace app\admin\controller;
//require_once '../model/User.php';
use app\admin\model\User as uM;
class User
{
public function index()
{
echo uM::get_list();
}
}
// \app\admin\model\User.php
<?php
namespace app\admin\model;
class User
{
public static function get_list()
{
return 'model目录User get_list';
}
}
//单图
//<form action="" method="post" enctype="multipart/form-data">
//<input type="file" id="file" name="pic" />
$des ='storage/';
if(!file_exists($des)){
mkdir($des,0770,true);//添加目录设置权限
chmod($des,0700);//修改目录权限
}
$img_up =move_uploaded_file($_FILES['pic']['tmp_name'],$des.$_FILES['pic']['name']);
if($img_up){
$img=$des.$_FILES['pic']['name'];
}else{
$img=null;
}
//多图
//<input type="file" id="file" name="pic[]" multiple />
if(!empty($_FILES['pic'])){
$des ='storage/';
if(!file_exists($des)){
mkdir($des,0770,true);//添加目录设置权限
chmod($des,0700);//修改目录权限
}
$imgs =$_FILES['pic'];
$pic =[];
foreach ($imgs['name'] as $k=>$im) {
$img_up =move_uploaded_file($imgs['tmp_name'][$k],$des.$im);
$pic[] =$des.$im;
}
$img= implode(';',$pic);
}
function uploads($file,$des='./storge/',$flag=true){
if(!empty($file)){
if($file['error'] == 0){
switch($file['error']) {
case 1:
return '文件大小超过了php.ini中允许的最大值,最大值是:'.ini_get('upload_max_filesize');
case 2:
return '文件大小超过了表单允许的最大值';
case 3:
return '只有部分文件上传';
case 4:
return '没有文件上传';
case 6:
return '找不到临时文件';
case 7:
return '文件写入失败';
default:
return '未知错误';
}
}else{
if($flag){
$arr = explode('.',$file['name']);
$houzhui = ['jpg','gif','png'];
if(!in_array($arr[1],$houzhui)){
$res = '图片类型不对';
}
}
if(!file_exists($des)){
mkdir($des,0770,true);//添加目录设置权限
chmod($des,0700);//修改目录权限
}
$imgs =$_FILES['pic'];
$pic =[];
foreach ($imgs['name'] as $k=>$im) {
$file_name_new = date('YmdHis',time());
$file_name_new .= rand(100,999);
$img_up =move_uploaded_file($imgs['tmp_name'][$k],$des.$file_name_new);
$pic[] =$des.$im;
}
$img= implode(';',$pic);
}
return $img;
}
return null;
}
<?php
session_start();// 开启会话
//判断session
if(empty($_SESSION['id'])){
echo '<script>window.location.href="login.php";</script>';
exit;
}
try{
$pdo = new PDO('mysql:host=127.0.0.1;dbname=inbox','root','root');
}catch(PDOException $e) {
echo '数据库连接失败' . $e->getMessage();
}
if(empty($_GET['p'])){
$p = 1;
}else{
$p = $_GET['p'];
}
$count = 5;
$sql = 'SELECT * FROM `user` LIMIT ' . ($p-1)*$count . ',' . $count;
$pre = $pdo -> prepare($sql);
$exe = $pre -> execute();
$data = $pre -> fetchAll();
$pre = $pdo -> prepare('SELECT count(*) as totoal FROM `user`');
$exe = $pre -> execute();
$totoal = $pre -> fetch()['totoal'];
$page = ceil($totoal/$count);
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>用户列表</title>
<link rel="stylesheet" href="list.css">
</head>
<body>
<div class="add"><a href="add.php">添加</a> <a href="loginout.php">退出</a></div>
<table>
<thead>
<tr>
<th width="50">ID</th>
<th width="100">姓名</th>
<th width="100">年龄</th>
<th width="100">手机号</th>
<th width="200">注册时间</th>
<th width="200">登录时间</th>
<th width="100">状态</th>
<th width="100">修改 | 删除</th>
</tr>
</thead>
<tbody>
<?php
foreach($data as $v){
?>
<tr>
<td><?=$v['id'] ?></td>
<td><?=$v['name'] ?></td>
<td><?=$v['age'].'岁' ?></td>
<td><?=$v['tel'] ?></td>
<td><?=$v['ctime'] ?></td>
<td><?=date('Y-m-d H:i:s',$v['utime']) ?></td>
<td>
<?php
if($v['status'] == 1){
echo '<span style="color:green;">开启</span>';
}else{
echo '<span style="color:red;">关闭</span>';
}
?></td>
<td><a href="edit.php?id=<?=$v['id'] ?>">修改</a> | <a href="del.php?id=<?=$v['id'] ?>">删除</a></td>
</tr>
<!-- 这里要循环的html标签 -->
<?php
}
?>
</tbody>
</table>
<ul class="pagination">
<?php
// 当前页面 等于1的话,就相当于没有上一页
$html = '';
if($p == 1){
$html .= '<li class="disabled">';
$html .= ' <a>上一页</a>';
$html .= '</li>';
}else{
$html .= '<li>';
$html .= ' <a href="lista.php?p='.($p-1).'">上一页</a>';
$html .= '</li>';
}
// 总页10 是一个变量
// 当前页 1,也是个变量
for($i=1;$i<=$page;$i++){
$html .= '<li ';
if($i == $p){
$html .= 'class="active"';
}
$html .= '>';
$html .= ' <a href="lista.php?p='.$i.'">'.$i.'</a>';
$html .= '</li>';
}
if($p == $page){
$html .= '<li class="disabled">';
$html .= ' <a>下一页</a>';
$html .= '</li>';
}else{
$html .= '<li>';
$html .= ' <a href="lista.php?p='.($p+1).'">下一页</a>';
$html .= '</li>';
}
echo $html;
?>
<!-- <div id="page" style="float:left;">-->
<!-- <select id="pageNum" onchange="search()"-->
<!-- style="width:50PX;margin-right:1px;" aria-controls="DataTables_Table_0" size="1" name="DataTables_Table_0_length">-->
<!-- <option selected="selected" value="1">1</option>-->
<!-- </select>-->
<!-- </div>-->
<div class="form-group">
<select class="form-control" onchange="window.location=this.value;">
<?php
$pages ='';
for($i=1;$i<=$page;$i++){
$pages .= '<option ';
if($i == $p){
$pages .= 'selected="selected" ';
}
$pages .= 'value="lista.php?p='.$i.'">第'.$i.'页</option>';
}
echo $pages;
?>
</select>
</div>
</ul>
</body>
</html>
<script>
function del(id){
if(confirm("确定要删除该用户吗?")){
this.location = "del.php?id=" + id;
}else{
return false;
}
}
</script>