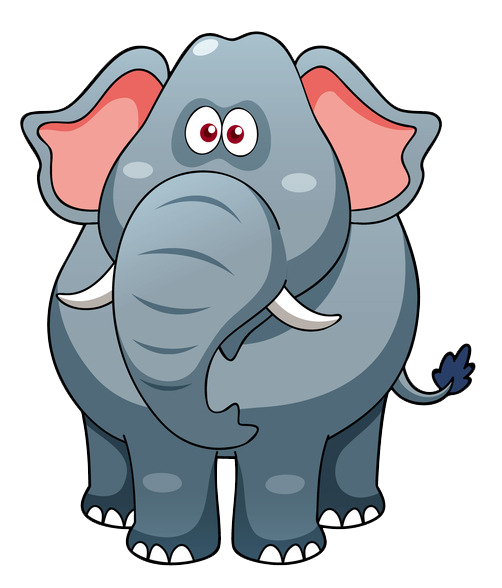
Correction status:qualified
Teacher's comments:
// JS 流程控制分支(if、switch)
// 程序默认执行流程:顺序(默认)
// 单分支
let salary = 36000;
// salary >= 36000 true: 执行
if (salary >= 36000) {
console.log("需要交税");
}
// 双分支: 在单分支基础上加一个"默认分支"
salary = 36000;
if (salary >= 36000) {
console.log("税率:3%+");
}
// * 默认分支
else {
console.log("税率:3%");
}
// 双分支的简化语法,三元运算符
// 语法: (条件) ? true : false
salary = 30000;
salary >= 36000 ? console.log('税率:3%+') : console.log('税率:3%');
// 多分支: 有多个"默认分支"
salary = 960001;
if (36000 < salary <= 144000) {
console.log("税率:10% - 2520");
}
// * 第1个默认分支
else if (144000 < salary <= 300000) {
console.log("税率:20% - 16920");
}
// * 第2个默认分支
else if (300000 < salary <= 420000) {
console.log("税率:25% - 31920");
}
// * 第3个默认分支: 异常分支, 使用 "||" 或 , 满足条件之一就可以了, true
else if (420000 < salary <= 660000) {
console.log("税率:30% - 52920");
}
else if (660000 < salary <= 960000) {
console.log("税率:35% - 85920");
}
// * 默认分支(可选)
else {
console.log("税率:45% - 181920");
}
// switch 进行优化
// 多分支 switch
salary = 960001;
// * 区间判断, 使用 true
switch (true) {
case salary <= 36000:
console.log("个税:3% - 0");
break;
case 36000 < salary && salary <= 144000:
console.log("税率:10% - 2520");
break;
case 144000 < salary && salary <= 300000:
console.log("税率:20% - 16920");
break;
case 300000 < salary && salary <= 420000:
console.log("税率:25% - 31920");
break;
case 420000 < salary && salary <= 660000:
console.log("税率:30% - 52920");
break;
case 660000 < salary && salary <= 960000:
console.log("税率:35% - 85920");
break;
default:
console.log("税率:45% - 181920");
}
// 单值判断: 变量
let lang = "html";
lang = "css";
lang = "javascript";
lang = "js";
lang = "CSS";
lang = "JavaScript";
lang = "HTML";
console.log(lang.toLowerCase());
switch (lang.toLowerCase()) {
// 将传入的进行判断的变量值,进行统一化
// 将传入的字符串, 全部小写, 或者 大写
case "html":
console.log("超文本标记语言");
break;
case "css":
console.log("层叠样式表");
break;
case "javascript":
case "js":
console.log("通用前端脚本编程语言");
break;
default:
console.log("不能识别");
}
// 流程控制: 循环
/**
* * 循环三条件
* * 1. 初始条件: 数组索引的引用 ( i = 0 )
* * 2. 循环条件: 为真才执行循环体 ( i < arr.length )
* * 3. 更新条件: 必须要有,否则进入死循环 ( i++ )
*/
const numroom = ["101", "102", "105", 106, 107];
// !while 循环
i = 0;
while (i < numroom.length) {
console.log(numroom[i]);
// * 更新条件
i++;
}
// * do {} while(), 出口型判断,无论条件是否成立, 必须执行一次代码块
i = 0;
do {
console.log(numroom[i]);
// * 更新条件
i++;
} while (i > numroom.length);
// ! for () 是 while 的简化
// * 语法: for (初始条件; 循环条件; 更新条件) {...}
for (let i = 0; i < numroom.length; i++) {
console.log(numroom[i]);
}
// 优化, 将数组长度,提前计算出来缓存到一个变量中
for (let i = 0, length = numroom.length; i < length; i++) {
console.log(numroom[i]);
}
// ! for - of : 快速迭代处理集合数据
// * 数组内部有一个迭代器的方法, 可以用for-of
// * for-of优点: 用户不必再去关心索引, 而将注意力集中到数组成员上
// v 输出数组中的 "键值" 对组成的数组
for (let item of numroom.entries()) {
console.log(item);
}
// v 输出数组中的 "键" 对组成的数组
for (let item of numroom.keys()) {
console.log(item);
}
// v 输出数组中的 "值" 对组成的数组
for (let item of numroom.values()) {
console.log(item);
}
// ! 默认调用values(),输出值
for (let item of numroom) {
console.log(item);
}
// ! for - in: 遍历对象
const obj = { '101': "sun", '102': "xu", '103': "du", noi: function () {} };
// 遍历对象
for (let key in obj) {
console.log(obj[key]);
}
// 数组也是对象
for (let i in numroom) {
console.log(numroom[i]);
}
// 数组也能用for-in,但不要这么用, for - of, forEach, map...
默认函数
,默认参数一般放最后
f = (a, b = 0) => a + b;
console.log(f(1));
console.log(f(1, 2));
...
rest
// 将多余的参数,全部接收? ...rest
f = (...arr) => arr;
// ...: rest语法,剩余参数,归并参数,将所以参数全部压入到一个数组中
console.log(f(1, 2, 3, 4, 5));
// ...集合数据,可以将它"展开"成一个个独立的元素,用在调用的时候
console.log(...[1, 2, 3, 4]);
// * 数组
f = () => [1, 2, 3];
console.log(f());
// * 对象: 模块
f = () => ({ a: 1, b: 2, get: function () {} });
console.log(f());
let name = "help";
let user = {
// 属性简化
// name = name;
name,
/* function getName(){
return user.name;
} */
getName() {
return this.name;
},
};
console.log(user.name());
console.log(user.getName());
// 传统
console.log("hello world");
// 模板字面量中,可以使用"插值"(变量,表达式),可以解析变量
let name = "help";
console.log("Hello " + name);
// 变量/表达式等插值,使用 ${...}插入到模板字面量中
console.log(`Hello ${name}`);
console.log(`10 + 20 = ${10 + 20}`);
console.log(`${10 < 20 ? `大于` : `小于`}`);
// y 声明模板函数
function total(strings, num, price) {
console.log(strings);
console.log(num, price);
}
let num = 10;
let price = 20;
// y 调用模板函数
total`数量: ${10}单价:${500}`;
// ! 模板函数的优化, 以后只用这一种, 上面也要能看懂
// * 使用 ...rest 将插值进行合并
function sum(strings, ...args) {
console.log(strings);
console.log(args);
console.log(`[${args.join()}] 之和是: ${args.reduce((a, c) => a + c)}`);
}
// 调用
sum`计算多个数和: ${1}${2}${3}${4}`;