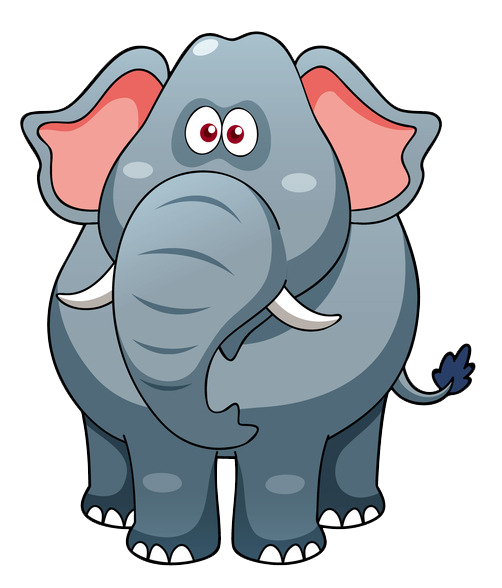
Correction status:qualified
Teacher's comments:
定义:有名字的函数;命名规范为:动词+名词
function getName(userName) {
return "Hello " + userName;
}
console.log(getName("Harvey"));
定义:没有名字的函数;分为二类:立即执行函数和变量函数
立即执行函数(IIFE): 声明 + 执行 2合1,创建临时作用域,不会给全局环境带来任何的污染;
(function (userName) {
console.log("Hello " + userName);
})("Herman");
将函数保存在一个变量中,”函数声明”变成了”变量声明”, 只不过变量的值相当于是一个函数声明;
// 函数声明
const getUserName = function (userName) {
return "Hello " + userName;
};
// 函数调用
console.log(getUserName("Harvey"));
箭头函数就是用来简化匿名函数的声明。
f1 = (a, b) => {
return a + b;
};
console.log(f1(20, 40));
只有一个参数的时候, 参数的括号可以不要了
f1 = username => {
return "Hello " + username;
};
console.log(f1("Harvey"));
没有参数时, 括号一定要写上;如果函数只有一条语句,可以不写大括号,同时函数语句默认就是return ,所以 return 也可以不写。
f1 = () => "今天天气很好";
console.log(f1());
原始类型包含数值型、字符型、布尔型、undefined和null型,此四种类型为基础类型,不可再分,是构成其它复合类型的基本单元。
console.log(123, typeof 123);
console.log("php", typeof "php");
console.log(true, typeof true);
console.log(undefined, typeof undefined);
console.log(null, typeof null);
引用类型都是对象,类似其它语言中的”复用类型”,默认返回 object。引用类型的数据,由一个或多个相同或不同类型的”原始类型”的值构造,是一个典型的多值类型。
// 声明
const arr = ["手机", 2, 5000];
// 输出
console.log(arr);
// 数组的索引是从0开始递增的正整数, 0, 1, 2
console.log(arr[0]);
console.log(arr[1]);
console.log(arr[2]);
console.log(arr[3]);
// 判断数组类型的正确方法
console.log(Array.isArray(arr));
对象的本质就是变量与函数的封装, 变量就是属性, 函数就是方法。
obj = {
name: "手机",
num: 3,
price: 7000,
total: function () {
let str = `${this.name} 总计 ${this.num * this.price} 元`;
return str;
},
};
console.log(obj.total());
函数是一种数据类型,是函数,也是对象。
function f1(callback) {
// 参数 callback 是一个函数
console.log(callback());
}
f1(function () {
return "Hello Herman";
});
function f2() {
let a = 1;
return function () {
return a++;
};
}
// f2()返回值是一个函数
console.log(f2());
const f = f2();
console.log(f);
console.log(f());
console.log(f());
console.log(f());
console.log(f());
console.log(f());
console.log(f());
console.log(f());
console.log(f());