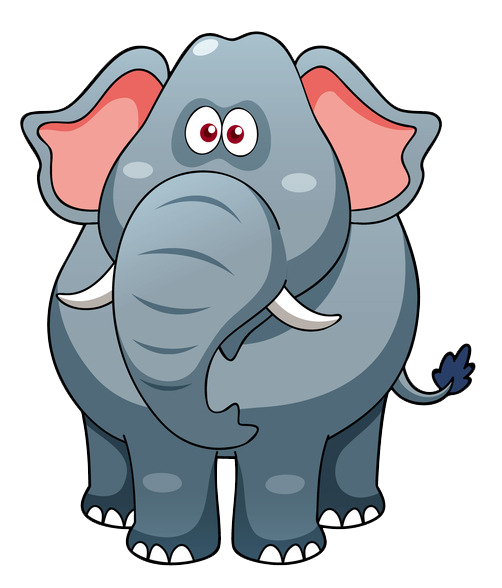
Correction status:qualified
Teacher's comments:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<form action="" method="post" id=login>
<label for="email" class="title">用户登录</label>
<label for="email">邮箱</label>
<input type="text" onblur="check(this)" name="email" class="email" placeholder="请输入邮箱">
<label for="password">密码</label>
<input type="password" onblur="check(this)" name="password" class="password" placeholder="请输入8位数得密码">
<button>提交</button>
</form>
<script>
function check(ele){
event.preventDefault();
event.stopPropagation();
let act = document.activeElement.name;
if (act==="email")
{
return false;
}
if (act=="password")
{
return false;
}
let parent=ele.form;
console.log(ele.value);
if (parent.email.value.length==0)
{
alert("邮箱不能为空");
parent.email.focus();
return false;
}
else if(parent.password.value.length==0)
{
alert("密码不能为空");
parent.password.focus();
return false;
}
else{
alert("验证通过");
return true;
}
}
</script>
</body>
</html>
str.length
str.charAt(0)
str.indexof("中")
str.concat("a","bcs")
str.replace("中文网",".cn")
str.slice(0,3)
str.substr(0,5)
str.tolowercase()
str.toUppercase()
let a=[1,2,3,4,5];
arr=Array.of(...a);
console.log(arr);
const likeArr = {
0: "red",
1: "blue",
2: "green",
length: 3,
};
console.log(likeArr);
console.log(Array.from(likeArr));
arr.push(10)
arr.pop(20)
arr.unshift(10)
arr.shift()
let arr = [1, 2, 3, 4, 5];
let res = arr.forEach(function (item, index, arr) {
// 三个参数中, 只有第一个 item是必须的
console.log(item, index);
// dom
document.body.append(item);
});
res = arr.map(item => item * 2);
console.log(res);
every断言函数,判断数组中每个值是否符合条件,全部符合返回true,否则返回falseconsole.log(arr.every(item => item >= 0));
some: 数组成员中只要有一个满足条件,就返回 true, 否则 false,console.log(arr.some(item => item >= 10));
filter: 返回数组中的满足条件的元素组成的新数组console.log(arr.find(item => item >= 3));
reduce: 归并
res = arr.reduce(function (acc, cur, index, arr) {
console.log("acc=", acc, "cur=", cur, "index=", index, "arr=", arr);
// 最终结果用 acc 返回, acc累加器
return acc + cur;
}, 5);
sort排序升序console.log(arr.sort((a, b) => a - b));
,降序console.log(arr.sort((a, b) => b - a));
join将数组转成字符串
arr = ["red", "green", "blue"];
console.log(arr.join());
console.log(arr.join("-"));
arr = [1, 2, 3, 4, 5, 6, 7, 8, 9];
console.log(arr.slice(2, 5));
console.log(arr.slice(2));
console.log(arr.slice(-6, -3));
// delete
console.log(arr);
console.log(arr.splice(1, 2));
console.log(arr);
// update
console.log(arr.splice(1, 2, "a", "b"));
console.log(arr);
// insert
console.log(arr.splice(1, 0, "red", "green"));
console.log(arr);
let data = ["red", "green", "blue"];
console.log(arr.splice(1, 0, ...data));
console.log(arr);