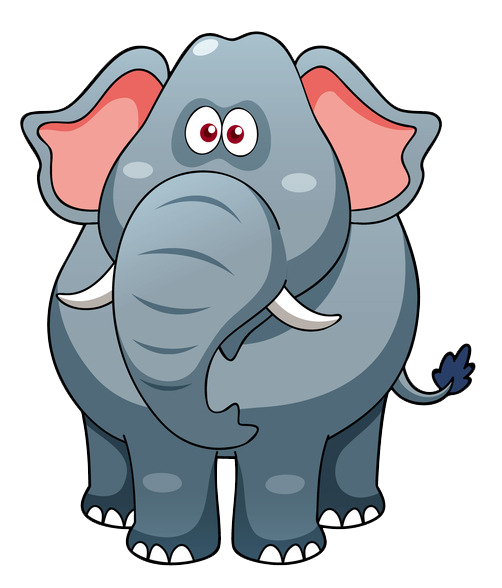
Correction status:qualified
Teacher's comments:
// node编程模式CPS:回调与异步
// 1. 传统风格:
// 定义函数时,return并未执行,程序调用时才执行,并返回结果给调用的地方(调用者)
function add1(a, b) {
return "传统风格:" + (a + b);
}
// 下面调用时,函数执行结果返回调用者
console.log(add1(5, 15));
// 2. CPS风格:
// 函数的参数a,b执行a+b,不直接用return返回,而是(a+b)结果传递给回调函数callback做为其参数使用
// 回调函数callback可以灵活运用,通过(a+b)结果实现特定功能
// 主体逻辑add2中调用回调函数拿到操做结果,而不是直接将其用return返回
function add2(a, b, callback) {
// (a+b)结果传递给回调函数callback做为其参数
callback(a + b);
}
add2(6, 22, (res) => console.log(res));
add2(18, 28, (res) => {
// 1000倍
console.log(res * 1000);
});
// add2是个同步的CPS函数
console.log("开始");
add2(6, 22, (res) => console.log(res));
console.log("结束");
// 显示如下:
// 开始
// 28
// 结束
// 3. 异步CPS函数
function add3(a, b, callback) {
setTimeout(() => {
callback(a + b);
}, 1000);
}
console.log("开始");
add3(6, 22, (res) => console.log(res));
console.log("结束");
// 显示:
// 开始
// 结束
// 28
Node模块风格: CommonJS
模块就是一js文件, 内部成员全部私有,只有导出才可以被访问
// 1. 核心模块无须声明,直接导入并使用
// 例如https模块,创建web服务器
const serverHttps = require("https");
// fs模块,文件服务
const serverFile = require("fs");
// 1. 核心模块无须声明,直接导入并使用
// 例如http模块,创建web服务器
const serverHttps = require("https");
const serverFile = require("fs");
// 2. 文件模块:先声明,再导入
let hero = require("./mode1.js");
console.log(hero.run());
hero = require("./mode2.js");
console.log(hero.run());
mode1.js
// 声名模块文件
// 模块中所有成员都是私有的,只有导出才能使用
// 导出语法exports,它是一个对象,导出多个成员
// 1. 逐个导出,给exports对象添加属性或方法导出
exports.hero = "闪电侠";
exports.superpowers = "神速力";
exports.run = () => {
return `${this.hero}的超能力是${this.superpowers}。`;
};
mode2.js
// 2. 统一导出
// module.exports 是对象,可以赋值
// 把module.exports作为当前模块中所有成员的“命名空间”来使用
module.exports = {
hero: "闪电侠",
superpowers: "神速力",
run() {
return `${this.hero}的超能力是${this.superpowers}。`;
},
};
// http模块
// 导入模块
var http = require("http");
// 创建服务器方法,request为请求对象,response为响应对象
http
.createServer((request, response) => {
// 写响应头,text/plain返回的是文本,text/html返回的是html
// response.writeHead(200, { "Content-Type": "text/plain" });
// 写入浏览器并结束
// response.end("http模块创建的页面。");
// text/html返回的是html
// response.writeHead(200, { "Content-Type": "text/html" });
// response.end("<h2>http模块创建的页面。</h2>");
// application/json返回的是json
response.writeHead(200, { "Content-Type": "application/json" });
response.end(`
{
"hero":"闪电侠",
"superpowers": "神速力"
}
`);
})
// 监听端口,回调函数
.listen(8081, () => {
console.log("服务已运行:http://127.0.0.1:8081/");
});
// 运行后就可访问http://127.0.0.1:8081/
// 引入模块
const fs = require("fs");
// Input.txt是读取的文件,err是错误处理,data是文件内容
// 文件要指定路径
fs.readFile(__dirname + "/input.txt", (err, data) => {
// 判断错误
if (err) return console.error(err);
// data是二进制,转为文本
console.log(data.toString());
});
// 定义字符串
const str = "./php/node.js";
// 引入模块
const path = require("path");
// 解析字符串的绝对路径
console.log(path.resolve(str));
// 显示:c:\www\php\node.js
// 解析目录部分
console.log(path.dirname(str));
// 显示:./php
// 文件名
console.log(path.basename(str));
// 显示:node.js
// 扩展名
console.log(path.extname(str));
// 显示:.js
// 转为对象
console.log(path.parse(str));
// 显示:{ root: '', dir: './php', base: 'node.js', ext: '.js', name: 'node' }
// 对象转为字符串
const obj = {
root: "",
dir: "./php",
base: "node.js",
ext: ".js",
name: "node",
};
console.log(path.format(obj));
// 显示:./php\node.js