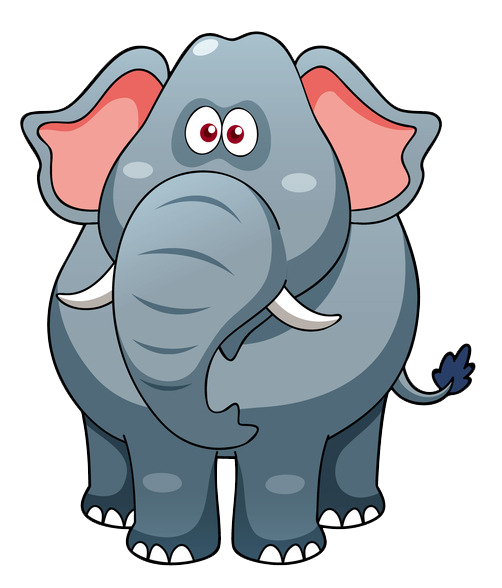
Correction status:qualified
Teacher's comments:
<!-- 作业内容:作业:1. mvc架构模式的运行原理是?三者各自的职责什么?请实例演绎m-v-c分离?2.你是如何理解依赖注入与服务容器的 -->
Model
:模型类,数据库操作View
:视图类,展示到客户端Controller
:控制器,协调模型与视图
Model 代码:
<?php
namespace mvc_demo;
// Model 模型类: 用于数据库操作
class Model
{
public function getData()
{
return (new \PDO('mysql:host=localhost;dbname=yllblroot', 'root','root'))
->query('SELECT * FROM `workplan0921` LIMIT 15')
->fetchAll(\PDO::FETCH_ASSOC);
}
}
View 代码:
<?php
namespace mvc_demo;
// 视图类
class View
{
public function fetch($data)
{
$table = '<table>';
$table .= '<caption>重点工作计划进度明细</caption>';
$table .= '<tr><th>STT</th><th>项目</th><th>计划</th><th>主责人</th><th>完成标准</th><th>开始时间</th><th>结束时间</th><th>完成时间</th><th>备注</th></tr>';
// 将数据循环遍历出来
foreach ($data as $plan) {
$table .= '<tr>';
$table .='<td>' .$plan['stt'] .'</td>';
$table .='<td>' .$plan['project'] .'</td>';
$table .='<td>' .$plan['plan'] .'</td>';
$table .='<td>' .$plan['nguoiphtrach'] .'</td>';
$table .='<td>' .date('Y-m-d', $plan['starttime']) .'</td>';
$table .='<td>' .date('Y-m-d', $plan['endtime']) .'</td>';
$table .='<td>' .date('Y-m-d', $plan['timehoanthanh']) .'</td>';
$table .='<td>' .$plan['ghichu'] .'</td>';
$table .= '</tr>';
}
$table .= '</table>';
return $table;
}
}
echo '<style>
table {border-collapse: collapse; border: 1px solid;text-align: center; margin: auto;}
caption {font-size: 1.2rem; margin-bottom: 10px;}
tr:first-of-type { background-color:wheat;}
td,th {border: 1px solid; padding:5px}
</style>';
控制器一(普通注入):
<?php
namespace mvc_demo;
// 控制器2
// 1. 加载模型类
require 'Model.php';
// 2. 加载视图
require 'View.php';
// 3. 创建控制
class Controller1
{
public function index(Model $model, View $view)
{
// 1. 获取数据
$data = $model->getData();
// 2. 渲染模板/视图
return $view->fetch($data);
}
}
// 客户端:
$model = new Model;
$view = new View;
// 实例化控制器类
$controller = new Controller1;
echo $controller->index($model, $view);
<?php
namespace mvc_demo;
// 控制器3
// 1. 加载模型类
require 'Model.php';
// 2. 加载视图
require 'View.php';
// 3. 创建控制
class Controller2
{
// 依赖对象属性: 对象参数
private $model;
private $view;
public function __construct(Model $model, View $view)
{
$this->model = $model;
$this->view = $view;
}
public function index1()
{
// 1. 获取数据
$data = $this->model->getData();
// 2. 渲染模板/视图
return $this->view->fetch($data);
}
}
// 客户端:
$model = new Model;
$view = new View;
// 实例化控制器类
$controller = new Controller2($model, $view);
echo $controller->index1();
控制器三(对象容器):
<?php
namespace mvc_demo;
// 1. 加载模型类
require 'Model.php';
// 2. 加载视图
require 'View.php';
// 3. 服务容器
class Container
{
// 对象容器
protected $instances = [];
// 绑定: 向对象容器中添加一个类实例
public function bind($alias, \Closure $process)
{
$this->instances[$alias] = $process;
}
// 取出: 从容器中取出一个类实例 (new)
public function make($alias, $params = [])
{
return call_user_func_array($this->instances[$alias], []);
}
}
$container = new Container;
// 绑定
$container->bind('model', function () {return new Model;});
$container->bind('view', function () {return new View;});
class Controller3
{
public function index(Container $container)
{
// 1. 获取数据
$data = $container->make('model')->getData();
// 2. 渲染模板/视图
return $container->make('view')->fetch($data);
}
}
// 客户端:
// 实例化控制器类
$controller = new Controller3();
echo $controller->index($container);
Controller1:
[http://help10086.cn/0221/Controller1.php]
Controller2:
[http://help10086.cn/0221/Controller2.php]
Controller3:
[http://help10086.cn/0221/Controller3.php]
依赖注入
:在客户端实例化类,把对象当做参数注入调用方法容器
:对象容器,对象管家