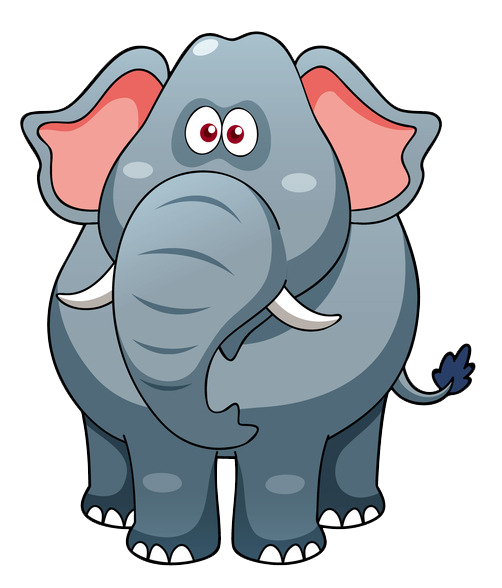
Correction status:qualified
Teacher's comments:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>计算样式</title>
</head>
<body>
<h2 style="color: red;" >第二等级的标题</h2>
<h3>第三等级的标题</h3>
<h4>第四等级的标题</h4>
<style>
.box{
color: yellow;
}
.box2{
background-color: blue;
}
</style>
</body>
<script>
// 获取计算样式有两种
// 第一种是只能计算行内样式
let h2=document.querySelector("h2");
//改变h2背景颜色为蓝色
h2.style.backgroundColor="blue";
//查看h2的背景样式 //控制台打印为blue
console.log(h2.style.backgroundColor);
//由于第一种不常用
//我们来看看第二种获取计算样式,第二种的话能计算外部样式和外联样式
let h3=document.querySelector("h3");
let h3cssobj=window.getComputedStyle(h3); //获取h3的外部样式和外两样式
console.log(h3cssobj.color);//打印rbg(0,0,0)
//我们来为h3标题添加字体颜色
//我们来看classlist属性,classlist属性可以访问,修改以及添加,删除class类的属性
//添加一个或多个类属性
//添加字体颜色为黄色
h3.classList.add("box");
//访问
console.log(h3.classList);
//删除
h3.classList.remove("box");
//toggle() : 当第一个参数存在class属性时,将移除该属性,当不存在class属性,则对元素添加class属性
h3.classList.toggle("box"); //第一个参数不存在,将添加class属性
h3.classList.toggle("box");//第一个参数存在,将移除class属性
</script>
</html>
通过看上面的代码,我们再来看看页面效果
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>js事件</title>
</head>
<body>
<ul class="list">
<li >item1</li>
<li>item2</li>
<li>item3</li>
<li>item4</li>
<li>item5</li>
</ul>
</body>
<script>
// 事件
// let li=document.querySelector("li"); //获取li节点第一个
// li.onclick=function(){
// console.log("事件创建成功");
// }
//事件监听器
//参数一为事件类型,参数二为行为
// li.addEventListener("click",function(){
// event.preventDefault; //取消默认行为
// event.stopPropagation;//停止事件冒泡行为
// console.log(`事件监听器被执行成功` );
// })
//自定义事件
// let click=new Event("click");
// //定时器执行
// setInterval(function(){
// li.dispatchEvent(click);
// },2000)
//事件冒泡
// let ul=document.querySelector(".list");
// li.addEventListener('click',function(){
// console.log(event.currentTarget); //事件的本体
// console.log(event.target); //事件的触发者
// event.preventDefault(); //是个方法,取消默认行为
// event.stopPropagation(); //阻止事件冒泡
// console.log("li");
// });
// ul.onclick=function(){
// console.log('ul');
// }
// document.body.onclick=function(){
// console.log("body");
// }
//事件委托
// 将需要添加到子元素本身上的事件,通过添加到父元素上来进行触发
let ul=document.querySelector(".list");
ul.addEventListener("click",function(){
console.log(event.currentTarget);
console.log(event.target);
console.log("ul");
});
document.querySelectorAll("li")[0].onclick=
function(){
console.log("li1");
}
document.querySelectorAll("li")[1].onclick=
function(){
console.log("li2");
}
document.querySelectorAll("li")[2].onclick=
function(){
console.log("li3");
}
</script>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>js表单事件</title>
</head>
<style>
form{
width: 300px;
margin: 0 auto;
}
fieldset{
/* 设置为网格容器 */
display: grid;
grid-template-columns: 4rem 1fr;
grid-gap: .5rem;
place-items: center;
}
fieldset legend{
text-align: center;
}
fieldset button{
grid-area: auto/ span 2;
}
.box{
box-shadow: 0 0 .2rem mediumturquoise;
border: none;
transition: 1s;
}
</style>
<body>
<form action="" method="POST " id="login">
<fieldset>
<legend>用户登录</legend>
<label for="username">用户名:</label>
<input type="text" name="username" id="username">
<label for="email">邮箱:</label>
<input type="email" name="email" id="email">
<button type="submit">登录</button>
</fieldset>
</form>
<script>
// 1. submit: 提交时触发
// 2. focus: 获取焦点时触发
// 3. blur: 失去焦点时触发
// 4. change: 值发生改变且失去焦点时触发
// 5. input: 值发生改变就会触发,不必等失去焦点,与change不同
let bnt1=document.querySelector("button");
bnt1.addEventListener("click",function(){
event.stopPropagation();//阻止事件冒泡
event.preventDefault();//取消表单默认行为
});
// 每一个表单控件都有对应的表单
let form=bnt1.form;
console.log(bnt1.form);
// 获取用户输入控件
let username=form.username;
//获取邮箱控件
let email=form.email;
//为用户输入框添加获取焦点是触发事件
username.onblur=function(){
if(username.value==''){
console.log("用户名不能为空");
}
}
//获取焦点时
username.onfocus=function(){
console.log(username.classList);
username.classList.add("box");
setTimeout(function(){username.classList.toggle("box")},2500);
}
</script>
</body>
</html>