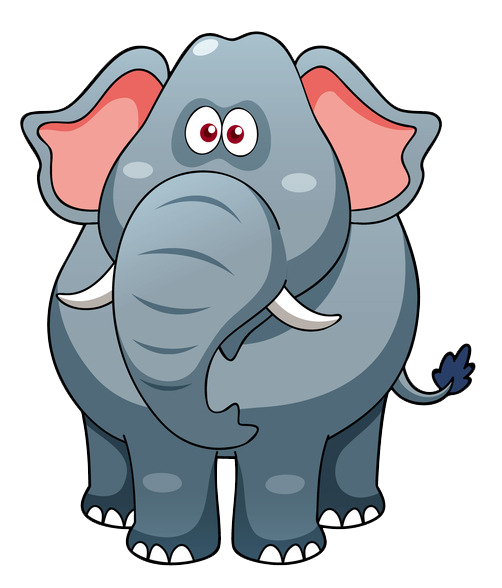
Correction status:qualified
Teacher's comments:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>购物车_vue</title>
<script src="https://unpkg.com/vue@next"></script>
<style>
*{
margin: 0;
padding: 0;
box-sizing: border-box;
}
.box {
width: 35em;
height: 2em;
margin: auto;
}
.list > li {
height: 1.6em;
background-color: #efefef;
display: grid;
grid-template-columns: repeat(5, 6em);
gap: 1em;
place-items: center left;
border-bottom: 1px solid #ccc;
}
.list > li:first-of-type {
background-color: rgb(33, 91, 138);
color: white;
}
.list > li:hover:not(:first-of-type) {
cursor: pointer;
background-color: rgb(85, 125, 212);
}
.list > li input[type="number"] {
width: 3em;
border: none;
outline: none;
text-align: center;
font-size: 1em;
background-color: transparent;
}
.list > li:nth-last-of-type(2) span.total-num,
.list > li:nth-last-of-type(2) span.total-amount {
grid-column: span 2;
place-self: center left;
color: rgb(85, 125, 212);
}
.list > li:last-of-type span{
grid-column: span 2;
place-self: center right;
color: red;
}
</style>
</head>
<body>
<!-- vue挂载点 -->
<div class="app">
<div class="box" @change="updata($event)">
<div class="select-all" @click="selectAll=!selectAll">
<input type="checkbox" class="check-all" :checked="selectAll"/>
<label for="check-all">全选</label>
</div>
<ul class="list" ref="list">
<!-- 表单头 -->
<li>
<span v-for="(item, index) of shopCartList" :key="index"
>{{item}}</span
>
</li>
<!-- 商品list -->
<Item-list name="手机" price="100"></Item-list>
<Item-list name="电脑" price="200"></Item-list>
<Item-list name="相机" price="300"></Item-list>
<Item-list name="冰箱" price="400"></Item-list>
<li>
<span>总计</span>
<span class="total-num">数量: {{numbers}}</span>
<span class="total-amount">总金额: {{totalPay}}</span>
</li>
<li>
<span>折扣金额: {{disCount}}</span>
<span>应付金额: {{disPay}}</span>
</li>
</ul>
</div>
</div>
<!-- 商品list模板 -->
<template id="itemLists">
<li>
<input type="checkbox"/>
<span class="content">{{name}}</span>
<input type="number" v-model="num" min="1"/>
<span class="price">{{price}}</span>
<span class="amount">{{payAmount}}</span>
</li>
</template>
</body>
<script>
// vue实例
const app = Vue.createApp({
data() {
return {
shopCartList: ["选择", "品名", "数量", "单价", "金额"],
numbers:0,
totalPay:0,
disCount:0,
disPay:0,
selectAll:true
};
},
methods:{
updata(el){
if(this.selectAll && ( el===0 || (el.target.className==="check-all") )){
[...document.querySelectorAll(".list>li input[type='checkbox']")].map(item=>item.checked=true);
}else if(el===0 ||el.target.className==="check-all"){
[...document.querySelectorAll(".list>li input[type='checkbox']")].map(item=>item.checked=false);
}
const item_ck = [...document.querySelectorAll(".list>li input[type='checkbox']")];
if(item_ck.every(item=>item.checked)){
this.selectAll=true;
}else{
this.selectAll=false;
}
const item_price = [...document.querySelectorAll(".price")];
const item_num = [...document.querySelectorAll(".list>li input[type='number']")];
// item_ck.forEach(item=>console.log(item.checked));
let temp_num_list = item_num.map((item,index)=>{
if(item_ck[index].checked){
return parseInt(item.value);
}else{
return 0;
}
});
this.numbers = temp_num_list.reduce((res,index)=>res+index);
this.totalPay = temp_num_list.map((item,index)=>item*item_price[index].textContent).reduce((acc,index)=>acc+index);
}
},
watch:{
totalPay(current,origin){
switch(true){
case current>=600:
this.disCount=100;
break;
default:
this.disCount = 0;
}
this.disPay = current-this.disCount;
}
},
mounted(){
this.$nextTick(function(){
this.updata(0);
})
},
});
// 注册组件
app.component("ItemList", {
template: "#itemLists",
props: ["name", "price"],
data(){
return {
num:1,
};
},
computed:{
payAmount(){
return this.num*this.price;
}
}
});
// 挂载实例
app.mount(".app");
</script>
</html>