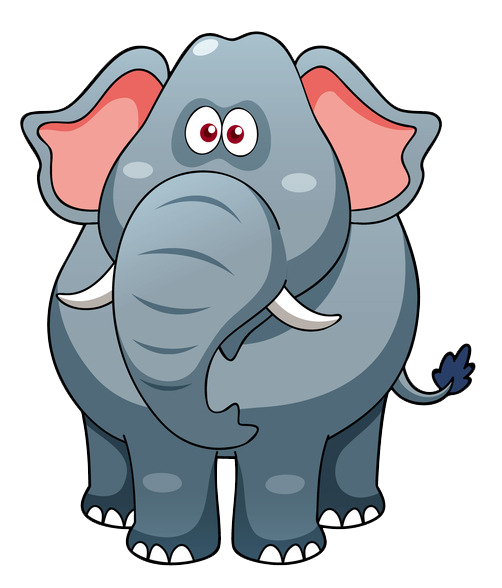
Correction status:qualified
Teacher's comments:
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>挂载点,vue实例,数据注入,响应式</title>
<script src="https://unpkg.com/vue@next"></script>
</head>
<body>
<!-- 1.挂载点:当前vue实例的作用域 -->
<div class="app">
<p>用户名:{{ username }}</p>
</div>
<script>
// 2.vue实例
const app = Vue.createApp(
// vue实例配置对象,要写到一对{}中
{
// vue中的变量/数据全部要写到一个data()
data() {
// 返回所有变量
return {
username: "李老师",
};
},
}
// app.mount(".app");
).mount(".app");
// console.log(app);
// 绑定:将vue实例与挂载点进行绑定
// app.mount(document.querySelector(".app"));
// document.querySelector(".app") => '.app'
// 3.数据注入:本质就是访问器属性
console.log(app.username);
// 完整注入
console.log(app.$data.username);
// 访问器属性
const obj = {
$data: {
email: "admin@qq.com",
},
// 访问器属性
get email() {
return this.$data.email;
},
};
console.log(obj.email);
// 4.响应式,vue实例中的数据,实时响应外部对数据的更新
// 外部的”张老师“ 改变内部的 ”李老师“
app.username = "张老师";
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>v-html和v-text内容填充</title>
<script src="https://unpkg.com/vue@next"></script>
</head>
<body>
<div class="app">
<p>用户名:{{username}}</p>
<p>用户名:<span v-text="username"></span></p>
<p>用户名:<span v-html="username"></span></p>
</div>
<script>
// innerHTML-> vue:v-html
// textContent -> vue:v-text
const app = Vue.createApp({
data() {
return {
username: "李老师",
};
},
}).mount(".app");
app.username = "<i style='color:red'>王老师</i>";
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>行内样式和类样式绑定</title>
<script src="https://unpkg.com/vue@next"></script>
<style>
.active {
color: green;
}
</style>
</head>
<body>
<div class="app">
<!-- 行内样式 -->
<p style="color: red">传统方式样式添加</p>
<p v-bind:style="style">样式绑定</p>
<!-- 语法糖简化:v-bind: =>:-> -->
<p :style="style">语法糖简化方式</p>
<!-- 多个变量改变 -->
<p :style="{color:mycolor,background:mybackground}">语法糖多个变量用对象方式</p>
<!-- class:类样式 -->
<p class="active">class:类样式原生语法演示</p>
<p :class="active">class:类样式vue演示</p>
</div>
<script>
const app = Vue.createApp({
data() {
return {
style: "color:cyan",
mycolor: "blue",
mybackground: "yellow",
active: "active",
};
},
}).mount(".app");
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>列表渲染</title>
<script src="https://unpkg.com/vue@next"></script>
</head>
<body>
<div class="app">
<p>数组渲染</p>
<ul>
<li>{{cities[0]}}</li>
<li>{{cities[1]}}</li>
<li>{{cities[2]}}</li>
</ul>
<ul>
<!-- v-for -> for of -->
<!-- <li v-for="city of cities">{{city}}</li> -->
<!-- :key 必须要添加,必须保证唯一性,diff算法:号称vue精华-->
<li v-for="(city,index) of cities" :key="index">{{index}}=>{{city}}</li>
</ul>
<hr />
<p>对象渲染</p>
<ul>
<li v-for="item of user">{{item}}</li>
<li v-for="(item,key) of user" :key="key">{{key}}=>{{item}}</li>
</ul>
</div>
<script>
const app = Vue.createApp({
data() {
return {
//array
cities: ["兰州", "天水", "白银"],
//obj
user: {
name: "李老师",
age: "29",
},
};
},
}).mount(".app");
</script>
</body>
</html>