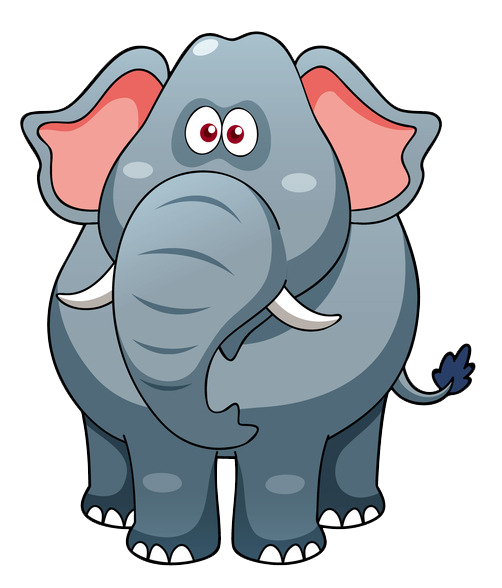
Correction status:qualified
Teacher's comments:
<?php
// printf('<pre>%s</pre>',print_r($_FILES,true));
// 1. 实例演示单文件上传与多文件上传
// 单文件
if(isset($_FILES['myfile'])){
extract($_FILES['myfile']);
if($error===0){
$ext = pathinfo($name)['extension'];
$dest = 'uploads/'. md5($name) . '.' . $ext;
if(move_uploaded_file($tmp_name,$dest)){
echo '<span style="color:green">上传成功</span><br>';
echo "<img src=$dest width='200'>";
}
}
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>单文件上传</title>
</head>
<body>
<form action="" method="post" enctype="multipart/form-data">
<fieldset>
<legend>单文件上传</legend>
<input type="file" name="myfile">
<button>上传</button>
</fieldset>
</form>
</body>
</html>
<?php
//printf('<pre>%s</pre>',print_r($_FILES,true));
// 多文件上传
if(isset($_FILES['myfile'])){
foreach($_FILES['myfile']['error'] as $key=>$error){
if($error===0){
$name = $_FILES['myfile']['name'][$key];
$tmpName = $_FILES['myfile']['tmp_name'][$key];
$desc = 'uploads/'. md5($name).'.'. pathinfo($name)['extension'];
move_uploaded_file($tmpName,$desc);
echo '<span style="color:green">上传成功</span><br>';
echo "<img src=$desc width='200'><br>";
}
}
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>多文件上传</title>
</head>
<body>
<form action="" method="post" enctype="multipart/form-data">
<fieldset>
<legend>多文件上传</legend>
<input type="file" name="myfile[]" multiple>
<button>上传</button>
</fieldset>
</form>
</body>
</html>
<?php
$p = $_GET['p']??1;
// 2. 实例演示简单分页操作,并理解分页所需参数的意思
$db = new PDO('mysql:dbname=phpedu','root','root');
// 数据表总数量:Total
$sql = 'SELECT COUNT(*) AS `total` FROM `staff`';
$stmt = $db->prepare($sql);
$stmt->execute();
$stmt->bindColumn('total', $total);
$stmt->fetch(PDO::FETCH_ASSOC);
// 单页显示数量:num=5
$num = 5;
// 分页数量
$pages = ceil($total/$num);
// 计算偏移量
$offset = ($p-1)*$num;
$sql = "SELECT * FROM `staff` LIMIT $num OFFSET $offset";
$stmt = $db->prepare($sql);
$stmt->execute();
$staffs = $stmt->fetchAll(PDO::FETCH_ASSOC);
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>分页操作</title>
<style>
table {
width: 400px;
border-collapse: collapse;
text-align: center;
}
table th,
table td {
border: 1px solid;
padding: 5px;
}
table thead {
background-color: lightcyan;
}
table caption {
font-size: larger;
margin-bottom: 8px;
}
p>a {
text-decoration: none;
color: #555;
border: 1px solid;
padding: 5px 10px;
margin: 10px 2px;
}
.active {
background-color: seagreen;
color: white;
border: 1px solid seagreen;
}
</style>
</head>
<body>
<table>
<caption>员工信息表</caption>
<thead>
<tr>
<th>ID</th>
<th>姓名</th>
<th>性别</th>
<th>邮箱</th>
</tr>
</thead>
<tbody>
<?php foreach ($staffs as $staff) : extract($staff) ?>
<tr>
<td><?= $id ?></td>
<td><?= $name ?></td>
<td><?= $gender ?></td>
<td><?= $email ?></td>
</tr>
<?php endforeach ?>
</tbody>
</table>
<p>
<?php for ($i = 1; $i <= $pages; $i++) : ?>
<?php
$url = $_SERVER['PHP_SELF'] . '?p=' . $i;
$active = $i == $_GET['p'] ? 'active' : null;
?>
<a href="<?= $url ?>" class="<?= $active ?>"><?= $i ?></a>
<?php endfor ?>
</p>
</body>
</html>