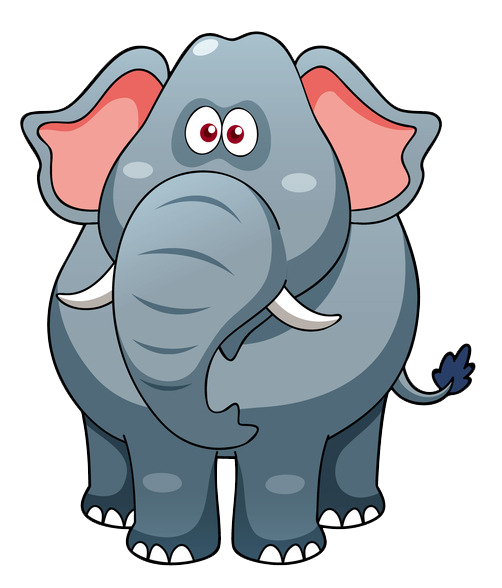
Correction status:qualified
Teacher's comments:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
//内部引入方式
</script>
//xx.js是外部引入的js文件名
<script src="xx.js"></script>
</body>
</html>
...
<script>
// 定义常量PI,定义时必须赋值,常量值不能被更新。
const PI = 3.141592653589793;
// 打印常量值
console.log('PI的值:',PI);
// 定义变量a
let a=0;
console.log('a的初始值:',a);
// 修改变量a的值
a = 10;
</script>
...
...
<script>
...
let a=0;
...
a = 10;
...
// 测试a的变量类型
console.log(typeof(a));
// 测试a+字符串之后的类型
console.log(typeof(a+'10'));
</script>
...
...
<script>
...
// 匿名块作用域内定义变量b并赋值
let b=20;
// 打印b的值
console.log('b的值:',b);
</script>
...
...
<script>
// 定义变量a
let a=0;
...
// 修改变量a的值
a = 10;
...
{
// 匿名块作用域内定义变量b并赋值
let b=20;
// 打印b的值
console.log('b的值:',b);
//打印a和b的值,a是全局作用域,可以打印
console.log('a = ',a,'b = ',b);
}
// 无法得到b,b在块作用域内
//let声明的变量支持作用域
//var声明的变量不支持块作用域
console.log('a = ',a,'b = ',b);
</script>
...
// 定义变量a
let a=0;
...
// 修改变量a的值
a = 10;
...
{
// 匿名块作用域内定义变量b并赋值
let b=20;
...
}
...
// 函数作用域
function sum(a, b){
// 函数内声明变量叫私有变量
return a+b;
}
console.log('sum result is: ', sum(10, 20));
-字母、数字、下划线、$
-不能以数字开头
-全部使用大写
-多个单词间用下划线
大驼峰:类、构造函数,别名,帕斯卡命名法
例:UserName
小驼峰:变量、函数
例:userName
例:
user_name
USER_NAME
就是加了下划线而已
...
<script>
...
function getname(name){
return 'My name is:'+ name;
}
console.log(getname('Wood'));
</script>
...
...
<script>
...
let fullName = function (name){
return 'My name is:'+ name;
}
console.log(fullName('Gavin Wood'));
</script>
...
多次使用时,可以使用立即执行函数。
可以创建出临时的作用域,适合写模块。
...
<script>
...
console.log(
(function(name){
return '你好,'+name;
})('灭绝老倪')
);
</script>
...
用来简化匿名函数
...
<script>
...
arrowName = (intro,name)=>{
return intro+ name;
}
console.log(arrowName('我是','箭头函数'));
</script>
...
如果只有一个参数,小括号可以省略。如果只有一条语句,大括号和return都可以省略。例:
...
<script>
...
arrowName = name=>'我是'+ name;
console.log(arrowName('箭头函数'));
</script>
...
没有参数时,小括号是必须的。
arrowName = ()=>’我是箭头函数’;
也有下面写法:
arrowName = $ =>’我是箭头函数’;
arrowName = _ =>’我是箭头函数’;
箭头函数,内部this是固定的,总是他的上下文绑定,没有自己的this。
箭头函数用在函数参数、返回值、回调函数等处。