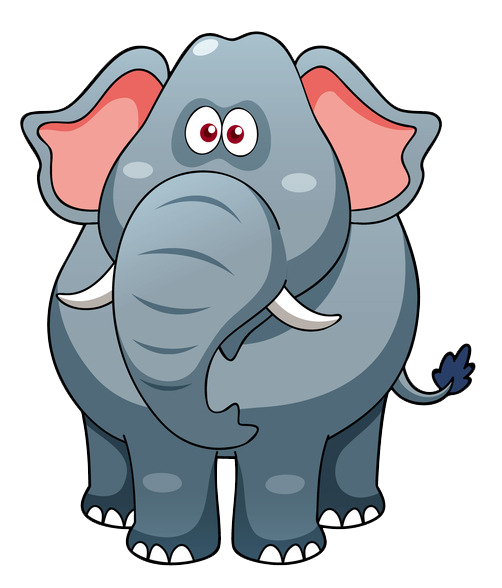
Correction status:qualified
Teacher's comments:
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>数据类型</title>
</head>
<body>
<script>
let a = '我是字符串';
let b = 123;
const arr= ['中文网',123,5000];
// 我是字符串 string
console.log(typeof a);
// 我是数值 number
console.log(typeof b);
// 我是数值。是对象object 类型 布尔类类型 true
console.log(typeof arr);
console.log(arr[2]);
console.log(arr);
console.log(Array.isArray (arr));
// 我是布尔类型
console.log(typeof true);
// 。是返回object 类型 但其实是 null
console.log(typeof null);
console.log(typeof undefined);
// 函数 function函数 是类型 在script 等于对象 , 但是在php不是
console.log(typeof function(){});
// instanceof 判断左右 边数据类型
console.log(function () {} instanceof Object);
console.log(function () {} instanceof Number);
console.log(function () {} instanceof String);
console.log('------------------------');
obj = {
name:'手机',
num: 30,
price:2000,
total :function () {
return obj.num*obj.price;
},
}
console.log(obj);
console.log(obj.total());
console.log('------------------------');
obj1 = {
name:'手机',
num: 30,
price:2000,
total :function () {
// this当前对象obj的引用
return this.num*this.price;
},
}
console.log(obj1.total());
console.log('------------------------');
obj2 = [
{ name:'电暖袋1' ,num:10, price:100 },
{ name:'电暖袋2' ,num:20, price:200 },
{ name:'电暖袋3' ,num:30, price:300 },
]
console.log(obj2);
console.log('------------------------');
console.log(obj2[1]);
console.log('------------------------');
console.log(obj2[1],[2]);
console.log('******************');
function f1(){
}
function f2(f){
console.log(typeof f);
}
// 函数调用函数 将f1传入,成为回调
f2(f1);
// 闭包
function f3(){
// 注意分好
return function(){
return 'hello';
};
}
console.log(f3());
console.log('------------------------');
console.log(f3()());
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>循环、流程</title>
</head>
<body>
<script>
// 单分支
if (true) {
console.log('你好呀');
}
if(false) {
console.log('真的,执行');
}
else {
console.log('假的');
}
// 多分支
// let a =100;
let a =10;
if (a>100){
console.log('我在等死');
}
else if (a<=100 && a>60 ){
console.log('我退休了');
}
else if (a<=60&& a>=18 ){
console.log('我得上班');
}
else{
console.log('我不上班');
}
console.log('------------------');
// 三元操作 条件判断 ? true : fsale
let b=1;
let res = b >= 18 ? '上班' : '不上班';
console.log(res);
console.log(b >= 18 ? '上班' : '不上班');
console.log('*************');
//switch
let c =110;
switch (true) {
case (c<18):
console.log('不上班');
break;
case (c >=18&& c <=60):
console.log('上班');
break;
default:
console.log('等死');
}
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
const colors =['红','绿','黄'];
console.log(colors);
console.log(colors[2]);
console.log(colors.length);
console.log(colors[colors.length - 1]);
console.log('=============');
// 取反
if (undefined) {
console.log(true+',我是真');
}else{
console.log( '我是假');
}
if (!undefined) {
console.log(true+',我是真');
}else{
console.log( '我是假');
}
console.log('=============');
d =0;
let length = colors.length;
console.log(length);
console.log('=============');
// while (d < length) {
// console.log(colors[2]);
// 必须要更新循环条件,否则进入死循环
// d++;
// }
console.log('----------------');
console.log(d);
// while (d > length){
// console.log(colors[d]);
// // d++;
// }
console.log('----------------');
console.log(length);
J = 0;
// 入口判断
while (J < length) {
console.log(colors[J]);
// 必须要更新循环条件,否则进入死循环
J= J+1;
console.log('******************');
console.log(colors);
}
console.log(J);
console.log('----------------');
i = 0;
// 出口判断, 至少要执行一次循环体中的代码,哪必条件为false
do {
console.log(colors[i]);
// 必须要更新循环条件,否则进入死循环
i++;
} while (i > length);
console.log('----------------');
console.log('******************');
for(let g = 0; i < length; i++){
console.log(colors[i]);
}
console.log('----------------');
for(let h of colors){
console.log('我是值valus: ' + h);
}
// 完整语法: 只看值
// for (let item of colors.values()) {
// console.log('values: ' + item);
// }
// for (let item of colors.keys()) {
// console.log('values: ' + item);
// }
console.log('==============');
for(let y of colors.keys()){
console.log('我是键key: ' + y);
}
console.log('==============');
for (let z of colors.entries()) {
console.log('我是键key和值values key-valus,中间用都和隔开: ' + z);
}
console.log('==============');
const obj = {
a: 1,
b: 2,
f: function () {},
};
// 对象要用for -in 遍历
for (let key in obj){
console.log(obj[key]);
}
console.log('----------------');
for(let h in colors){
console.log('我是键key: ' + h);
console.log('我是值valus:' + colors[h]);
}
</script>
</body>
</html>